This content originally appeared on Level Up Coding - Medium and was authored by Laura Diaz
TypeScript is a programming language developed and maintained by Microsoft. It is a strict syntactical superset of JavaScript and adds optional static typing to the language.
Typescript compiles to Javascript so Node and browsers run javascript and not typescript.
Let’s start with an example: This is a function which adds two values (a and b) both are type number and the function return type is number. In order to run this code in the browser or on Node we have to compile it using the typescript compiler and the typescript compiler will output the javascript we can run in the browser or Node.
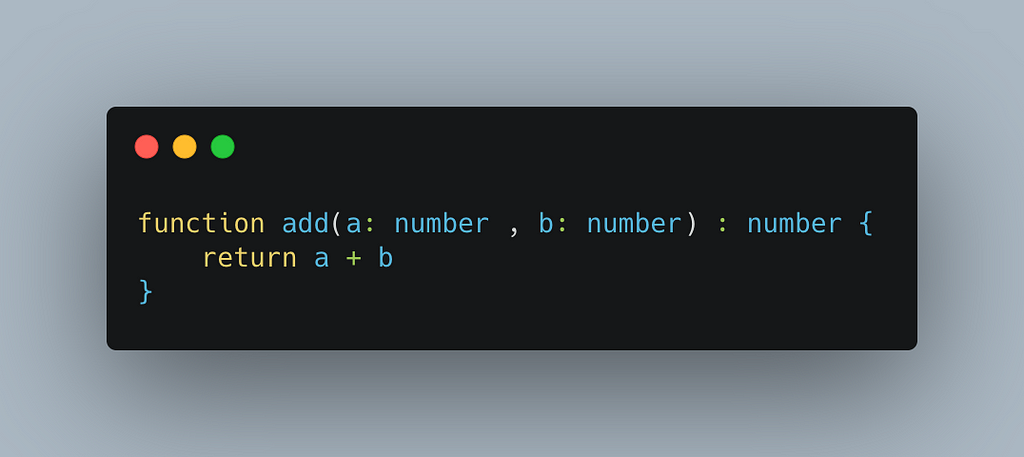
As I mention before, Typescript is a superset of javascript. The types system help us to catch errors in our code before production.
Here’s an example:
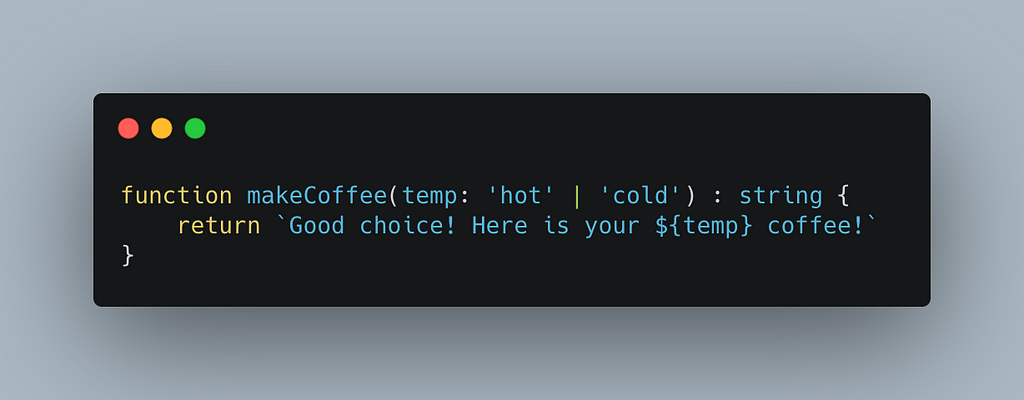
This function must return a string.
If I call makeCoffee with a value that doesn’t expect, for example an object, we will get an error saying that the parameter of type “hot” | “cold” doesn’t expect an object:
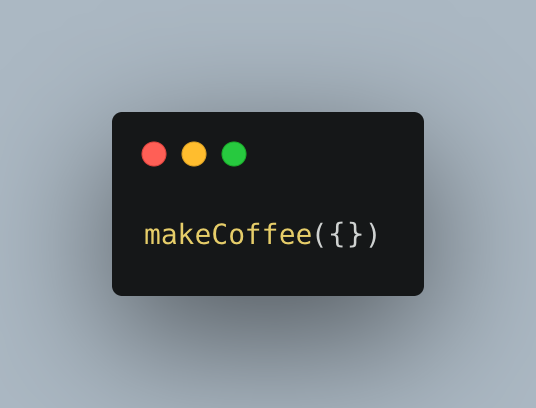
Error: “Argument of type ‘{}’ is not assignable to parameter of type ‘ “hot” | “cold” ’. Type ‘{}’ is not assignable to type ‘ “cold” ’.
