This content originally appeared on Level Up Coding - Medium and was authored by Satyam Saluja
Good animations make the interface user-friendly and creative. Adding animations with Flutter is a bliss! In Flutter, there are 2 ways to add animations:
- Implicit animations
- Explicit animations
Implicit animations hide the internal complexity and manages animations for you. On the other hand, explicit animations provide more control and customizations, but has a learning curve.
This article is all about leveraging implicit animations and creating a social share button animation as shown below
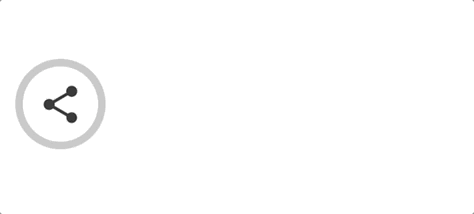
Controlling Implicit animations:
To control your animations, ImplicitlyAnimatedWidget provides you with 3 properties:
- duration - length of the animation
- curve - rate of change of animation within the given duration (list of curves)
- onEnd - callback function which gets called once the animation completes(can be used to trigger different animation or move to different route once the animation completes)
Let’s code’m up!
Consider the following code snippet:
Overwhelming? Don’t worry, let try to visualise the above code using a widget diagram
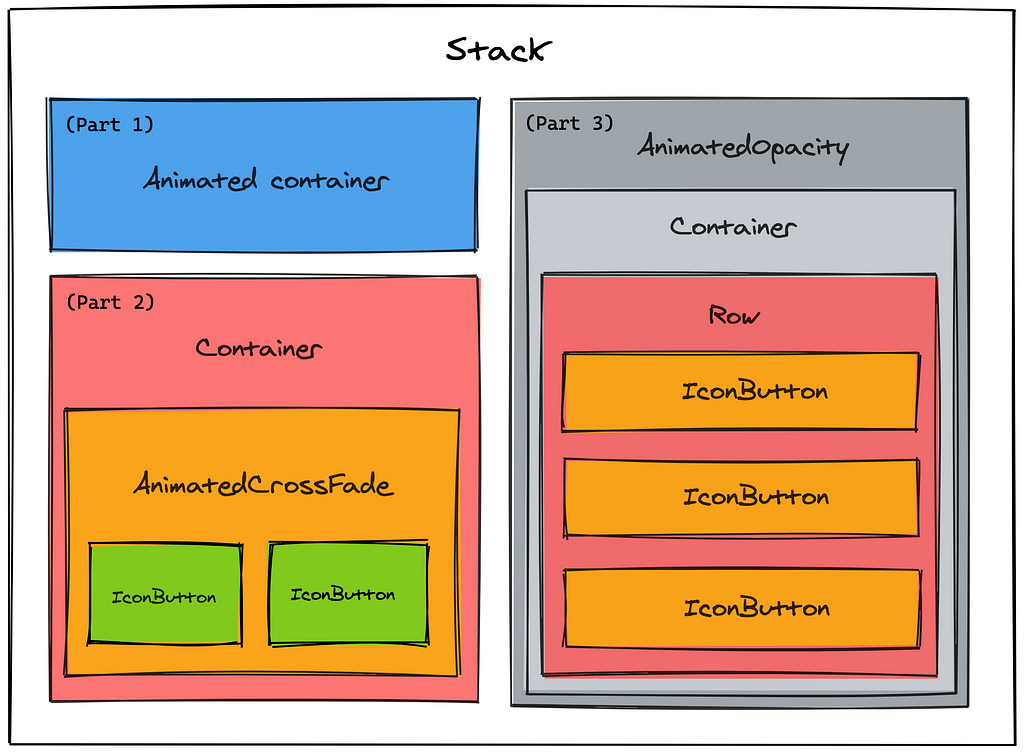
The beauty about Flutter is that everything can be broken down into widgets. Let us divide the whole widget diagram into 3 parts as mentioned and study each widget one by one.
Stack
A widget that positions its children relative to the edges of its box.
Stack acts as parent widget in our example.
Stack is useful if you want to overlap several children in a simple way. We have stacked AnimatedContainer (Part 1), Container (Part 2) and AnimatedOpacity (Part 3) which we’ll be discussing next.
Part 1
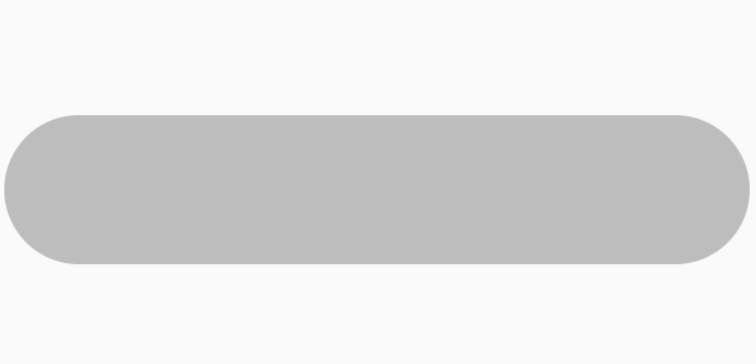
AnimatedContainer
AnimatedContainer as the name suggests is the animated version of Container that gradually changes its values over a period of time.
