This content originally appeared on Bits and Pieces - Medium and was authored by Viduni Wickramarachchi
Using Workbox with Webpack to Generate Service Workers
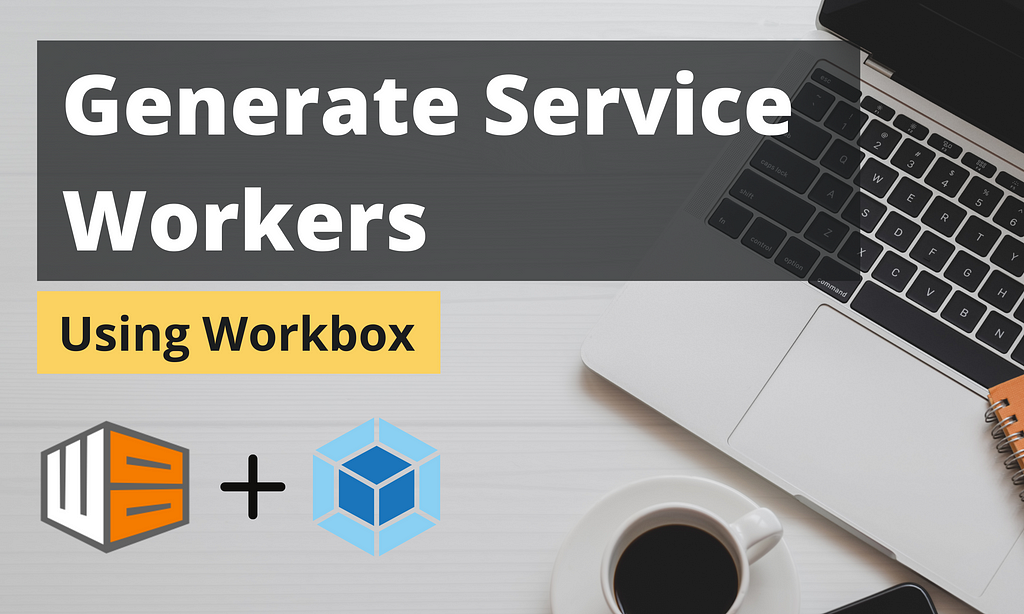
During the past few years, Service Workers emerged to fill the void of background script execution in JavaScript.
With the increasing adoption of Service Workers, many tools and libraries emerged to improve developer productivity. Out of these, one such library is Workbox.
The Workbox
Workbox brings all the best Service Worker creation practices into a single place while removing typical boilerplate work. Besides, you can use it in three different ways.
- Command-line interface — Can be integrated into any application.
- Node.js module —Can use with any build tool such as gulp.
- Webpack plugin — Integrates with projects that use Webpack.
This article will focus on the third approach since Webpack is one of the most used module bundlers.
So, let’s have a look at how we can use Workbox with Webpack.
Workbox with Webpack
To use Workbox with Webpack, you will have to install the workbox-webpack-plugin. You can use either NPM or Yarn for that:
npm i workbox-webpack-plugin // with NPM
yarn add --dev workbox-webpack-plugin // with Yarn
There are two sub plugins implemented as modules within the workbox-webpack-plugin named GenerateSW and InjectManifest.
So out of these, which one should I choose? To answer that, let’s get into their details.
1. GenerateSW Plugin
We can use this plugin to pre-cache files or simple runtime configurations.
However, it won’t work if you need Service Worker features such as Web Push or if you need to import additional scripts with these capabilities.
To use the GenerateSW plugin, you need to integrate it into your Webpack config as follows.
// webpack.config.js:
const { GenerateSW } = require('workbox-webpack-plugin');
module.exports = {
// Other webpack configs...
plugins: [
// Other plugins...
new GenerateSW({
option: 'value',
})
]
};
The object inside the plugin constructor is there for using any configuration option for the GenerateSW plugin. This object determines how Workbox operates at runtime.
Tip: Build & share independent components with Bit
Bit is an ultra-extensible tool that lets you create truly modular applications with independently authored, versioned, and maintained components.
Use it to build modular apps & design systems, author and deliver micro frontends, or simply share components between applications.