How to install typescript and use the typescript compiler?
Typescript compiler is distributed as a NodeJs package so you need to install NodeJs. You can install it from here : https://nodejs.org/es/
To install it we run: npm install typescript
Once it is installed, this package will provide us with the tsc binary that is available to us in the console by running this command: tsc — help .
Now create a folder with mkdir and open the folder in VSCode. Inside create a basic .ts file: hello-world.ts and write the following code:
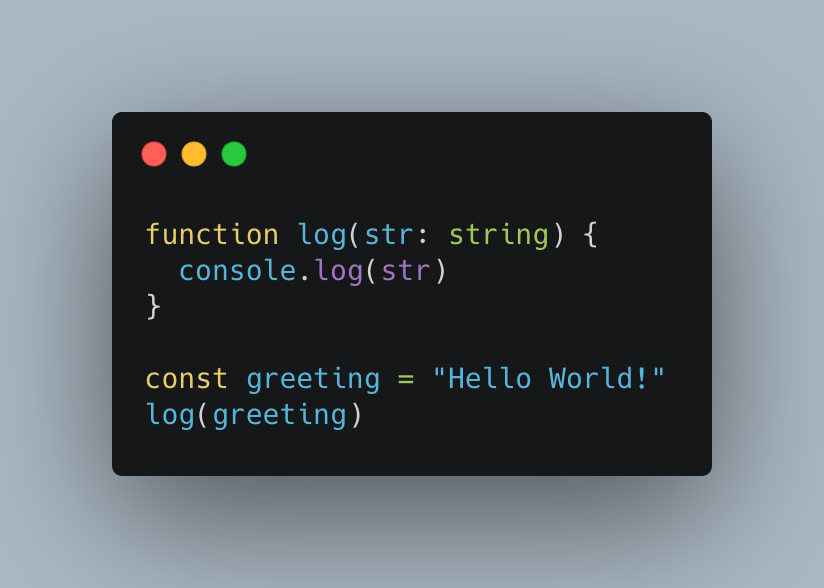
Let’s open the terminal and compile our file, to achieve this, we use tsc command and we specify the path : tsc hello-world.ts
We can also specify different compiler options, like target (the target option tells typescript into what version of javascript we would like to compile our typescript code), to see all the options available: https://www.typescriptlang.org/docs/handbook/compiler-options-in-msbuild.html
If we don’t specify an option typescript will use the default value.
By default it creates the compile file next to the source file, we have the file with the same name but with .js like so: hello-world.js .
Another way to provide options to the typescript compiler is to use a tsconfig.json file. For example like this:
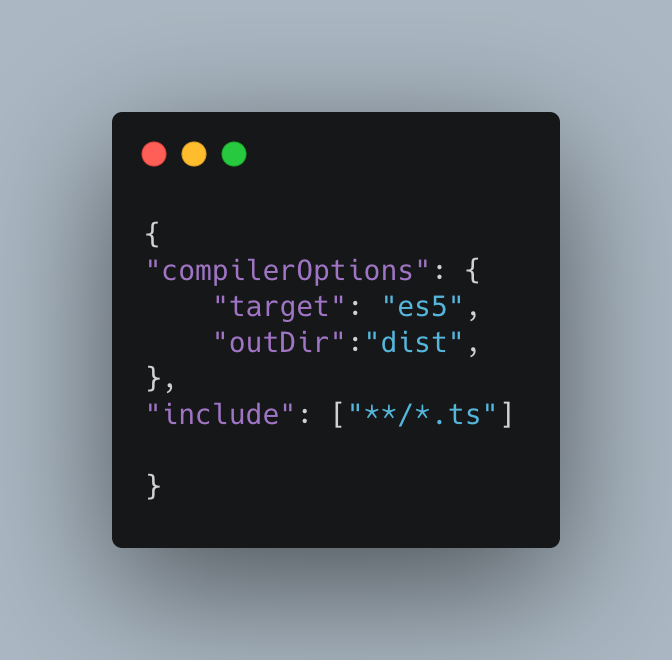
“compilerOptions” : List all the options available to us in this section.
