This content originally appeared on Alligator.io and was authored by Alligator.io
This is the final part of a three-part series about creating an app that leverages the web Push API and cron-schedule. Click here, for the article about creating an installable PWA, and here for the article about the Push API.
Obviously, in this article, we’ll cover the cron-scheduling aspect of the app and we’ll be making use of node-cron to do that.
What are we building
We are building a simple progressive web app (PWA) which will remind me to take my pills every day ??.
Our app will have a web server powered by Express.js. Express will push notifications to the clients which subscribed to the push notifications. Express will also serve the frontend for the app.
What We’ve Built so Far
So far, we created an installable Progressive Web App and an Express.js Server to serve our static files on http://localhost:3000
and push notifications to the frontend.
What We Want to Build
I want to take my ? at 8:30am, 3:30pm and 11:15pm. To do so, we will use a task scheduler.
The Basic of Cron
Cron is (one of || the)
most famous job scheduler out there. It is used in Unix-based operating systems.
Let’s say you have a very susceptible computer. You want to be sure you don’t forget its birthday. If we want to run echo "I love you ?"
every year at a specific date (this will output ‘I love you ?’ in whatever environment you scheduled it in).
Here is what it would look like in cron (try to guess when is my computer’s birthday).
# ┌───────────── minute (0 - 59)
# │ ┌───────────── hour (0 - 23)
# │ │ ┌───────────── day of the month (1 - 31)
# │ │ │ ┌───────────── month (1 - 12)
# │ │ │ │ ┌───────────── day of the week (0 - 6) (Sunday to Saturday;
# │ │ │ │ │ 7 is also Sunday on some systems)
# │ │ │ │ │
# │ │ │ │ │
# 10 8 23 9 * echo "I love you ?"
Every year, the 23rd of September at 8:10am the computer will get a lovely message. Please note that the date is military so if we want to celebrate the birthday at 8pm we would write:
# 10 20 23 9 * echo "I love you ?"
The wildcard sign *
means any
. In this example it means any day of the week. If we wrote:
# 10 20 23 9 1 echo "I love you ?"
It would only wish a happy birthday when the computer’s birthday is on a Monday. The wild card can be used anywhere. To run a command every hour we would do: 0 * * * *
. You can translate that command into “Run command when minute === 0”. Every hour on Monday would be 0 * * * 1
.
For our apps the cron jobs will be:
# Morning ?
30 8 * * *
# Afternoon ?
30 15 * * *
# Night ?
15 23 * * *
Using node-cron
I didn’t choose node-cron
over other Node.js cron-like libraries for any other reason that it works for what I need.
To register a task all we have to do is:
const cron = require('node-cron');
const sendBirthdayMessage = () => { console.log("I love you ?") };
// schedule takes two arguments, cron time and the task to call when we reach that time
cron.schedule('10 20 23 9 1', sendBirthdayMessage())
Let’s incorporate it in our app.
