This content originally appeared on Level Up Coding - Medium and was authored by Jennifer Takagi
Welcome to the second post of Haskell series highlights! Today, I will explore functions and pattern matching in this incredible language ?
Some useful links I’ve been using in my studies:
(1) Learning the concepts based on these books: Haskell an introduction to functional programming and Learn You a Haskell for Great Good! ?
(2) Taking notes on this Notion document ?
(3) Coding some exercises on my GitHub repository ?
Functions
Functions are defined as a reusable block code that can be used many times on the same program.
In Haskell, the file extension .hs is where we write functions. The syntax is very simple and clear: funcName parameter1 parameter2 … = execution
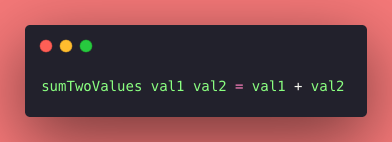
I listed the differences that impacted me as a JavaScript and Python user:
* There is no keyword to define a function, just nominate it and go on.
* There is no comma between parameters or curly brackets involving the block, the language is quite clean. ?
* To define the execution code just use an equal symbol and write what the function does — kind of similar to the arrow function syntax in JS.
Hindley-Milner type system
Hindley-Milner is a type system discovered independently by Roger Hindley and later by Robin Milner. It's the base for the type system of almost every statically typed functional language.
In Haskell, the functions can have types and they can be explicitly declared. Actually, it's a good practice to write the functions’ types, and for that we can use the Hindley-Milner type system to define these types.
For that matter, we can say that the Hindley-Milner type system works like the function documentation. We're able to know which parameter type the function receives and which type it will be returned (the language is able to interpret this information as well, but I still didn't go that far).
In the example below, I used Hindley-Milner on the isVowel function. The function receives a parameter with Char type and returns a Boolean value. The syntax is easy: (functionName :: type -> type).
*“::” can be read as “has type”
** “->” split each parameter, the last type means the returned one.
*** Explicit types are always denoted with the first letter in a capital case.
**** Here's a link so you can read more about the Hindley-Milner type system
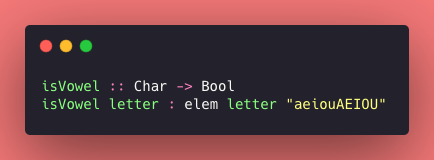
Pattern Matching
Pattern matching consists of specifying patterns to which some data should conform and then checking to see if it does and deconstructing the data according to those patterns. (LIPOVACA, Miran)
This feature is amazing and it’s not exclusive to Haskell. I’ve already used on Elixir, for example. Pattern Matching enables us to define separate function bodies for different patterns, keeping the code simple and readable. ?
On the code below, we can easily create a function to check if a name is valid — verifying if the string is empty or not — , without the control IF/ELSE! ?
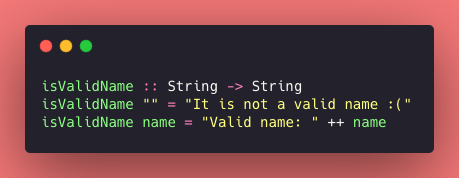
In summary, I wrote the function isValidName behavior in 2 scenarios:
(1) receiving an empty string as the parameter, then returning a message about an invalid name;
(2) receiving any string value — observe that I typed the receiving parameter as a String. If I tried to send a Number instead of a String, it would throw me an error — , then returning a message including the valid name.
* if you don’t care about using the explicit value on the Pattern Matching, you can just give a random name to it. In the example I used “name”, but it could be “x”.
Can you imagine the whole possibility by using Pattern Matching? It helps us to handle different parameters on the same function. Let’s try a little more complex example:
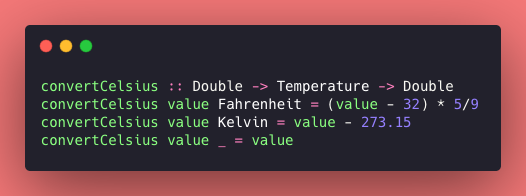
In the above code, our function converts some temperature to Celsius unit. Think about the solution: You need to know which temperature unit the function receives, then apply the conversion according to this. So it could be a classic IF/ELSE usage, but we have Pattern Matching to save the day! ?