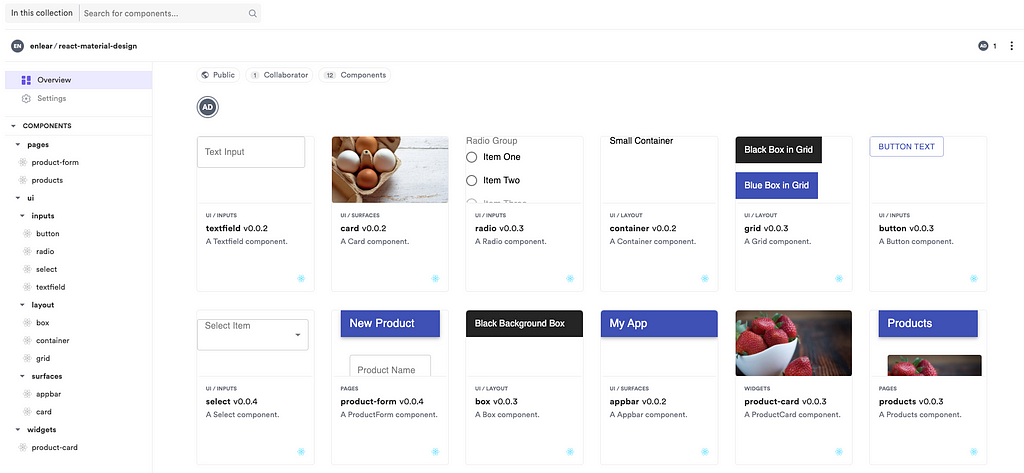
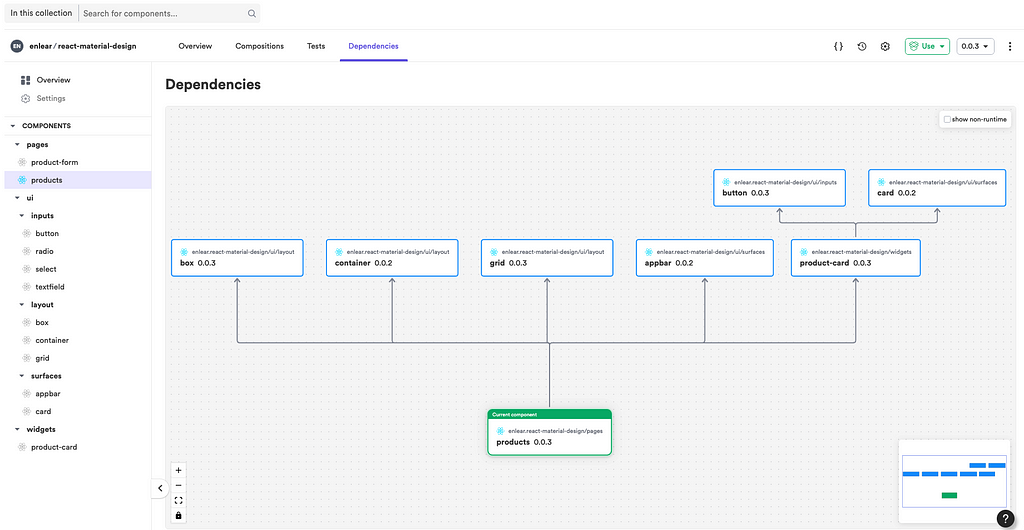
2. Inject Manifest Plugin
If you are looking for the easiest way to add a Service Worker to your website, this is NOT your go-to option. However, this plugin has many advantages. Therefore you should use this if you,
- Need more control over the Service Worker.
- Have more complex routing needs.
- Need to precache files.
- Need to use your Service Worker with other APIs such as Web Push.
Next, let’s take a look at how to use this plugin in your Webpack config.
// webpack.config.js:
const { InjectManifest } = require('workbox-webpack-plugin');
module.exports = {
// Other webpack configs...
plugins: [
// Other plugins...
new InjectManifest({
swSrc: './src/sw.js',
})
]
};
This will create a precache manifest (a list of Webpack assets) and inject it into the Service Worker file via importScripts().
Tip: Workbox revisions each file based on its contents. Therefore, Workbox should always be the last plugin to be called.
Generated Service Worker
Once you configure the suitable sub plugin, Webpack will generate the Service Worker automatically following the basic structure as shown below.
importScripts('workbox-sw.prod.v1.0.1.js');
/**
* DO NOT EDIT THE FILE MANIFEST ENTRY
* ...
*/
const fileManifest = [
{
"url": "/main.css",
"revision": "c751059c834d06d8a066535504616945"
},
{
"url": "/main.js",
"revision": "dbe85ddb2050f016120d2e018a677b40"
}
];
const workboxSW = new self.WorkboxSW();
workboxSW.precache(fileManifest);
Note: This may change based on the different configurations you select.
As you can observe, this includes file revisions as well. If we go into the details, the registered Service Worker will return main.js and main.css on repeated visits. Based on the caching strategy used, the Service Worker will use the hash to check for any changes.
