This content originally appeared on DEV Community and was authored by Tulio Calil
The React community has created incredible hooks since this feature was released, I will show some of the 5 that I use and help me a lot.
- Image lazy load
- Outside click hook
- Get browser location
- Read from and Write to clipboard
- Set document title
generated with Summaryze Forem ?
Image lazy load ?
React use lazy load image uses the Intersection Observer API to provide a performant solution to lazy loading images, it a super lightweight so won't add any extra bulk to your app, and its very simple to use:
import React from 'react';
import useLazyLoadImage from 'react-use-lazy-load-image';
function App() {
useLazyLoadImageReact();
return (
<div>Lots of content that means the image is off screen goes here</div>
<img src="IMAGE DATA" data-img-src="https://link-to-my-image.com/image.png" alt="My image" />
)
}
Outside click hook ?
I think that useOnClickOutside is one of the bests, with this hook, you can easily capture outside clicks from an element, very useful for a modal for example.
Here is a demo code:
import * as React from 'react'
import useOnClickOutside from 'use-onclickoutside'
export default function Modal({ close }) {
const ref = React.useRef(null)
useOnClickOutside(ref, close)
return <div ref={ref}>{'Modal content'}</div>
}
Get browser location ?
useLocation help you to get browser location.
import {useLocation} from 'react-use';
const Demo = () => {
const state = useLocation();
return (
<pre>
{JSON.stringify(state, null, 2)}
</pre>
);
};
Read from and Write to clipboard ⌨️
useClippy is a small hook that helps you to easily read from and write to the user's clipboard board:
import useClippy from 'use-clippy';
export default function MyComponent() {
const [clipboard, setClipboard] = useClippy();
return (
<div>
<button
onClick={() => {
alert(`Your clipboard contains: ${clipboard}`);
}}
>
Read my clipboard
</button>
<button
onClick={() => {
setClipboard(`Random number: ${Math.random()}`);
}}
>
Copy something new
</button>
</div>
);
}
Set document title ???
@rehooks/document-title allows you to set the page title simple calling it from a component and passing the title string:
import useDocumentTitle from '@rehooks/document-title';
function MyComponent() {
useDocumentTitle('Page Title');
return <div/>;
}
Conclusion
These are some of the hooks that I use and save me a lot of time.
Do you know some incredible hooks? Comment here!
All the best!
This content originally appeared on DEV Community and was authored by Tulio Calil
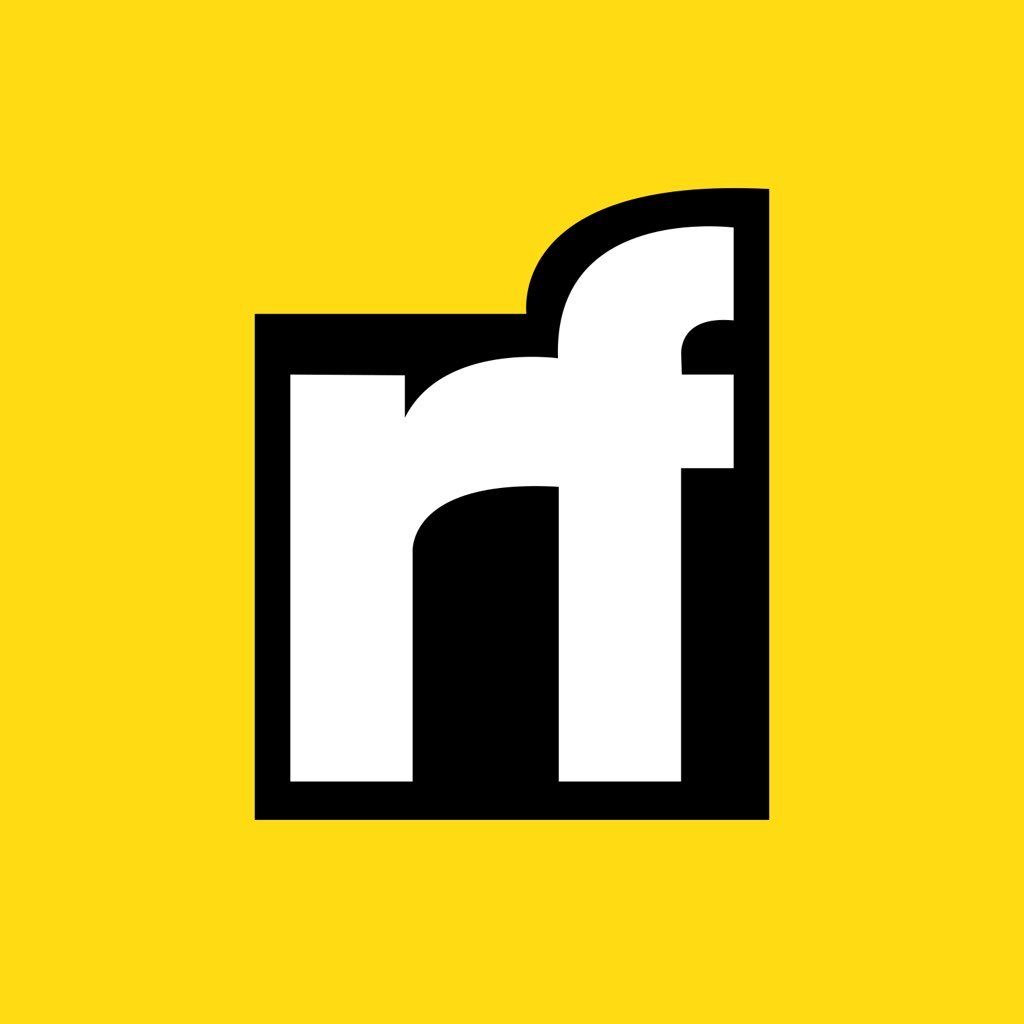
Tulio Calil | Sciencx (2021-03-13T12:16:21+00:00) 5 Awesome React Hooks ⚛️. Retrieved from https://www.scien.cx/2021/03/13/5-awesome-react-hooks-%e2%9a%9b%ef%b8%8f/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.