This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
The Python standard library provides the socketserver
package. We can use that to create a TCP server.
from socketserver import BaseRequestHandler, TCPServer
class handler(BaseRequestHandler):
def handle(self):
while True:
msg = self.request.recv(1024)
if msg == b'quit\n':
break
self.request.send(b'Message received: ' + msg)
with TCPServer(('', 8000), handler) as server:
server.serve_forever()
Connect to this using Netcat, a handy utility that is very useful to test-drive TCP and UDP servers. It’s installed by default on Linux and macOS, available under the nc
command:
nc localhost 8000
Once it’s connected to the server, you can send any message by typing it. The server will reply with a confirmation of the message received.
Until you say quit
. Then the connection will close (but the server will still run, you can connect again)
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
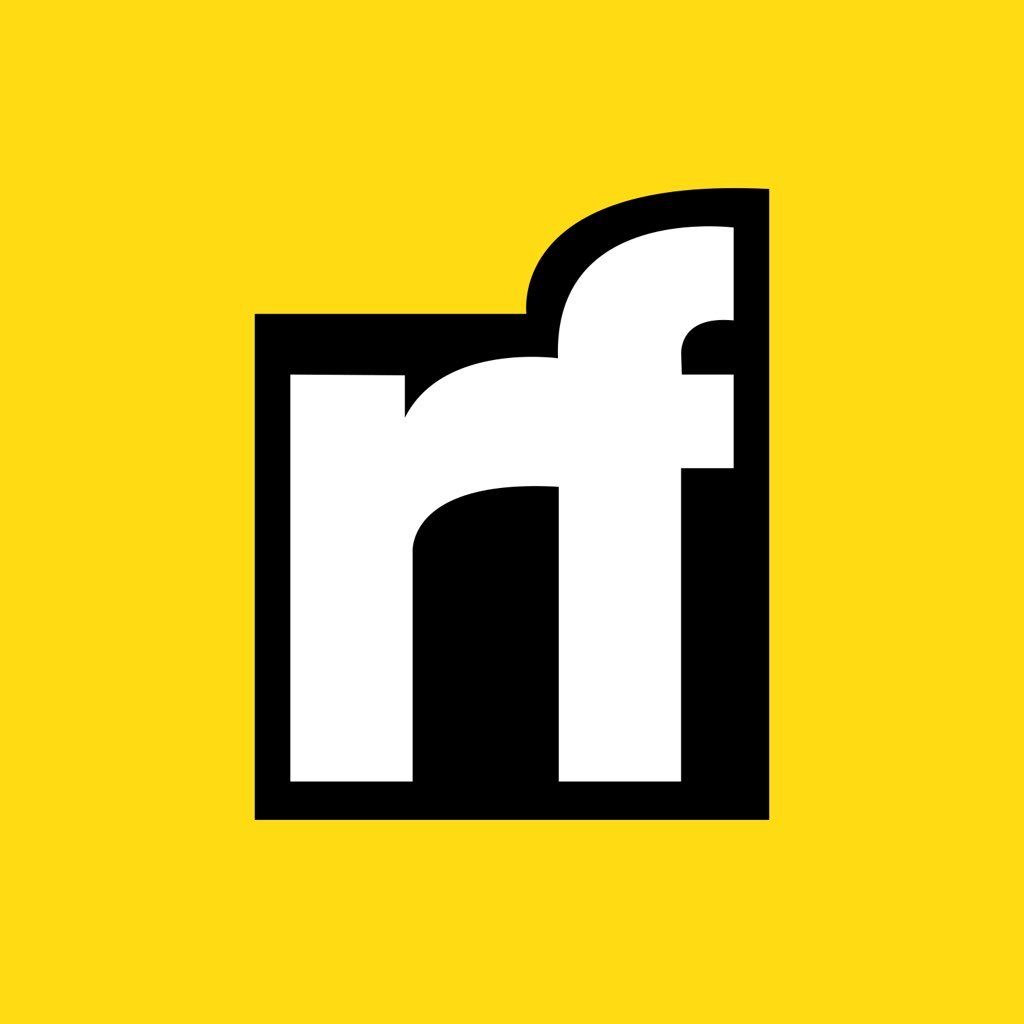
flaviocopes.com | Sciencx (2021-02-11T05:00:00+00:00) Python, create a TCP server. Retrieved from https://www.scien.cx/2021/02/11/python-create-a-tcp-server/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.