Custom Service Worker
However, if you need to have your own custom Service Worker file, you can enable it using the swSrc option. This will specify the path to your Service Worker file and disable the automatically generated one.
import WorkboxPlugin from 'workbox-webpack-plugin';
module.exports = {
// Other webpack configs...
plugins: [
// Other plugins...
new WorkboxPlugin({
globDirectory: './dist/',
globPatterns: ['**/*.{html,js,css}'],
swSrc: './src/client/service-worker.js',
swDest: './dist/service-worker.js'
}),
],
}
Besides, many other configurations can be included when using the workbox-webpack-plugin. Again, you can find more details in their documentation.
Conclusion
Workbox makes Service Worker creation extremely easy, and it is a tool that will continue getting better.
However, this is not the first Service Worker generation tool that was created. There were tools such as sw-precache for similar purposes. But the Service Worker file created by this is not easily readable.
Thus Workbox not only solves this issue but is also more configurable. Besides, the generated file is smaller in size.
So, I invite you all to try out this plugin when you work with Service Workers next time.
Thanks for reading!
Learn More
- PWA Finding its Way Towards Data-Driven Lite Apps Landscape
- Why learning webpack is important as Front-End Developer
- Setting A React Project From Scratch Using Babel And Webpack
How to Generate Service Workers Automatically was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Viduni Wickramarachchi
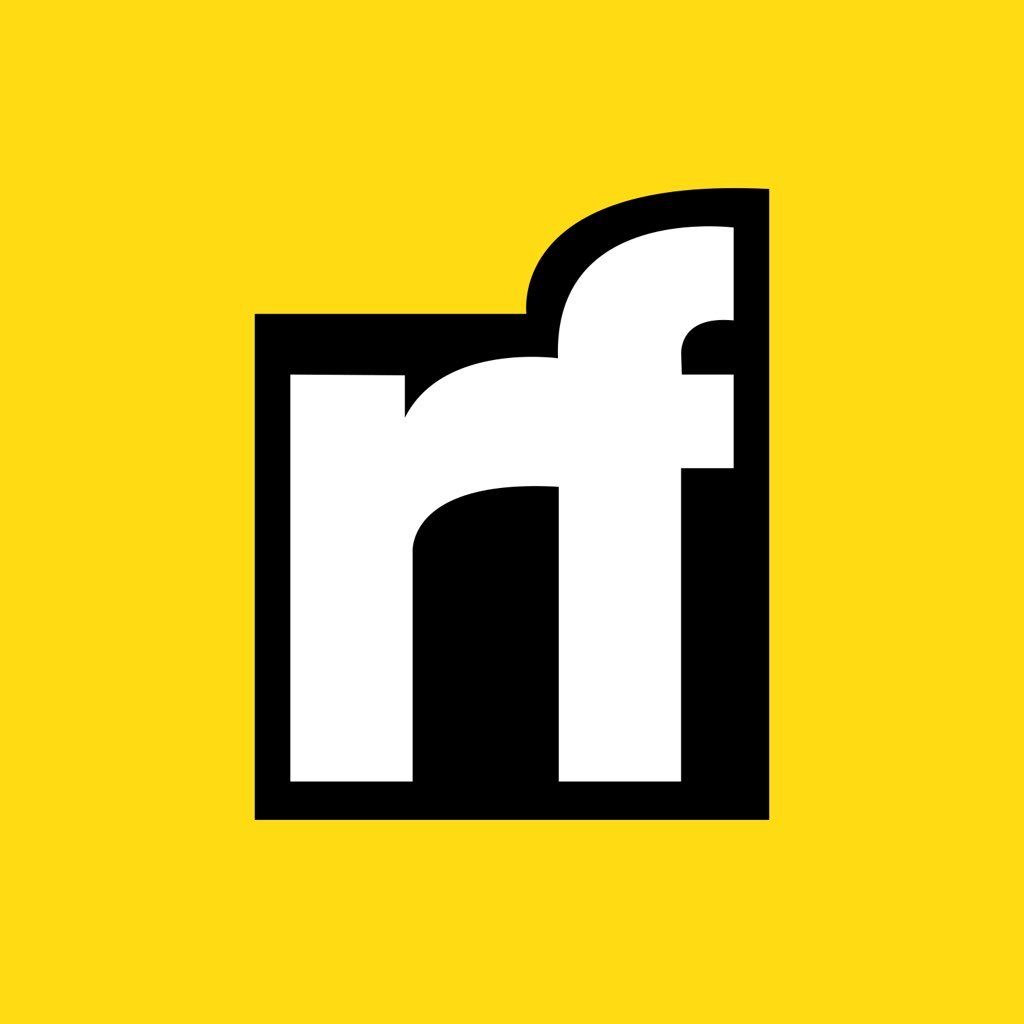
Viduni Wickramarachchi | Sciencx (2021-04-06T23:05:39+00:00) How to Generate Service Workers Automatically. Retrieved from https://www.scien.cx/2021/04/06/how-to-generate-service-workers-automatically/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.