This content originally appeared on Level Up Coding - Medium and was authored by Leonard Yeo
An Easy Guide to Learn Abstract Factory in Golang
An Abstract Factory design pattern solves the problem of creating entire product groups without specifying their concrete classes.
Problem

Imagine that you are creating an Sports Attire shop. Your code could consist of classes that represent:
- A group of related products: Shirt + Short
- Variants of this group. For example, products Shirt + Short are avalable in these variants: Brand A + Brand B
Customers are unhappy when they receive non-matching sports attire. There needs to be a way to create individual sport attire objects so that they match other objects of the same group.
Then there is another problem where sport attire vendors update their catalog often and you wouldn’t want to change the code source code each time it happens.
The following is a solution…
Solution
- explicitly declare interfaces for each distinct product of the product group
- then make all variants of products follow those interfaces
- for example, all shorts variants can implement the Short interface and etc

- declare the Abstract Factory — an interface with a list of creation methods for all products that are part of the product group (for example, makeShirt, makeShort and etc)
- these methods must return abstract product types represented by the interfaces extracted previously: Shirt, Short and etc

- For each variant of a product group, create a separate factory class based on the AbstractFactory interface
- The client code has to work with both factories and products via their respective abstract interfaces
- This allows changes to the type of a factory that pass to the client code, as well as the product variant that the client code receives, without breaking the actual client code
Diagram

Pros and Cons
And of course, the abstract factory design pattern has its own tradeoff. Here are some pros and cons of this design pattern.
Pros
- The products from a factory are compatible with each other
- Avoid tight coupling between concrete products and client code
- Fulfills Single Responsibility Principle
- Fulfills Open/Closed Principle
Cons
- Code may become complicated than it should be
How to code a simple Abstract Factory in Golang?
iShoe Interface
Declare a interface that would implement functionalities of the shoe.
iShort Interface
Declare a interface that would implement functionalities of the short.
Brand A Shoe
Brand A Short
Brand A Concrete Factory
Brand B Shoe
Brand B Short
Brand B Concrete Factory
Sports Attire Factory Interface
Main
Explanation
- define an interface for creating all distinct products but leaves the actual product creation to concrete factories
- each factory type has a close similarity to a product variety
- the main will call the creation methods of a factory object instead of creating the products directly
- since each factory type has a close similarity to a product variety, all its products will be compatible
- the main work with factories and products only through their abstract interfaces
How to run
Command:go run .
# go run .
Logo: brandB
Size: 14
Logo: brandB
Size: 14
Logo: brandA
Size: 14
Logo: brandA
Size: 14
Takeaways
I hope you understand how to code a simple abstract factory in Golang and more importantly understand how a abstract factory design pattern can help you to design your code better and to ensure maintainability.
Here the link to my github page for all source code on abstract factory design pattern in Golang: https://github.com/leonardyeoxl/go-patterns/tree/update_creational_patterns/creational/abstract_factory
In the next article, I might explore chain of responsibility design pattern written in Golang. So stay tuned! Peace!✌️
A Easy Guide to Learn Abstract Factory in Golang was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Leonard Yeo
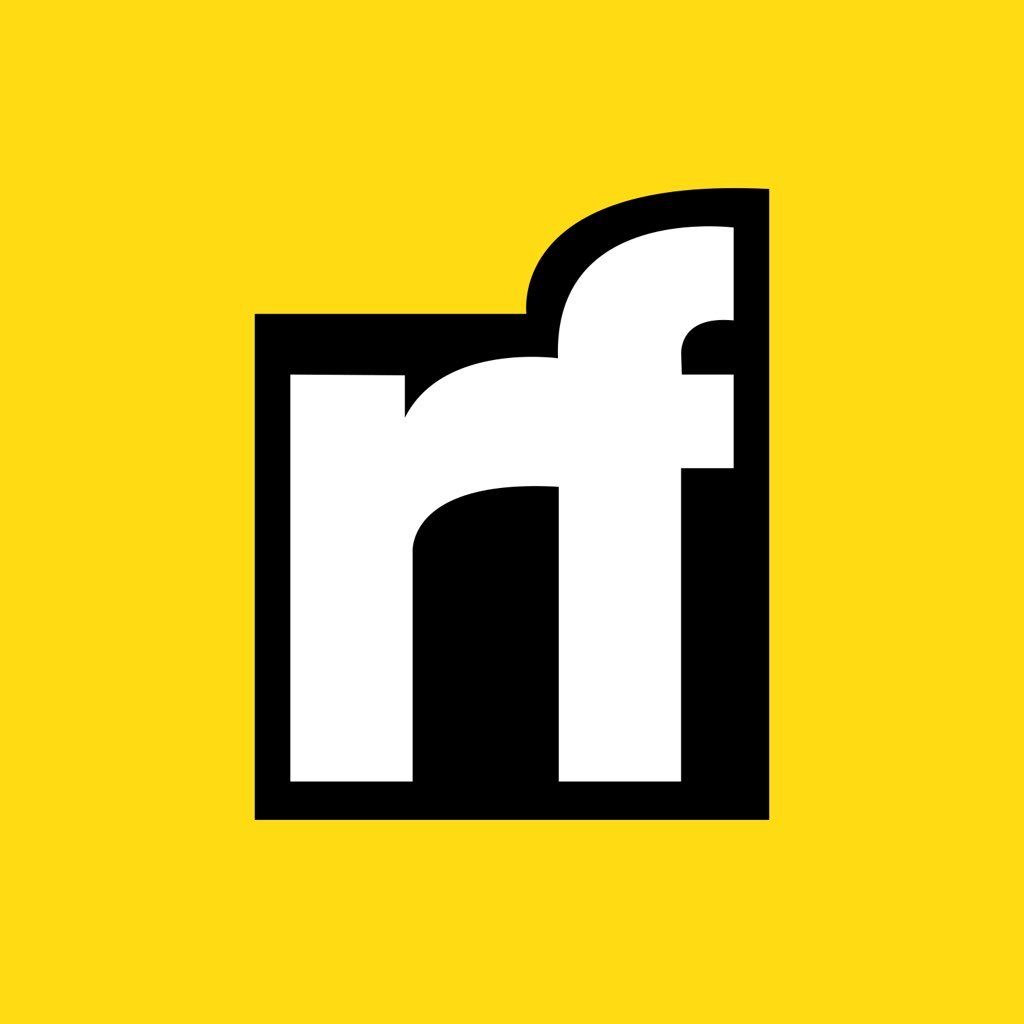
Leonard Yeo | Sciencx (2021-06-27T18:19:50+00:00) A Easy Guide to Learn Abstract Factory in Golang. Retrieved from https://www.scien.cx/2021/06/27/a-easy-guide-to-learn-abstract-factory-in-golang/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.