This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
This tutorial belongs to the Swift series
Sets are used to create collections of non-repeated items.
While an array can contain many times the same item, you only have unique items in a set.
You can declare a set of Int values in this way:
let set: Set<Int> = [1, 2, 3]
or you can initialize it from an array:
let set = Set([1, 2, 3])
Add items to the set using insert()
:
var set = Set([1, 2, 3])
set.insert(17)
Unlike arrays, there is no order or position in a set. Items are retrieved and inserted randomly.
The way to print the content of a set ordered is to transform it into an array using the sorted()
method:
var set = Set([2, 1, 3])
let orderedList = set.sorted()
To check if a set contains an element, use the contains()
method:
var set = Set([1, 2, 3])
set.contains(2) //true
To get the number of items in the set, use the count
property:
let set = Set([1, 2, 3])
set.count //3
If a set is empty, its isEmpty
property is true
.
let set = Set([1, 2, 3])
set.isEmpty //false
To remove one item from the array, use remove()
passing the value of the element:
var set = Set([1, 2, 3])
set.remove(1)
//set is [2, 3]
To remove all items from the set, you can use removeAll():
set.removeAll()
Sets, like arrays, are passed by value, which means if you pass an array to a function, or return it from a function, the set is copied.
Sets are great to perform set math operations like intersection, union, subtracting, and more.
These methods help with this:
intersection(_:)
symmetricDifference(_:)
union(_:)
subtracting(_:)
isSubset(of:)
isSuperset(of:)
isStrictSubset(of:)
isStrictSuperset(of:)
isDisjoint(with:)
Sets are collections, and they can be iterated over in loops.
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
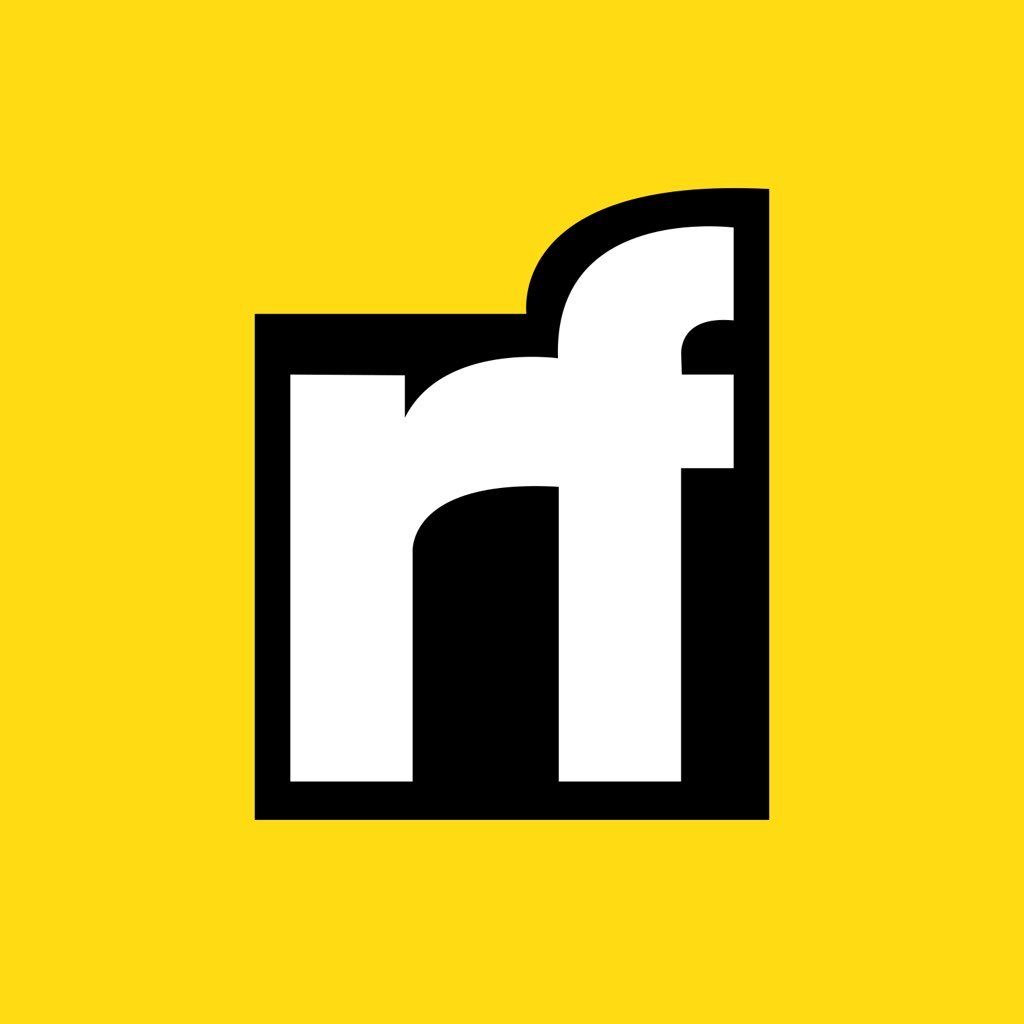
flaviocopes.com | Sciencx (2021-06-04T05:00:00+00:00) Swift Sets. Retrieved from https://www.scien.cx/2021/06/04/swift-sets/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.