This content originally appeared on Level Up Coding - Medium and was authored by Anello
? Templates for Building Machine Learning Models in Python ? (with Notebook)
I bet you won’t regret it.
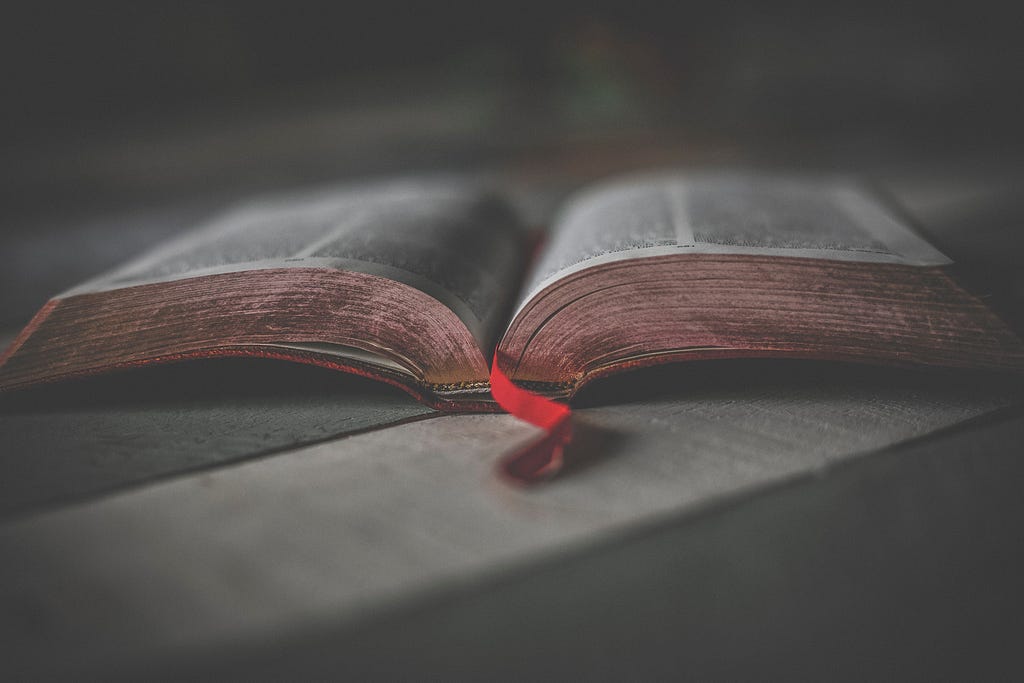
We have seen the categories of supervised, unsupervised, and reinforcement learning — the main machine learning algorithms, and the process of building predictive models. Now we’ll see all of this in practice in the Python language through its main packages and build some machine learning models.
Study Notebook
Visit Jupyter Notebook to see the concepts that will cover how to Build Machine Learning Models.
We will cover all preprocessing, machine learning algorithm training, model evaluation, and new data prediction during the composition of the Machine Learning template. The goal here is to address an introduction to the topic.
Template for Building Machine Learning Models
This notebook contains a template of the code needed to create the main Machine Learning algorithms. Within scikit-learn, we have several algorithms ready. Just adjust the parameters, feed them with the data, do the training, produce the model and finally make predictions.
1. Linear Regression
Summarizing: For linear regression, we need to import the linear_model from the sklearn library. We prepare the training and test data. Then we create the predictive model by instantiating it into an object called linear the LinearRegression algorithm that is a class of the linear_model package. Later we trained the algorithm with the fit function and then used the score to evaluate the model. Finally, we print the coefficients and make new predictions with the model.
# Import modules
from sklearn import linear_model
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create linear regression object
linear = linear_model.LinearRegression()
# Train the model with training data and check the score
linear.fit(x_train, y_train)
linear.score(x_train, y_train)
# Collect coefficients
print('Coefficient: \n', linear.coef_)
print('Intercept: \n', linear.intercept_)
# Make predictions
predicted_values = linear.predict(x_test)
2. Logistic Regression
In this case, the only thing that changes from linear regression to logistic regression is the algorithm we’re going to use. We changed LinearRegression to LogisticRegression.
# Import modules
from sklearn.linear_model import LogisticRegression
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create logistic regression object
model = LogisticRegression()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Collect coefficients
print('Coefficient: \n', model.coef_)
print('Intercept: \n', model.intercept_)
# Make predictions
predicted_vaues = model.predict(x_teste)
3. Decision Trees
Once again, we changed the algorithm to DecisionTreeRegressor:
# Import modules
from sklearn import tree
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted_variable
x_test = test_dataset_precictor_variables
# Create Decision Tree Regressor Object
model = tree.DecisionTreeRegressor()
# Create Decision Tree Classifier Object
model = tree.DecisionTreeClassifier()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
4. Naive Bayes
This time we use the GaussianNB algorithm:
# Import modules
from sklearn.naive_bayes import GaussianNB
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create GaussianNB object
model = GaussianNB()
# Train the model with training data
model.fit(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
5. Support Vector Machines
In this case, we use the SVC class of the SVM library. If it were SVR, it would be a regressor:
# Import modules
from sklearn import svm
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create SVM Classifier object
model = svm.svc()
# Train the model with training data and checking the score
model.fit(x_train, y_train)
model.score(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
6. K-Nearest Neighbors
In the KneighborsClassifier algorithm, we have a hyperparameter called n_neighbors, which is how we make adjustments to this algorithm.