“outDir”: I would use this option to specify the path to the folder where I would like to store the compiled files and it’s called “dist”.
“include”: Specify the files that we want to compile. It’s an array of files and folders.
“**” — any subfolder.
“*” — any file with the .ts extension in the current project.
Now if you run tsc inside the terminal , a folder called “dist” will be created automatically and will store the compiled files inside.
Common TypeScript compiler and tsconfig options:
You can store the TS compiler configuration in the file called “tsconfig.json”. You’ll usually add this file to the root directory of your project, together with the “package.json”.
When you launch the compiler, it reads the “tsconfig.json” from the folder you launched it from, to get the instructions about how to compile your project (e.g., which source files to compile, where to store the output, etc).
The structure of the “tsconfig.json” looks like this:
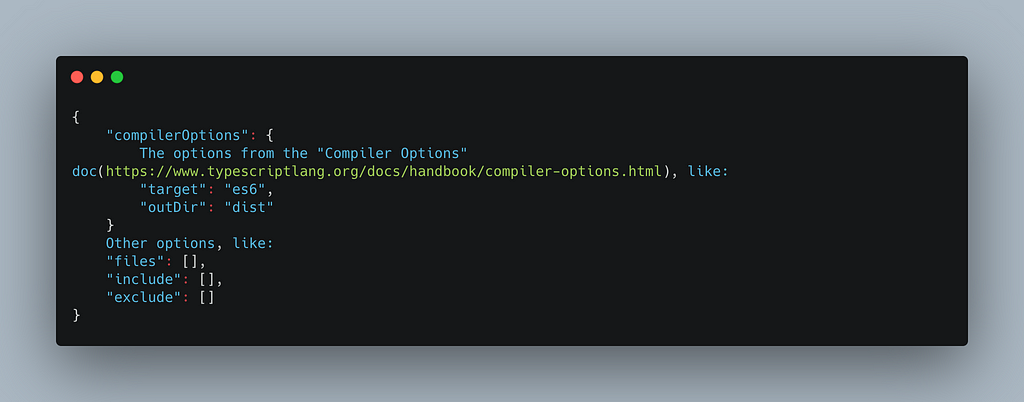
Some of the most common compiler/tsconfig options:
“target”: “es6"
This is the version of ECMAScript you would like to compile your code to. Choose this option based on the environment you would like to run your code in. For example, if you are targeting very old browsers, you might want to choose “ES5”.
“lib”: [“dom”, “dom.iterable”, “esnext”]
This is the list of library files you would like to include during the compilation. Basically, the library files tell the compiler which features you can use in your TypeScript code.
“strict”: true
Enables all strict type checking options, like noImplicitAny, noImplicitThis, alwaysStrict, strictBindCallApply, strictNullChecks, strictFunctionTypes, strictPropertyInitialization. Is recommended to enable this option in order to improve the type safety of your code.
“module”: “commonjs”
This option sets the module system that will be used in the compiled (.JS) files.
“moduleResolution”: “node”
This option defines how the compiler resolves modules — how it looks at the module import statements — how it decides what should be imported given an import statement, like “import a from ‘moduleA’”. The value “node” tells the compiler to mimic the Node.Js module resolution strategy.
“esModuleInterop”: true
This option allows us to import default from commonjs modules which don’t have a default export (modules which didn’t export the “default” property), like React, as if they have it. If we have this option enable we can import React like this : import React from ‘react’;.
“jsx”: “preserve”
We use this option to tell the compiler how to transform the JSX code, “preserve” leaves JSX as is in the compiled files, while the “react” option turns JSX into the React API calls (e.g., React.createElement(‘div’)).
“skipLibCheck”: true
This option tells the compiler whether to type check the declaration(*.d.ts) files (yours and the ones from the third party packages) in your project.
The idea behind this option is to reduce the compile time of a project, by skipping the type checking of the declarations which are already tested by their authors and are known to work correctly.
“files”: [“./file1.ts”, “./file2.d.ts”, …]
We use this option to list the files which the compiler should always include in the compilation. The files included using this option are included regardless of the “exclude” option.
“include”: [“src/**/*”]
We use this option to list the files we’d like to be compiled. While the “files” option requires relative or absolute paths to the files, the “include” option allows glob-like patterns, like:
“**” — any subdirectory
“*” — any file name
“?” — a character followed by the question mark becomes optional (e.g., “src/*.tsx?”)
