This content originally appeared on DEV Community and was authored by The Nerdy Dev
In the first part of the Hooks article series, we covered about the basic React Hooks in great depth - The useState and the useEffect hooks. In this article, let us cover yet another important topic which is regarding the Context API, a concept that is used in state management in applications. But before we understand what the Context API is, let us first understand why do we need this Context API in the first place.
Need for the Context API
Now it is quite common that you pass data to components through props, but it's always a problem if you forward state through multiple components. This is called as prop drilling which means that there is no direct established connection between the component from where a certain state is present and the component to which we want that state or data to reach. So with prop drilling, we first pass a prop from the source component to an intermediate component and then from that intermediate component we funnel the prop down till it reaches the destination component where it is required. So it is where the React Context comes into picture.
What is React Context ?
React Context is some component-wide under the hood managed form of state storage. So we have this concept of React Context which then allows us to trigger some action in that context where we house our state and methods pertaining to a certain feature of the application and it is then made to directly pass to the component where it is required without building such long prop chains
Using Context
To use Context, you need to do two things
You basically need to provide it (You need to declare globally - "Hey this is the file that houses a specific feature context.". All the components that are wrapped by this feature context will be able to access it.
Besides providing, you also need to setup a way so that you can consume the feature context wherever required. So in essence, you need to hook into it. You need to listen to it.
Step 1: Providing the Context
Providing means that you wrap in JSX code i.e all the components that should be able to tap into that context. Any component that is not wrapped will not be able to listen or subscribe to the Context.
Step 2: Consuming the Context
Next we can also leverage the useContext Hook to consume instead of the Consumer. It allows us to tap into context and listen to changes on it. Remember that our context that we define can be dynamic meaning that we don't just pass data to our components but also the functions which technically of course work with this data.
Remember that in most cases you would just use props to pass data to other components because props are your mechanism to configure components and make them reusable.
Only if you have something which you would forward through a lot of components and you are forwarding it to a component that does something very specific would you use the Context API.
So now let us see an example to understand the Context API:
So inside the App.js file, the very first step is to import React.
Next we setup a piece of state to manage some company details inside the App component. So we first make use of the useState React Hook to setup an initial state companyDetails and we also get the handler (state updating function) to work with the companyDetails state.
Now if you see in the above image, to setup a Provider we first need to create a context so we create CompanyContext and on that we can access the Provider.
To create a Context, all we have to say is :
Next we setup the provider in the App component and we pass a special value prop to which we specify all the properties and functions to the JSX code that gets wrapped by the provider. So all the components (here Company) that are wrapped by the Provider will listen to changes in Context and will directly be able to access the exposed data and functions that we specify in the value prop.
Next let us see how we can consume the Context that was setup.
Consuming Context
So it is the Company Component that we need to define which will access the Context.
So here is the code for the Company Component:
So all we do here is that we setup a Consumer on the CompanyContext and here we have two ways of consuming the context.
Let us first see the first approach :
APPROACH 1
The approach 1 is shown in the above image where we have receive an anonymous arrow function within the Consumer. Since the arrow function represents a JavaScript expression, we need to wrap this in curly braces in our JSX code. Now in the arrow function, all we get is a single context object from which we can destructure the data that we want. So here we want the companyName, employees and teams and it is this data that we render in our Company Component. So we are directly getting this from Context and not via a prop chain.
APPROACH 2
The approach 2 is to make use of the special React Hook - useContext. I strongly recommend that you use useContext Hook for consuming the context instead of APPROACH 1. It is more elegant and quite straight forward. So we get access to the feature context that we have using the useContext hook and directly access the data on the context object.
Feel free to play with the code :
So this is it for the second part of React Hooks article series. In the next article, we will learn about other React Hooks. So stay tuned !
