This content originally appeared on Level Up Coding - Medium and was authored by Zayn Korai
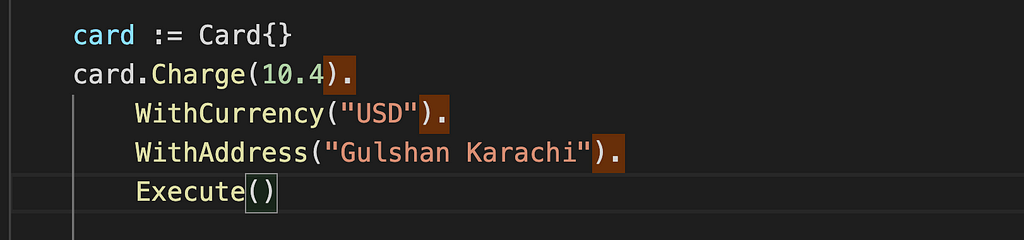
Method Chaining is the practice of calling different methods in a single expression instead of calling different methods with the same object reference separately. As given below
dialogue := AlrtDilagoue{}
dialogue.SetTitle(" Warning ").
SetMessage("Do you really want to delete this ?").
Show();
Why is method chaining preferred?
I will say it depends on developers as well and we see it in other languages like java, c#, php quite often. In Go libraries like GORM rely on method chaining in order to build more complex SQL queries.
Some developers promote method chaining as a good practice that improves the readability of source code. Redundant temporary variables and code repetitions are eliminated an expression becomes easy to read from left to right as natural language texts.
And for some others method chaining is often referred to as a bad practice. In the thread on StackOverflow i.e Method chaining — why is it a good practice, or not? many posts claim that method chaining worsens the readability.
But still method chaining is heavily used in ORM’s.
Implementing Method chaining in Golang
Lets try to implement it anyway, because I like it for few cases like writing a wrapper on API’s.
package main
import "fmt"
type Card struct {
amount float64
currency string
address string
}
func (c *Card) Charge(amount float64) *Card {
c.amount = amount
return c
}
func (c *Card) WithCurrency(currency string) *Card {
c.currency = currency
return c
}
func (c *Card) WithAddress(address string) *Card {
c.address = address
return c
}
func (c *Card) Execute() {
fmt.Printf("Dear Customer, \n%s %v is Debited from your account ", c.currency, c.amount)
}
To make a method a chain-able in Golang all you have to do is return a receiver. It’s not necessary to return anything on last method you are using in chain like as Execute() method in the code
func main() {
card := Card{}
card.Charge(10.4).
WithCurrency("USD").
WithAddress("Gulshan Karachi").
Execute()
// you can write it as below as well in a single line
card.Charge(10.4).WithCurrency("PKR").WithAddress("Gulshan Karachi").Execute()
}
Does it has some draw back in Golang?
Yes error handling in method isn’t straight forward while Chain Forwarding ain Go as it returns an error.
Languages like Java, Python can get away with it is because they idiomatically communicate errors via exceptions and exceptions stop the method chain immediately and jump to the relevant exception handler.
If this post was helpful, please click the clap ? button below a few times to show your support for the author! ⬇
Method Chaining In Golang was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Zayn Korai
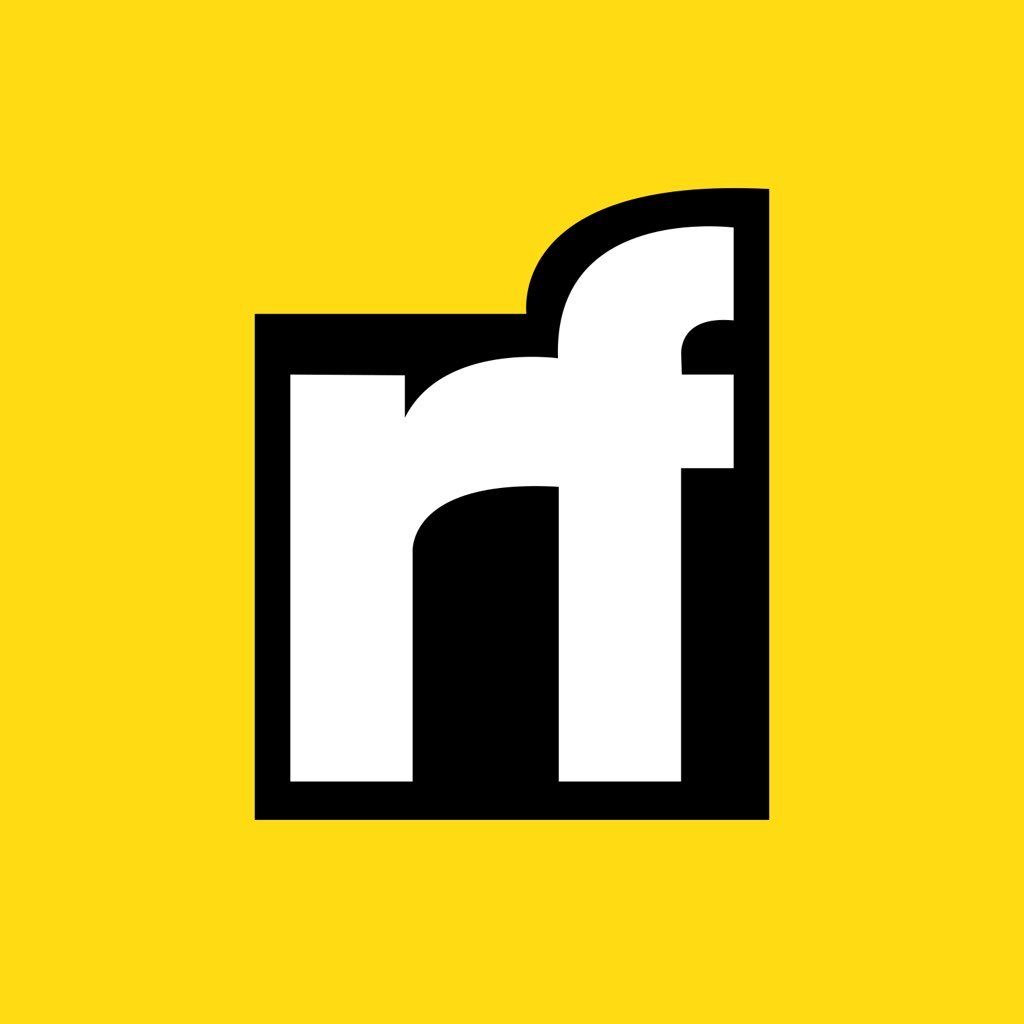
Zayn Korai | Sciencx (2021-07-15T01:25:13+00:00) Method Chaining In Golang. Retrieved from https://www.scien.cx/2021/07/15/method-chaining-in-golang/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.