“exclude”: [“node_modules”, “**/*/*.test.ts”]
This option excludes the files from the compilation. It accepts the same patterns as the “include” option. You can use this option to filter the files specified using the “include” option. The “exclude” option doesn’t affect the “files” option.
This is the 1st part of a series of articles I will be writing on Typescript and Typescript + React ♥️
Thanks for reading,
With love,
Laura.
Info from: https://www.typescriptlang.org/
You can find this article in spanish here: https://lalidiaz.medium.com/breve-introducci%C3%B3n-a-typescript-configuraci%C3%B3n-e0eb0a46a799
Brief introduction to Typescript + config ✨ was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Laura Diaz
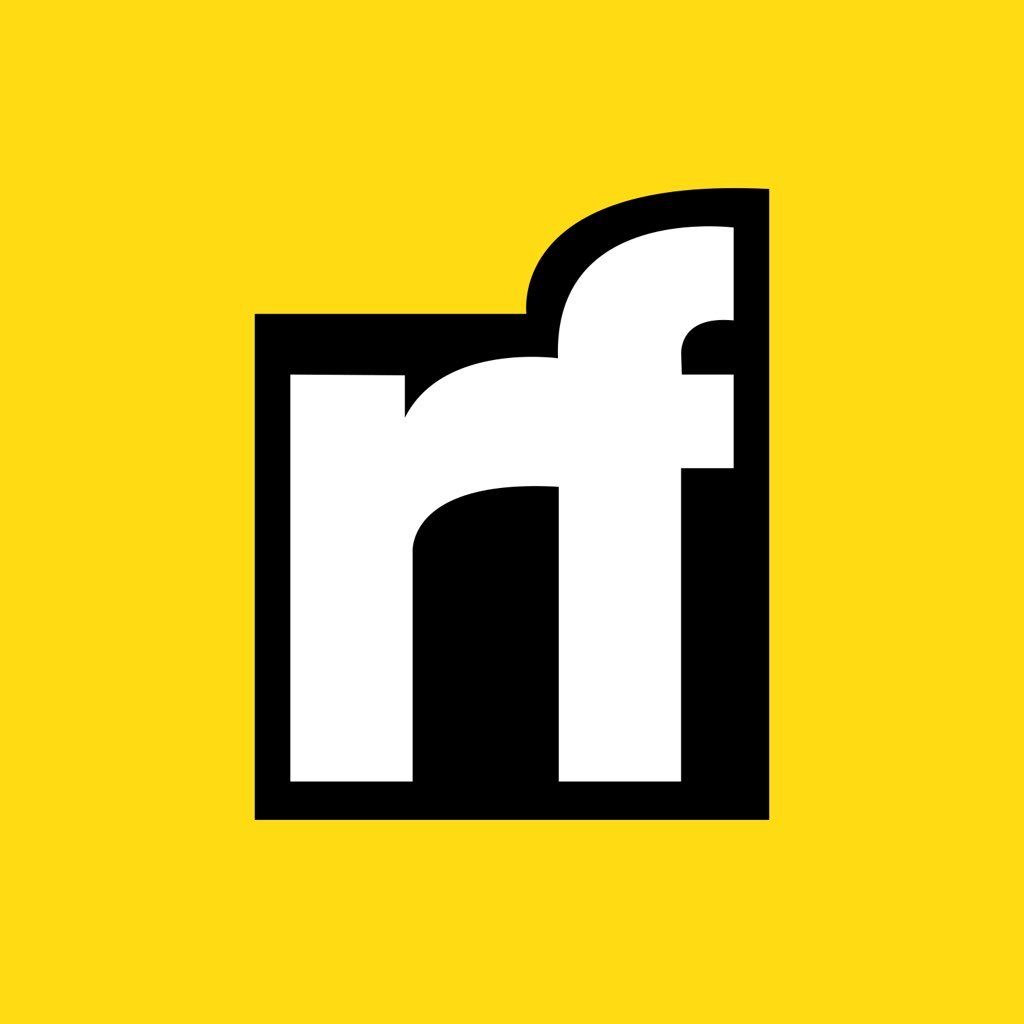
Laura Diaz | Sciencx (2021-05-07T22:00:03+00:00) Brief introduction to Typescript + config ✨. Retrieved from https://www.scien.cx/2021/05/07/brief-introduction-to-typescript-config-%e2%9c%a8/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.