The AnimatedContainer will automatically animate between the old and new values of properties when they change using the provided curve and duration.
AnimatedContainer(
duration: const Duration(milliseconds: 350),
curve: Curves.fastOutSlowIn,
width: isOpen ? 240 : 48,
height: 48,
decoration: ShapeDecoration(
color: Colors.grey[400],
shape: StadiumBorder(),
),
)
You see, in our example we have a grey box which container Twitter, Facebook and Instagram share options. According to its open or closed state, we change the width to either 240 or 48 and over the period of 350 milliseconds using curve fastOutSlowIn and it get’s animated.
Part 2
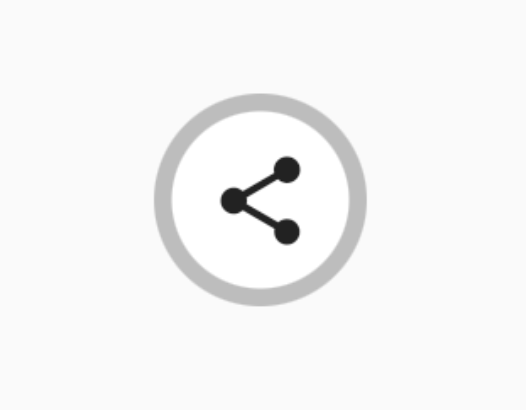
Container
A convenience widget that combines common painting, positioning, and sizing widgets.
We use Container widget to add a white circular shaped container, which wraps AnimatedCrossFade widget that we’ll be discussing next.
Container(
width: 40,
margin: const EdgeInsets.only(left: 4),
decoration: BoxDecoration(
color: Colors.white,
shape: BoxShape.circle,
),
child: AnimatedCrossFade(
...
AnimatedCrossFade
A widget that cross-fades between two given children and animates itself between their sizes.
For open and close state of our social share widget, we need to show either a share icon or across icon button. To achieve the toggle transition, we have used AnimatedCrossFade widget.
AnimatedCrossFade(
duration: const Duration(milliseconds: 450),
firstChild: IconButton(
icon: Icon(Icons.share),
onPressed: () => _toggleShare(),
),
secondChild: IconButton(
icon: Icon(Icons.close),
onPressed: () => _toggleShare(),
),
crossFadeState: !isOpen
? CrossFadeState.showFirst
: CrossFadeState.showSecond,
),
IconButton
Since we need to toggle the open and closed state on click, we have used IconButton widget. On onPressed callback, we toggle the open and closed state by changing the value of isOpen to true or false inside setState
Part 3
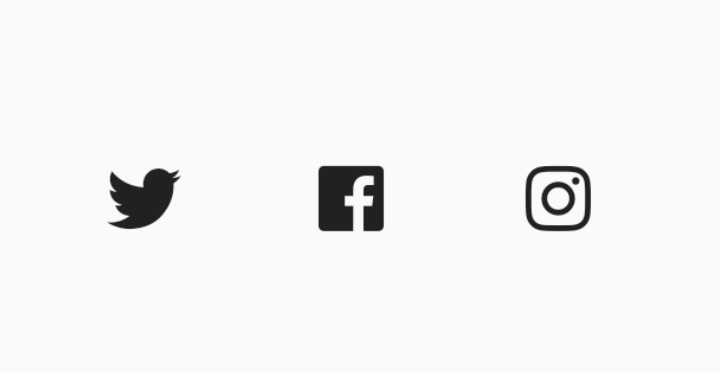
AnimatedOpacity
AnimatedOpacity automatically transitions the child’s opacity over a given duration whenever the given opacity changes.
If you observe closely in our example, we have animated the opacity of our share items(Twitter, Facebook and Instagram)
We’ve achieved this using AnimatedOpacity widget and changing the opacity to 1 or 0 according the the open or closed state.
AnimatedOpacity(
duration: const Duration(milliseconds: 450),
opacity: isOpen ? 1 : 0,
child: Container(
...
Container
To layout 3 share options — Twitter, Facebook and Instagram, we have used another Container widget.
Container(
width: 240,
padding: const EdgeInsets.only(left: 40),
child: Row(
...
Row
A widget that displays its children in a horizontal array.
