This content originally appeared on Envato Tuts+ Tutorials and was authored by Kingsley Ubah
React is a JavaScript library built by Facebook for composing user interfaces in a single-page application. In this tutorial, we'll discuss how to properly set up routing and also how to handle nested routing in a React web application using React Router.
A Closer Look at Route Nesting
In order to clearly explain what nested routes are and how they are implemented in React, I created a simple website.
Before proceeding, please view the app demo below. Expand the right window and pay attention to the URLs as you navigate through the site.
Basically, the idea behind this website is that on the front page you have a navigation menu with links to three pages: the Home page, Dashboard page and Products page respectively.
The first two pages, Home and Dashboard, both have simple routes which look like this: /home and /dashboard. However, in certain situations, we might be required to use nested routes to structure our pages.
Back in our case, the Products page will have multiple nested routes to display different pages.
On the Products homepage, we have a route for products search, a route to display the list of products and another route to add a product. The routes for each of these pages will be nested upon /products, as in /products/add, products/all and products/search.
Now that we have understood what nested routes are and have an idea of our project, let's go through the process of setting them up in a React application.
Installations
We'll use the create-react-app
code generator to generate the skeleton of our React project.
You’ll need to have Node >= 14.0.0 and npm >= 5.6 on your machine. To create a project, run the following on your terminal:
npx create-react-app demo-app cd demo-app
This will create your React application inside of the demo-app
folder.
To implement routing in our app, we'll also install the popular react-router-dom
library from npm. React Router is a popular library for routing in React. The library enables the navigation among various components in a React Application by mapping different parts of the application UI to unique URL paths.
To install the react-router package, run the following:
npm i react-router-dom
With the package installed, let's now go over the details for our website.
Project Structure
The overall structure of our website will look like this:
demo-app ├── src │ ├── components │ ├── products │ ├── AddProduct.js │ ├── Products.js │ ├── ProductsList.js │ ├── ProductsSearch.js │ └── SingleProduct.js │ ├── Home.js // path: /home │ └── Dashboard.js // path: /dashboard │ ├── App.css │ ├── App.js │ ├── ProductsData.js │ ├── components ├── public ├── package.json └── README.md
Using this diagram as a reference, create a components
folder inside the folder src
, then inside components
, create the Home.js, Dashboard.js files and the products folder.
Then inside the folder products, create the Products.js, AddProducts.js, ProductsList.js, SingleProduct.js and ProductSearch.js files for the nested pages.
Implementing Routes Within the App
Before we begin creating React components to render the different pages, let's first take a look at the final version of our root component, App.js.
We'll begin by making the imports:
import { BrowserRouter as Router, Link, Routes, Route } from "react-router-dom"; import "./App.css"; import Home from "./components/Home"; import Products from "./components/products/Products"; import Dashboard from "./components/Dashboard"; import ProductsSearch from "./components/products/ProductsSearch"; import AddProduct from "./components/products/AddProduct"; import SingleProduct from "./components/products/SingleProduct"; import ProductsList from "./components/products/ProductsList";
On the first line of the code, we imported some core components to enable us define the routes for our website. The Router
component will act as wrapper for all of our application routes, markup and URL paths. Routes
is used to group together all routes defined within the app. Each route is defined with the Route
component, which takes a URL path and maps that path to a React component.
Below the react-router
imports, we also imported the respective components to be rendered across these routes.
Now, below the imports, include the following code:
function App() { return ( <Router> <nav> <Link to="/"> Home </Link> <Link to="dashboard"> Login </Link> <Link to="products/search"> Products </Link> </nav> <Routes> <Route path="/" element={<Home />} /> <Route path="dashboard" element={<Dashboard />} /> <Route path="products" element={<Products />}> <Route path="search" element={<ProductsSearch />} /> <Route path="list" element={<ProductsList />} /> <Route path="add" element={<AddProduct />} /> <Route path=":id" element={<SingleProduct />} /> </Route> </Routes> </Router> ); } export default App;
To nest routes, we simply placed all four routes as children of /products, thereby making /products the parent route. This organization will create paths like products/search, product/list, product/add and product/2.
