This content originally appeared on DEV Community and was authored by Ushieru Kokoran
La forma más sencilla de tener control en nuestros diseños es imaginar que dividimos nuestra pantalla entre 100 bloques iguales, a lo ancho y a lo alto. De esta manera podremos definir que widgets abarquen un 10% de ancho o un 35% de alto.
La siguiente clase me ayuda a definir los tamaños.
import 'package:flutter/widgets.dart';
class SizeConfig {
static double screenWidth = 0;
static double screenHeight = 0;
static double _blockSizeHorizontal = 0;
static double _blockSizeVertical = 0;
static double _safeAreaHorizontal = 0;
static double _safeAreaVertical = 0;
static double _safeBlockHorizontal = 0;
static double _safeBlockVertical = 0;
SizeConfig(BuildContext context) {
var mediaQueryContext = MediaQuery.of(context);
screenWidth = mediaQueryContext.size.width;
screenHeight = mediaQueryContext.size.height;
_blockSizeHorizontal = screenWidth / 100;
_blockSizeVertical = screenHeight / 100;
_safeAreaHorizontal =
mediaQueryContext.padding.left + mediaQueryContext.padding.right;
_safeAreaVertical =
mediaQueryContext.padding.top + mediaQueryContext.padding.bottom;
_safeBlockHorizontal = (screenWidth - _safeAreaHorizontal) / 100;
_safeBlockVertical = (screenHeight - _safeAreaVertical) / 100;
}
static double blockSizeHorizontal(double porcent) {
return _blockSizeHorizontal * porcent;
}
static double blockSizeVertical(double porcent) {
return _blockSizeVertical * porcent;
}
static double safeBlockSizeHorizontal(double porcent) {
return _safeBlockHorizontal * porcent;
}
static double safeBlockSizeVertical(double porcent) {
return _safeBlockVertical * porcent;
}
}
Para inicializarla solo debes inicializar el constructor de SizeConfig en tu initialRoute o en tu home, dependiendo que utilices. Solo recuerda inicializarlo en el primer Widget consecuente de tu MaterialApp.
class Ushieru extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Ushieru ??',
theme: ThemeData(
primarySwatch: Colors.blue,
),
routes: {
IndexScreen.routeName: (context) => IndexScreen(),
},
initialRoute: IndexScreen.routeName,
home: IndexScreen(),
);
}
}
Ejemplo:
class IndexScreen extends StatelessWidget {
static final routeName = "IndexScreen";
@override
Widget build(BuildContext context) {
SizeConfig(context);
return Container(
width: SizeConfig.screenWidth, // Toma todo el ancho de la pantalla
height: SizeConfig.blockSizeVertical(50), // Toma el 50% del alto de la pantalla
);
}
}
Al ser estáticas las variables de clase estas podrán ser utilizadas por cualquier otro widget a partir solo del nombre de la clase SizeConfig.
class SecondScreen extends StatelessWidget {
static final routeName = "SecondScreen";
@override
Widget build(BuildContext context) {
// SizeConfig(context); No se necesita inicializar otra vez
return Container(
width: SizeConfig.screenWidth,
height: SizeConfig.blockSizeVertical(50),
);
}
}
¿Y que pasa si utilizo un SafeArea para mi aplicación?
También ya está previsto solo cambias del método blockSizeVertical a safeBlockSizeVertical, asi de simple.
class ThirdScreen extends StatelessWidget {
static final routeName = "ThirdScreen";
@override
Widget build(BuildContext context) {
return SafeArea(
child: Container(
width: SizeConfig.screenWidth,
height: SizeConfig.safeBlockSizeVertical(50), // Usamos el metodo safeBlockSizeVertical
),
);
}
}
This content originally appeared on DEV Community and was authored by Ushieru Kokoran
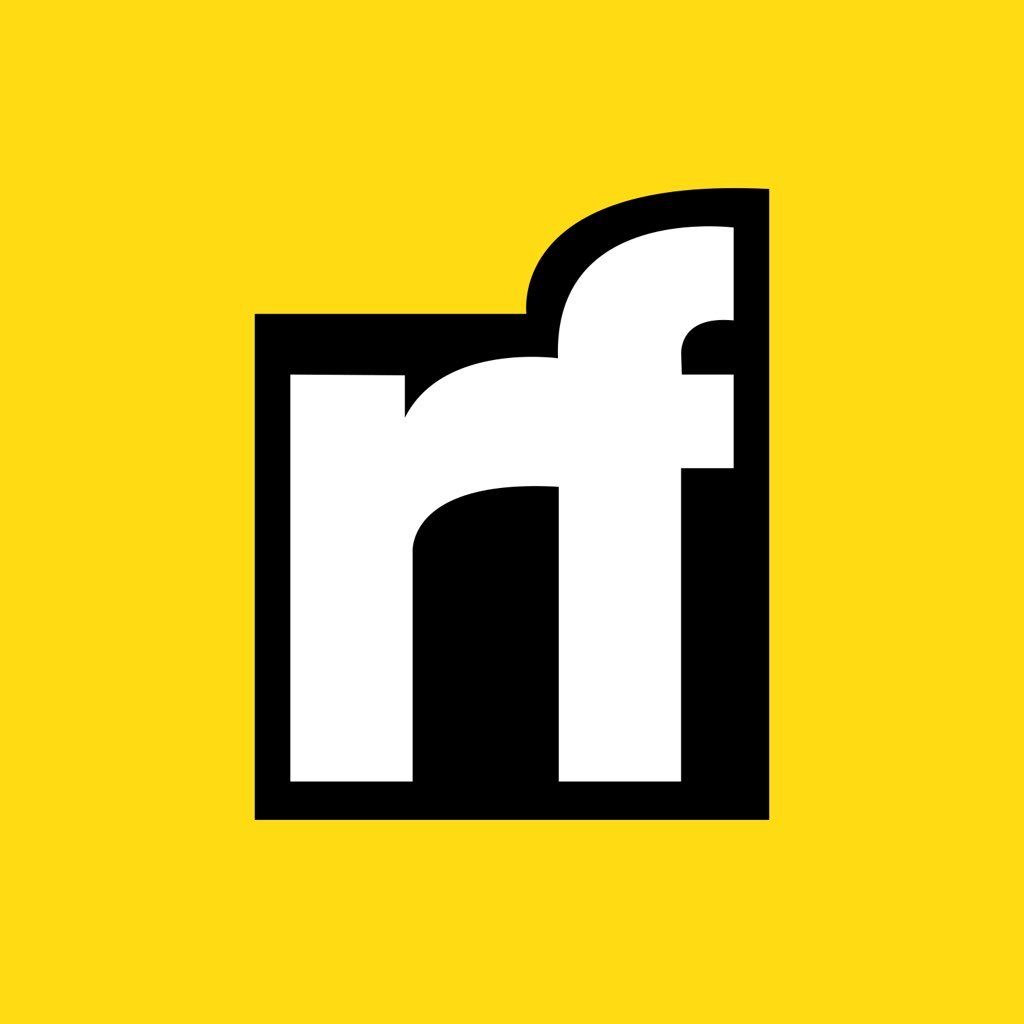
Ushieru Kokoran | Sciencx (2021-05-27T05:05:44+00:00) Responsive in flutter. Retrieved from https://www.scien.cx/2021/05/27/responsive-in-flutter/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.