To align our 3 share options, we have used Row widget and to distribute the space evenly, we have set mainAxisAlignment to MainAxisAlignment.spaceAround
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
IconButton(
icon: Icon(AntDesign.twitter),
onPressed: () {},
),
IconButton(
icon:Icon(AntDesign.facebook_square),
onPressed: () {},
),
IconButton(
icon: Icon(AntDesign.instagram),
onPressed: () {},
),
],
),
IconButton
Each of our individual share option is a IconButton widget. You can handle onPressed callback to trigger the share functionality in your application.
And voila! You have a beautiful animated share button widget in place!
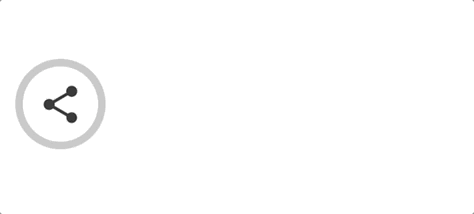
Thank you for taking time and scrolling till the end ?
Follow me for more awesome content. Let’s connect over - Twitter, LinkedIn or Github
References:
- raywenderlich.com | High quality programming tutorials: iOS, Android, Swift, Kotlin, Flutter, Server Side Swift, Unity, and more!
- Flutter - Dart API docs
Animate a social share button using Implicit animations in Flutter was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Satyam Saluja
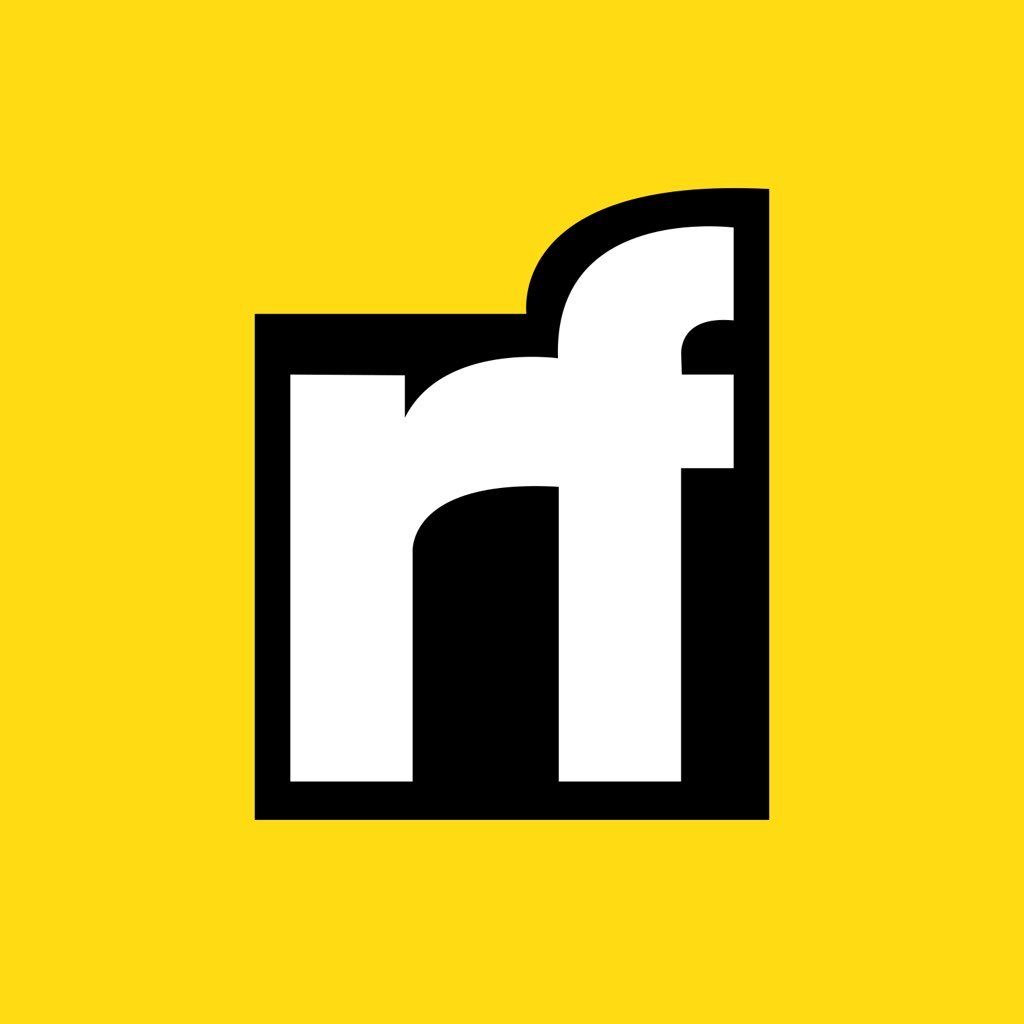
Satyam Saluja | Sciencx (2021-02-15T01:29:34+00:00) Animate a social share button using Implicit animations in Flutter. Retrieved from https://www.scien.cx/2021/02/15/animate-a-social-share-button-using-implicit-animations-in-flutter/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.