This content originally appeared on DEV Community and was authored by Shreyansh Sinha
Scope of var
Scope essentially means where these variables are available for use. var declarations are globally scoped or function/locally scoped.
The scope is global when a var variable is declared outside a function. This means that any variable that is declared with var outside a function block is available for use in the whole window.
var is function scoped when it is declared within a function. This means that it is available and can be accessed only within that function.
var variable1 = "outside";
function func() {
var variable2 = "inside";
}
console.log(variable1) //correct
console.log(variable2) //error
Here variable1 has global scope while variable2 has local/function scope.
var variables can be re-declared and re-updated.
Eg:
var variable1 = "first"; //correct
var variable1 = "second"; //correct
var variable1 = "initial"; //correct
variable1 = "final"; //correct
Hoisting of var
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their scope before code execution.
Eg:
console.log(name)
var name = "shreyansh";
would be interpreted as
var name
console.log(name) //name is undefined
name = "shreyansh"
Another Example:
getName();
console.log(x);
var x = 7;
function getName () {
console.log('shreyansh');
}
is actually interpreted as
function getName() {
console.log('shreyansh');
}
var x;
getName(); // shreyansh
console.log(x); // undefined
x = 7;
var variables are hoisted to the top of their scope and initialized with a value of undefined.
let
Let is block scoped. A block is a chunk of code bounded by {}. A block lives in curly braces. Anything within curly braces is a block.
let greeting = "Hello, myself Shreyansh";
let value = 4;
if (value > 3) {
let hello = "hello everyone";
console.log(hello);// "hello everyone"
}
console.log(hello) // hello is not defined
Let can be updated but not redeclared.
Correct:
let greeting = "say Hi";
greeting = "say Hello instead";
Incorrect:
let greeting = "say Hi";
let greeting = "say Hello instead"; // error: Identifier 'greeting' has already been declared.
However, if the same variable is defined in different scopes, there will be no error:
let greeting = "say Hi";
if (true) {
let greeting = "say Hello instead";
console.log(greeting); // "say Hello instead"
}
console.log(greeting); // "say Hi"
Why is there no error?
This is because both instances are treated as different variables since they have different scopes.
Hoisting of let
Just like var, let declarations are hoisted to the top. Unlike var which is initialized as undefined, the let keyword is not initialized. So if you try to use a let variable before its declaration, you'll get a Reference Error.
const
Like let declarations, const declarations can only be accessed within the block they were declared.
const cannot be updated or re-declared.
This means that the value of a variable declared with const remains the same within its scope. It cannot be updated or re-declared.
However, this is not true for objects. While a const object cannot be updated, the properties of these objects can be updated.
const greeting = {
message: "say Hi",
times: 4
}
Wrong
greeting = {
words: "Hello",
number: "five"
} // error: Assignment to constant variable.
Correct
greeting.message = "say Hello instead";
Hoisting of const
Just like let, const declarations are hoisted to the top but are not initialized.
This content originally appeared on DEV Community and was authored by Shreyansh Sinha
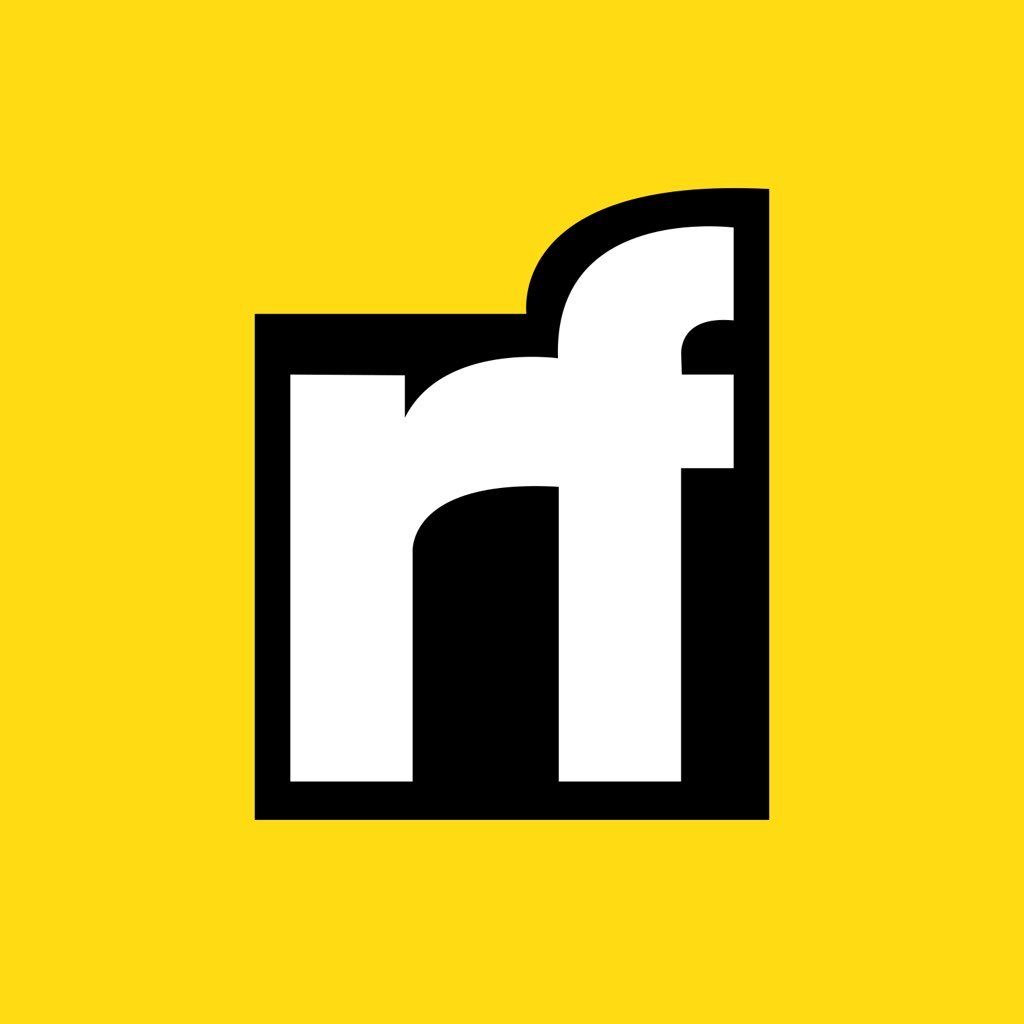
Shreyansh Sinha | Sciencx (2021-06-01T10:45:01+00:00) let, var & const explained.. Retrieved from https://www.scien.cx/2021/06/01/let-var-const-explained/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.