This content originally appeared on DEV Community and was authored by CodeOzz
Hey ! Welcome here ! At the end of this article you will improve your javascript skills thanks to know the difference between a lot of concept that some dev is always asking !
Spread vs Rest Operator
The operator is the same (...
), but the usage is different.
The spread operator
is use for argument function, it allows us to have an unlimited number of parameter for this function !
// rest parameter is handle as array in the function
const add = (...rest) => {
return rest.reduce((total, current) => total + current)
}
// Nice your function can handle different number of parameters !
add(1, 2, 3)
add(1, 2, 3, 4, 5)
The rest operator
has another utilisation, it permits to extract values from array
or object
, for example :
// We extract the first Item of the array into the variable and the others variable in an array named others
const [ firstItem, ...others ] = [ 1, 2, 3, 4 ]
firstItem // 1
others // [ 2, 3, 4 ]
We can make it with object
const toto = { a: 1, b: 2, c: 3 }
const { a, ...others } = toto
a // 1, we extract the a key from toto object
others // { b: 2, c: 3 }, we extract other key in the object thanks to rest operator
Nullish coalescing (??
) vs OR operator (||
)
At the first impression we can assimilate the fact that Nullish Coalescing (??
) operator is similar to the OR (||
) operator. But it's not the case actually !
The Nullish
will check if the value is null
or undefined
,
and ...
The OR
operator will check if the value is falsy
or not.
It's not really understood ? Let's check this in the code bellow !
const foo = 0 ?? 42
const foo2 = 0 || 42
foo // 0 since nullish check if value is null or undefined
foo2 // 42 since OR operator will check if the value is falsy, in this example it's the case
const toto = null ?? 42
const toto2 = null || 42
toto // 42 since nullish check if value is null or undefined
toto2 // 42 since OR operator will check if the value is falsy, in this example it's the case (null is a falsy value)
Double equals (==
) vs Triple equals (===
)
The double equals
will check value not the type, but if the type is different, it will make a implicit coercion
, in order to convert value into the same type and check the value.
The mechanic of implicit coercion
is not easy to understand but you can check it in details in my previous article https://dev.to/codeozz/implicit-coercion-in-javascript-neh
The triple equals
will check the value AND the type ! And it will not make an implicit coercion like double equal !
In general I advise you to always use triple equals !
A code bellow will illustrate the difference :
Double equals example :
// Not the same type so implicit coercion will be made
'24' == 24
// Convert string into number so
Number('24') == 24
// We got an number so we can check value
24 == 24 // true !
Triple equals example :
// Not the same type and triple equal will not make an implicit coercion !
'24' === 24 // false
var vs let vs const
A common question ask in interview !
I will divide this explication into 3 parts :
I) Scope
var
declaration are globally scoped or function scoped.
if (true) {
var timeVar = 56
}
console.log(timeVar) // 56
It can be dangerous since you can erase an existing variable
var timeVar = 24
if (true) {
// the first variable is erased
var timeVar = 56
console.log(timeVar) // 56
}
console.log(timeVar) // 56
let
& const
declaration are block scoped.
if (true) {
let timeLet = 56
const timeConst = 98
console.log(timeLet) // 56
console.log(timeConst) // 98
}
console.log(timeLet) // Error: timeLet is not defined in this scope since it's only block scope
console.log(timeConst) // Error: timeConst is not defined in this scope since it's only block scope
II) Re-declaration & Update variable
var
can be re-declared and updated
// can be re-declared
var timeVar = 56
var timeVar = 'toto'
// can be updated
timeVar = 'tutu'
let
can't be re-declared and updated
// can't be re-declared
let timeLet = 56
let timeLet = 'toto' // Error: Identifier 'timeLet' has already been declared
// can be updated
timeLet = 'tutu'
const
can't be re-declared and can't be updated
// can't be re-declared
const timeConst = 56
const timeConst = 'toto' // Error: Identifier 'timeConst' has already been declared
// can't be updated
timeConst = 'tutu' // TypeError: Assignment to constant variable.
III) Hoisting
Some people are not aware about Hoisting
in javascript, I will not explain you what is this in this article, but hoisting handle var
, let
and const
is a different way.
// They are all hoisted to the to of their scope.