The function convertCelsius must handle 3 scenarios:
(1) receiving a Fahrenheit unit as a double type, then applying conversion;
(2) receiving a Kelvin unit as a double type, then applying conversion;
(3) receiving some other unit and just ignore it.
* if you just want to ignore the parameter on Pattern Matching, you should use the symbol “_”.
What a long post! Thanks for reading it and I hope now, just like me, you are able to enjoy Haskell and Functional Programming a little bit more ?
Maybe you are wondering “what about the loops and the conditional statement, Jennifer?” Well, I promise to answer these questions on one of the next posts. See yah and stay safe! ????
Haskell Journey: Functions and Pattern Matching was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Jennifer Takagi
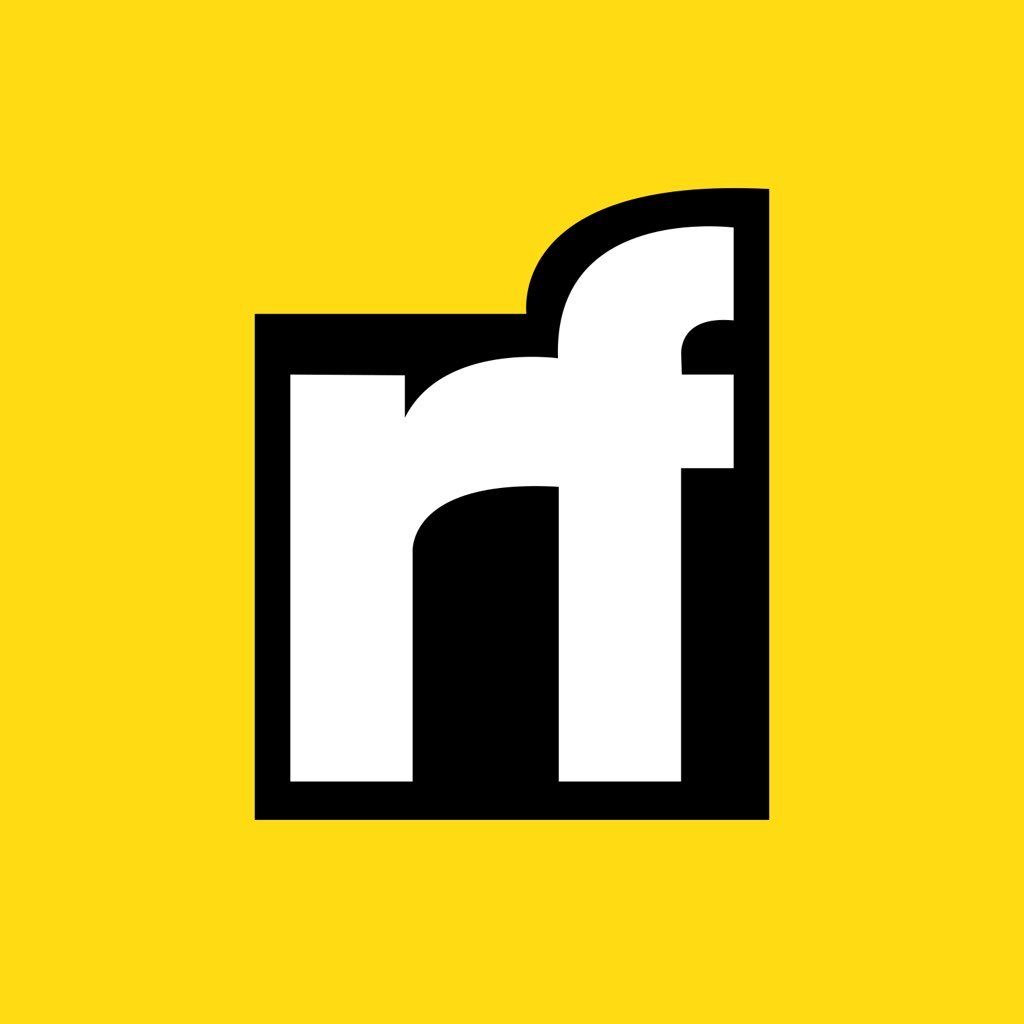
Jennifer Takagi | Sciencx (2021-04-30T01:19:25+00:00) Haskell Journey: Functions and Pattern Matching. Retrieved from https://www.scien.cx/2021/04/30/haskell-journey-functions-and-pattern-matching/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.