The best part of route nesting is that you can define a base layout inside the Products
component. This layout will then be reused across all pages nested within /products in the routes.
Here's the CSS code which goes inside the App.css file:
@import url(https://fonts.googleapis.com/css?family=Open+Sans); .App { text-align: center; } body { padding: 0%; margin: 0%; font-family: "Open Sans", sans-serif; } nav { margin: 0; width: 100%; height: 80px; background-color: purple; display: flex; justify-content: center; align-items: center; color: white; text-decoration: none; } a { color: white; text-decoration: none; margin: 10px; font-size: 25px; } .search { width: 100%; position: relative; display: flex; } .searchTerm { width: 100%; border: 3px solid purple; border-right: none; padding: 5px; height: 20px; border-radius: 5px 0 0 5px; outline: none; color: #9dbfaf; } .searchTerm:focus { color: purple; } .searchButton { width: 40px; height: 36px; border: 1px solid purple; background: purple; text-align: center; color: #fff; border-radius: 0 5px 5px 0; cursor: pointer; font-size: 20px; } /*Resize the wrap to see the search bar change!*/ .wrap { width: 30%; position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); } .products { width: 100%; height: calc(100vh - 80px); display: flex; flex-direction: column; align-items: center; padding-top: 60px; } .productsNav { width: 70%; height: 80px; border-radius: 12px; display: flex; justify-content: center; } .productsNav a { width: 150px; height: 50px; background-color: purple; border-radius: 5px; display: flex; align-items: center; justify-content: center; } .listOfProducts { width: 100%; height: calc(100vh - 80px); display: flex; justify-content: center; padding-top: 60px; } .productsList { columns: 3 auto; display: inline-block; } .productDisplay { width: 300px; height: 300px; background-color: rgb(233, 233, 233); cursor: pointer; } * { padding-top: 0; margin-top: 0; }
Let's take a closer look at implementing base layouts in the following section.
Implementing Base Layout For Nested Routes
We'll include the following code in the src/components/products/Products.js file:
import React from "react"; import { Link, Outlet } from "react-router-dom"; function Products() { return ( <div className="products"> <div className="productsNav"> <Link to="/products/search"> Search </Link> <Link to="/products/list"> List </Link> <Link to="/products/add"> Add </Link> </div> <Outlet /> </div> ); } export default Products;
We began by importing React
, Link
and Outlet
.
As its name implies, Outlet allows you to "let out" the component related to the active route. For example, whenever we navigate to products/add, React Router will "let out" the component AddProduct
and hide the other three nested routes.
An implication of this setup is that any other markup return from the component (as the nav menu in our case) will be outputted across all other nested routes. So the navbar will also appear in products/list and products/2.
Dynamic Routes
Going back to our route structure in App.js, the last route was defined as follows:
// other routes <Route path=":id" element={<SingleProduct />} />
This will be used to display a single product based on the product's id
property.
Include the following code inside of src/components/products/SingleProduct.js:
import React from "react"; import { useParams } from "react-router-dom"; import { ProductsData } from "../../ProductsData"; function ProductSingle() { const { id } = useParams(); return ( <div className="listOfProducts"> <div className="productDisplay"> <h1>{ProductsData[id - 1].name}</h1>{" "} <p>{ProductsData[id - 1].description}</p>{" "} </div> </div> ); } export default ProductSingle;
useParams
allows us to use the parameters related to the active route. Here we destructure the id
property from the param and using this id
, we'll retrieve a corresponding product data from ProductsData
and pass it into the template.
The final version of our app can be viewed here.
Wrapping It Up
In this tutorial, you saw how to implement nested routing in a React application using React Router.
I hope this tutorial helped you understand nested routes in React better. You should now be able to properly structure the routes for your React Application using the react-router-dom package.
