This content originally appeared on CodeSource.io and was authored by Deven
In JavaScript to Check if two Arrays Are Equal the most simple approach is using a basic for
loop with a counter. below is a simple example:
function checkArraysAreEqual(arrayA, arrayB) {
if (!Array.isArray(arrayA) || !Array.isArray(arrayB)) {
return false;
} else if (arrayA === arrayB) {
return true;
} else if (arrayA.length !== arrayB.length) {
return false;
} else {
for (let x = 0; x < arrayA.length; ++x) {
if (arrayA[x] !== arrayB[x]) return false;
}
return true;
}
}
const vegetableNamesA = ["potato", "tomato", "onion", "ladyfinger"];
const vegetableNamesB = ["tomato", "onion", "ladyfinger", "potato"];
console.log(
checkArraysAreEqual(vegetableNamesA.sort(), vegetableNamesB.sort())
);
In the code snippet above we sort our arrays before we test them for equality. The above code returns True
in the console.
The post JavaScript Check if Two Arrays Are Equal appeared first on CodeSource.io.
This content originally appeared on CodeSource.io and was authored by Deven
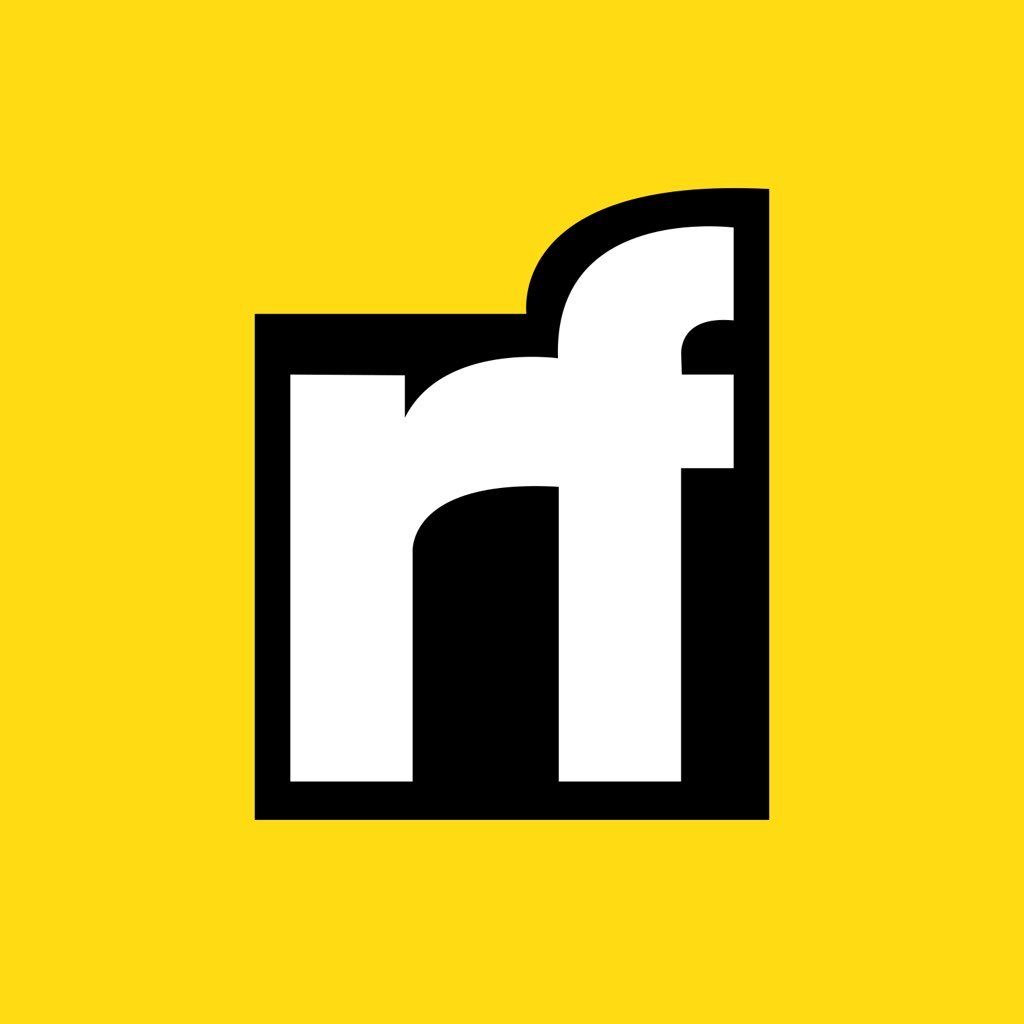
Deven | Sciencx (2021-02-07T15:06:21+00:00) JavaScript Check if Two Arrays Are Equal. Retrieved from https://www.scien.cx/2021/02/07/javascript-check-if-two-arrays-are-equal/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.