# Import modules
from sklearn.neighbors import KNeighborsClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create KNeighbors Classifier Objects
KNeighborsClassifier(n_neighbors = 6) # default value = 5
# Train the model with training data
model.fit(x_train, y_train)
# Make predictions
predicted_values = model.predict(x_test)
7. K-Means
# Import modules
from sklearn.cluster import KMeans
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create KMeans objects
k_means = KMeans(n_clusters = 3, random_state = 0)
# Train the model with training data
model.fit(x_train)
# Make predictions
predicted_values = model.predict(x_test)
8. Random Forest
# Import modules
from sklearn.ensemble import RandomForestClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Create Random Forest Classifier objects
model = RandomForestClassifier()
# Train the model with training data
model.fit(x_train, x_test)
# Make predictions
predicted_values = model.predict(x_test)
9. Dimensionality Reduction
# Import modules
from sklearn import decomposition
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Creating PCA decomposition object
pca = decomposition.PCA(n_components = k)
# Creating Factor analysis decomposition object
fa = decomposition.FactorAnalysis()
# Reduc the size of the training set using PCA
reduced_train = pca.fit_transform(train)
# Reduce the size of the training set using PCA
reduced_test = pca.transform(test)
10. Gradient Boosting & AdaBoost
# Import modules
from sklearn.ensemble import GradientBoostingClassifier
# Create training and test subsets
x_train = train_dataset_predictor_variables
y_train = train_dataset_predicted variable
x_test = test_dataset_precictor_variables
# Creating Gradient Boosting Classifier object
model = GradientBoostingClassifier(n_estimators = 100, learning_rate = 1.0, max_depth = 1, random_state = 0)
# Training the model with training data
model.fit(x_train, x_test)
# Make predictions
predicted_values = model.predict(x_test)
Therefore, our job will be to transform each block of these algorithms into a project. First, defining a business problem, pre-processing the data, training the algorithm, adjusting hyperparameters, verifiable results, and iterate in this process until we reach a satisfactory accuracy to make the desired predictions.
Thank you.
10 Templates for Building Machine Learning Models with Notebook was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anello
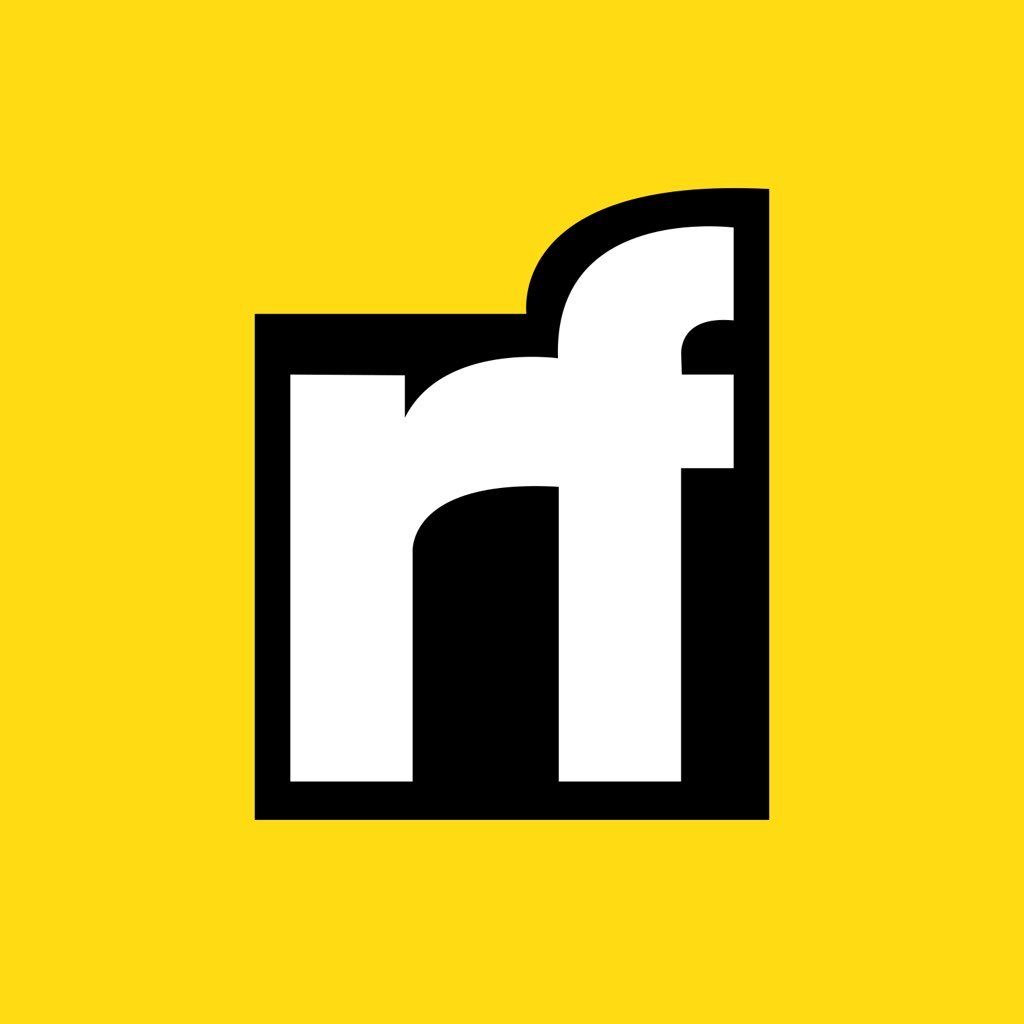
Anello | Sciencx (2021-04-15T14:39:11+00:00) 10 Templates for Building Machine Learning Models with Notebook. Retrieved from https://www.scien.cx/2021/04/15/10-templates-for-building-machine-learning-models-with-notebook/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.