React has become one of the main UI frameworks for working on the web. It’s not without its learning curves, and there are plenty of resources to keep you busy, as well. Source code from this tutorial is available on Codesandbox.
This content originally appeared on Envato Tuts+ Tutorials and was authored by Kingsley Ubah
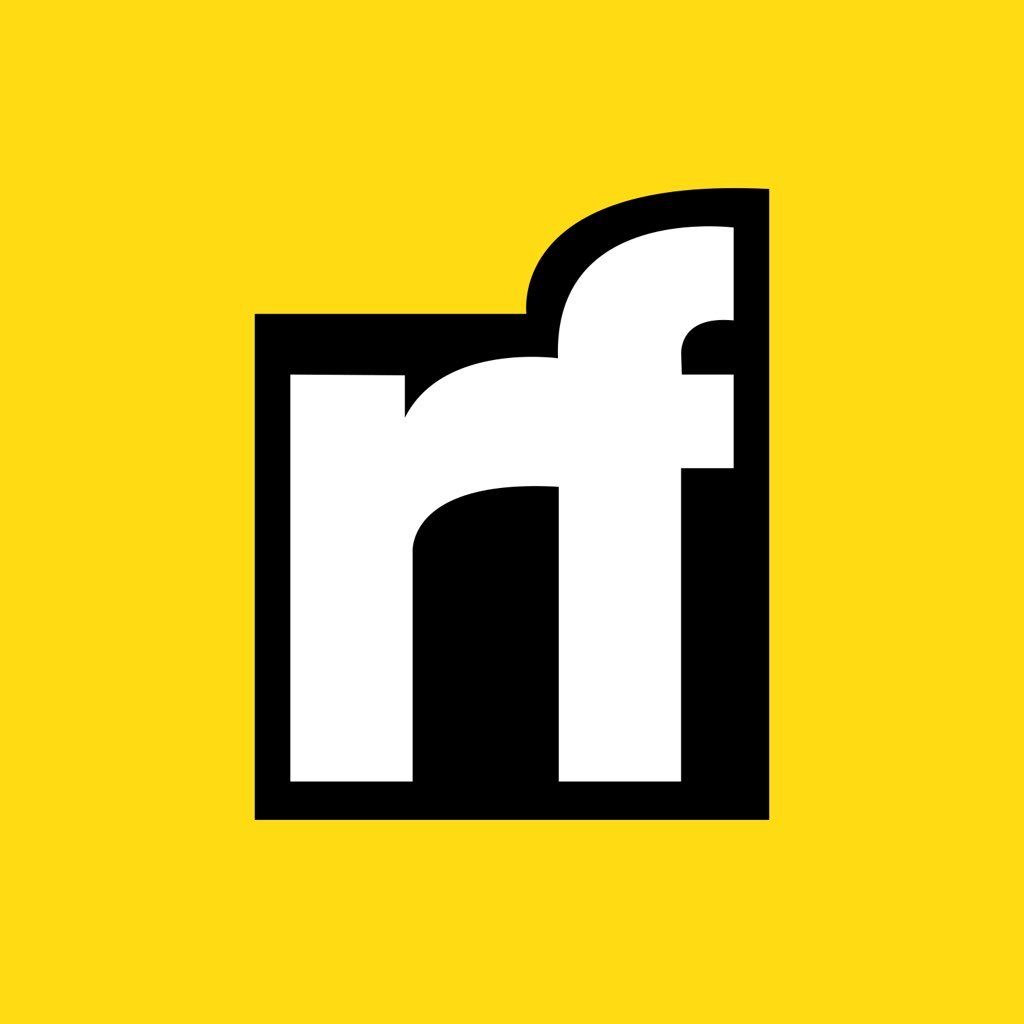
Kingsley Ubah | Sciencx (2016-12-31T08:04:32+00:00) Understanding Nested Routing in React. Retrieved from https://www.scien.cx/2016/12/31/understanding-nested-routing-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.