// But while var variable are initialized with undefined
// let and const variables are not initialized.
myVarVariable // undefined
myLetVariable // Error since not initialized by Hoisting, you cannot use it's declaration
myConstVariable // Error since not initialized by Hoisting, you cannot use it's declaration
myVarVariable = 5
myLetVariable = 'letValue'
myConstVariable = 'constValue'
var myVarVariable
let myLetVariable
const myConstVariable
In general I advise you to always use const
(for constant value) and let
for other purpose.
I hope you like this article, I'm trying to share some knowledge in JS in a different way, in this article it's about difference !
This content originally appeared on DEV Community and was authored by CodeOzz
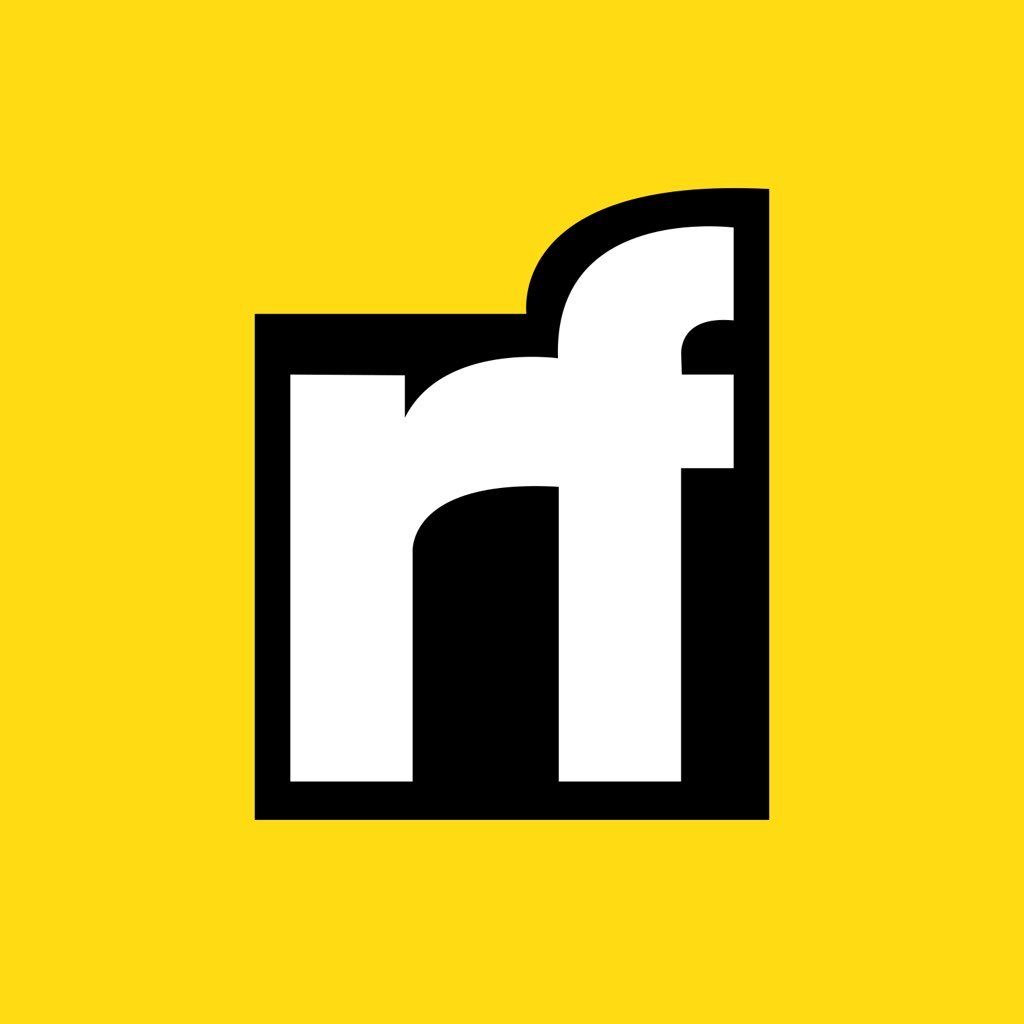
CodeOzz | Sciencx (2021-03-23T10:49:29+00:00) Know the difference between theses JS concept in order to skill up #1. Retrieved from https://www.scien.cx/2021/03/23/know-the-difference-between-theses-js-concept-in-order-to-skill-up-1/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.