Check out the first article of this React Hooks series:

React Hooks Part 1 - Understanding useState and useEffect Hooks
The Nerdy Dev ・ May 9 ・ 6 min read
If you are looking to learn Web Development, I have curated a FREE course for you on my YouTube Channel, check the below article :

(2021) - Web Developer Full Course : HTML, CSS, JavaScript, Node.js and MongoDB
The Nerdy Dev ・ Apr 28 ・ 2 min read
If you have spare 2 hours, then utilize them by creating these 10 JavaScript Projects in under 2 Hours
?? Follow me on Twitter : https://twitter.com/The_Nerdy_Dev
?? Check out my YouTube Channel : https://youtube.com/thenerdydev
This content originally appeared on DEV Community and was authored by The Nerdy Dev
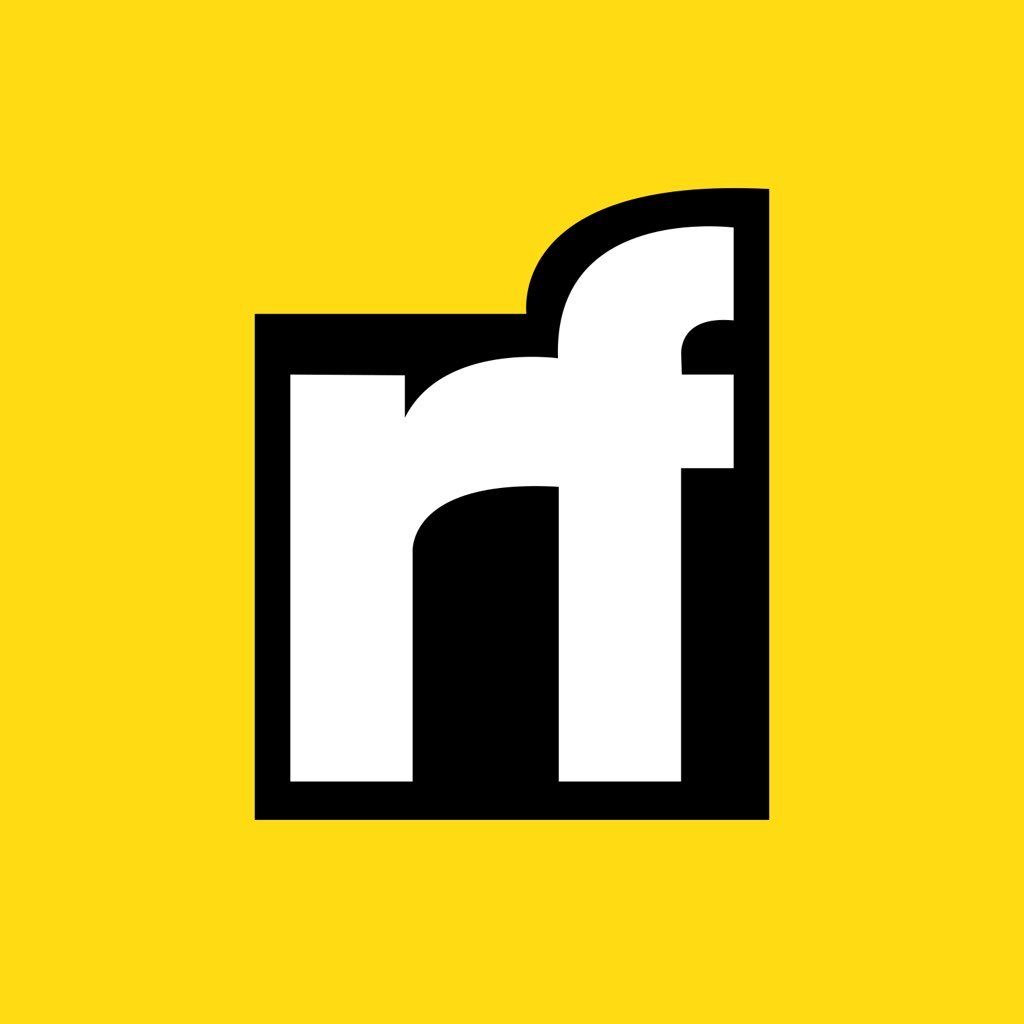
The Nerdy Dev | Sciencx (2021-05-10T10:54:35+00:00) React Hooks Part 2 – Understanding the Context API and The useContext Hook. Retrieved from https://www.scien.cx/2021/05/10/react-hooks-part-2-understanding-the-context-api-and-the-usecontext-hook/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.