This content originally appeared on DEV Community and was authored by Ata Parvin Ghods
Sometimes we need to hide pages/routes from the user and disabling their accessibility to those pages.
In this post I want to show you the easiest way(which I think it is) to do this.
1.I will start with create-react-app
// Create a new app
npx create-react-app route-app react-router-dom
// Run the created app
cd route-app
yarn start
// http://localhost:3000
2.In our main component(App.js) we will define our routes
(src/App.js)
import ProtectedRoute from './ProtectedRoute'
import { BrowserRouter, Switch, Route } from 'react-router-dom'
export default App = () => {
return(
<BrowserRouter>
<Switch>
<Route exact path='/posts' component={Home} />
<ProtectedRoute exact path='/login' component={Login} />
<Route path='/' component={NotFound} />
//this is 404 page btw - no "exact" prop
</Switch>
</BrowserRouter>
)
}
As you can see we will create our route with a custom route component, So let's build that.
3.Our ProtectedRoute component
(src/ProtectedRoute.js)
import React from 'react'
import { Redirect, Route } from 'react-router'
export const ProtectedRoute = ({ component: Component, ...rest}) => {
const userIsLoggedIn = true //our user logged in
return(
<Route
{...rest}
render={(props) => {
if(!userIsLoggedIn){
return <Component {...props}/>
} else {
return <Redirect to={{ pathname: '/', state: {from: props.location }}} />
}
}}
/>
)
}
If our user is not logged in then show the Component(which is loggin page in this case because we don't want logged in user see the login in page), If not Redirect the user to home page.
This is how we protect route with react-router-dom
In Next Js we use HOC(high-order-component)
1.Create next app
// Create a new app
npx create-next-app route-app
// Run the created app
cd route-app
yarn dev
// http://localhost:3000
2.First We create HOC
(src/HOC/ProtectedRoute)
import { useRouter } from "next/router"
const ProtectedRoute = (ProtectedComponent) => {
return (props) => {
if (typeof window !== "undefined") {
const Router = useRouter()
const userIsLoggedIn = true
if (userIsLoggedIn) {
Router.replace("/")
return null
} else {
return <ProtectedComponent {...props} />
}
}
return null
}
}
export default ProtectedRoute
In this case we say if the user is logged in then redirect to home page else return the component
3.Make our page protected
(src/pages/login.js)
import ProtectedRoute from "../HOC/ProtectedRoute"
const Login= () => {
return (
<div>
<a>Login Page</a>
</div>
)
}
export default ProtectedRoute(Login) //wrap our component in hoc
That's all you need! Thank you
This content originally appeared on DEV Community and was authored by Ata Parvin Ghods
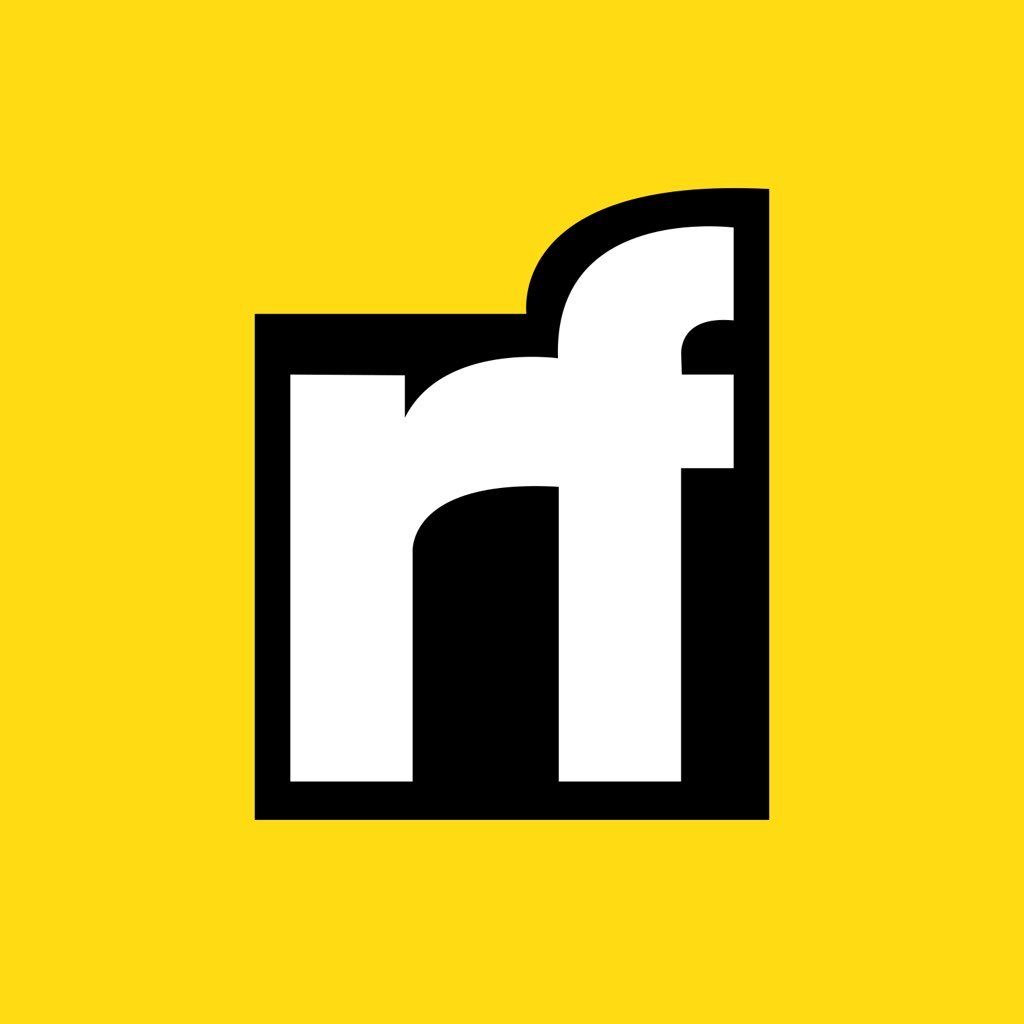
Ata Parvin Ghods | Sciencx (2021-06-18T00:47:15+00:00) Protected Routes (Next js & CRA) simple but efficient. Retrieved from https://www.scien.cx/2021/06/18/protected-routes-next-js-cra-simple-but-efficient/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.