This content originally appeared on DEV Community and was authored by Muhammad Faran Aiki
Credential: I do not know, but I, at least, am 4 years experienced; I am still learning C and Assembly.
What is any
and all
in Python?
By Python definition, any
will return if there is an item whereby true in a list, whereas all
will return if all items are true in a list.
It looks like the operator or
and and
for any
and all
. It is actually easy to implement in C, Assembly, or any other language.
Here are examples of the function any
,
any([true, false, false]) # Result in True
any([false, false, false]) # Result in False
Here are examples of the function all
,
all([true, true, true]) # Result in True
all([true, true, false]) # Result in False
Implementation in C for any
,
// My algorithm for "any"
int any(int* arr, int size) {
int i = 0, t = 0;
for (; i < size - 1 ; i += 2) {
t += arr[i] + arr[i + 1];
}
return (t + arr[size % i]) && 1;
}
With a O(log n)
time complexity and O(1)
space complexity.
Implementation in C for all
,
// My algorithm for "all"
int all(int* arr, int size) {
int i = 0, t = 0;
for (; i < size - 1 ; i += 2) {
t += arr[i] + arr[i + 1];
}
return (t + (i % size && arr[size % i]) > (i >> 1));
}
With a O(log n)
time complexity and O(1)
space complexity.
I will explain how the algorithm works in another post.
How to use any
,
# Real world application
if any(person.alive for person in people):
myself.shout("Who is dead?")
How to use all
,
# Real world application
if all(person.alive for person in people):
myself.shout("We are safe!")
This content originally appeared on DEV Community and was authored by Muhammad Faran Aiki
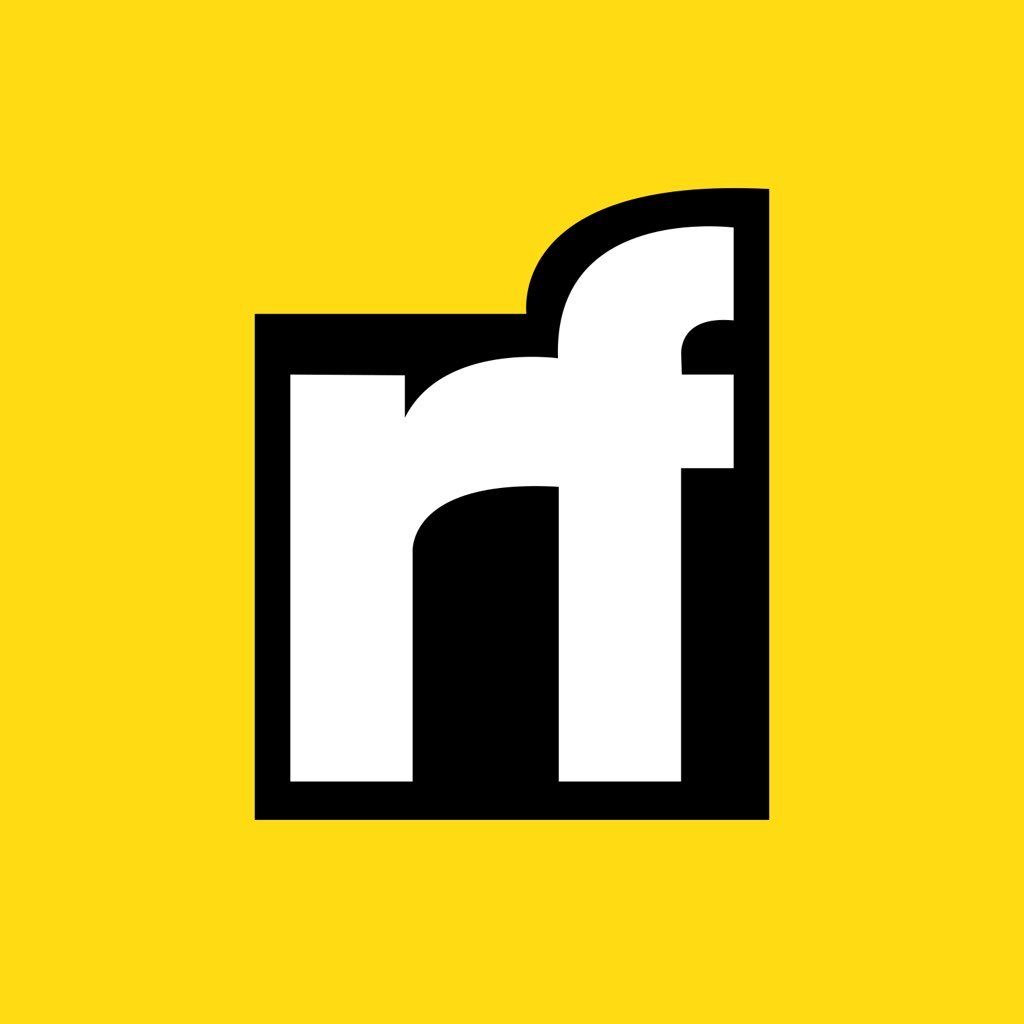
Muhammad Faran Aiki | Sciencx (2021-06-16T15:22:59+00:00) How to use “any” and “all” in Python. Retrieved from https://www.scien.cx/2021/06/16/how-to-use-any-and-all-in-python/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.