// Each subscription is connected to a client
const allSubscriptions = {}
const registerTasks = (subscription) => {
const endpoint = subscription.endpoint;
// My remote machine is on UTC time and I live in Japan so I made the appropriate calculation
const morningTask = cron.schedule('30 23 * * *', () => {
sendNotification(subscription, JSON.stringify({ timeOfDay: 'morning' }));
});
const afternoonTask = cron.schedule('30 6 * * *', () => {
sendNotification(subscription, JSON.stringify({ timeOfDay: 'afternoon' }));
});
const nightTask = cron.schedule('15 14 * * *', () => {
sendNotification(subscription, JSON.stringify({ timeOfDay: 'evening' }));
});
allSubscriptions[endpoint] = [morningTask, afternoonTask, nightTask];
};
app.post('/subscribe', (req, res) => {
const subscription = req.body;
registerTasks(subscription);
res.send('subscribed!');
});
// Allows our client to unsubscribe
app.post('/unsubscribe', (req, res) => {
const endpoint = req.body.endpoint;
allSubscriptions[endpoint].forEach(task => {
// destroy allows us to delete a task
task.destroy();
});
delete allSubscriptions[endpoint];
});
✨ And that's it! We are finally done with the app, you can find the full code in this Github repository. ✨
This content originally appeared on Alligator.io and was authored by Alligator.io
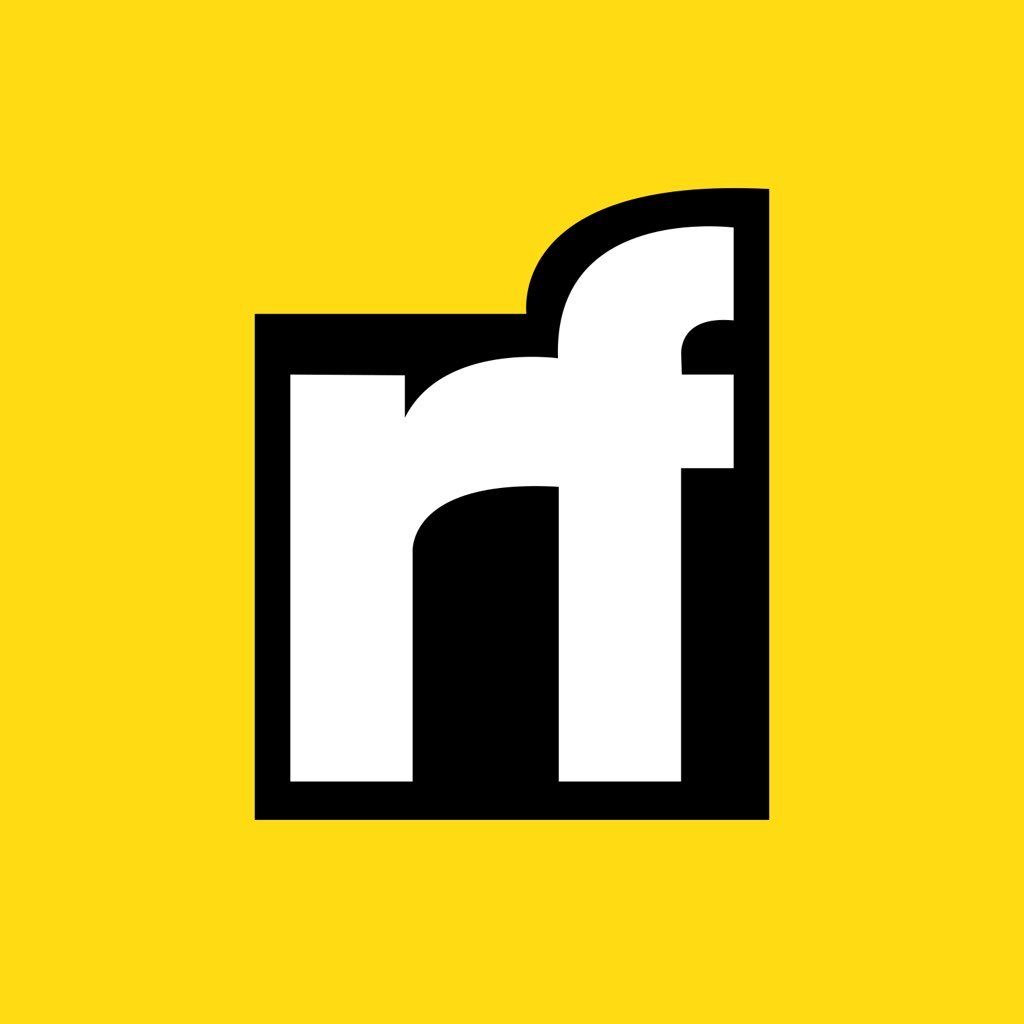
Alligator.io | Sciencx (2020-03-07T00:00:00+00:00) Scheduling Tasks in Node.js with node-cron. Retrieved from https://www.scien.cx/2020/03/07/scheduling-tasks-in-node-js-with-node-cron/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.