This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
Polymorphism generalizes a functionality so it can work on different types. It’s an important concept in object-oriented programming.
We can define the same method on different classes:
class Dog:
def eat():
print('Eating dog food')
class Cat:
def eat():
print('Eating cat food')
Then we can generate objects and we can call the eat()
method regardless of the class the object belongs to, and we’ll get different results:
animal1 = Dog()
animal2 = Cat()
animal1.eat()
animal2.eat()
We built a generalized interface and we now do not need to know that an animal is a Cat or a Dog.
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
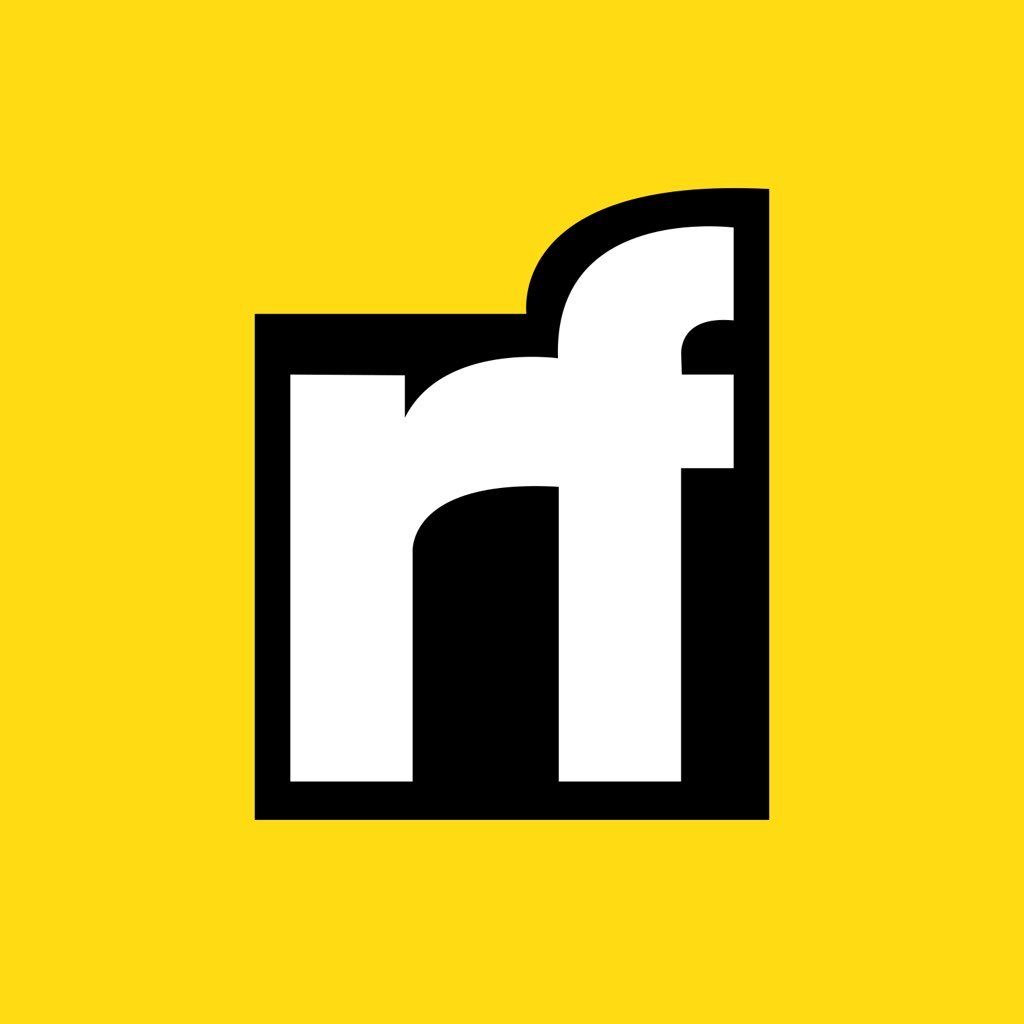
flaviocopes.com | Sciencx (2021-02-20T05:00:00+00:00) Python Polymorphism. Retrieved from https://www.scien.cx/2021/02/20/python-polymorphism/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.