This content originally appeared on DEV Community and was authored by Gobinath Varatharajan
This video by far the best piece of advice to learn react.?
Baisc React JSX
import React from 'react';
import ReactDOM from 'react-dom';
// greeting is what it will render
function greeting() {
return <div>Hello World</div>
}
// root from html is where to render
const rootNode = document.getElementById('root');
// ReactDOM.render(what, where);
ReactDOM.render(greeting(), rootNode);
Nesting Expression
- { } - it help us to interpreted the javascript expression in JSX
const year = 2020;
function Date() {
return <div>Covid-19 hit on the year {year}</div>
}
Styling JSX with Inline-CSS
- it help us to interpreted the javascript expression in JSX as an object.
const greeting = <button
style={{backgroundColor: 'red'}}>Submit
</button>
Using Multi-Component with JSX
- Function vs Class
import React from 'react';
import ReactDOM from 'react-dom';
// 1. Using Function Component
const Header = () => {
return <h1>Hello World</h1>;
}
const Footer = () => {
return <p>Welcome to React Component</p>;
}
// 2. Using Class Component
class Header extends React.Component {
render() {
return <h1>Hello World</h1>;
}
}
class Footer extends React.Component {
render() {
return <p>Welcome to React Component</p>;
}
}
function App() {
return (
<>
<Header />
<Footer />
</>);
}
const rootNode = document.getElementById('root');
ReactDOM.render(<App />, rootNode);
Argument Vs Parameter
- Parameters are declare within the function
- Arguments are the value pass to the function
// Parameter
function argumentVar(par1, pa2, par3, par4, par5) {
console.log(par1 + par2 + par3 + par4 + par5);
}
// Function call
argumentVar(1,2,3,4, 5);
// 11
// Arguments
function argumentVar(parameter1, parameter2, parameter3) {
console.log(arguments.length);
}
// Function call
argumentVar(1,2,3,4,5);
// 5
Single Props
- Help to pass value to the component
- props are object.
- They are read-only(immutable) and they cannot directly change
function Name(props) {
return <h1>Hello {props.username}</h1>
}
const rootNode = document.getElementById("root");
ReactDOM.render(
<Name username="Tony Robbie" />,
rootNode
);
// Hello Tony Robbie
Parent and child relationship with Props
- you should implement {props.children} when you're passing children in the parent component.
import React from 'react';
import ReactDOM from 'react-dom';
// This is child
function Header(props) {
return <h1>Hello {props.username}</h1>;
}
// This is the parent
function Layout() {
return
<div style={{ background: 'golden' }}>
{props.children}
</div>;
}
function App() {
return (
<Layout>
<Header username="Tony Robbie" />
</Layout>
);
}
const rootNode = document.getElementById('root');
ReactDOM.render(<App />, rootNode);
Map
- Help toaccess array of element
function App() {
const actor = ["bruce", "robert", "will"];
return (
<ul>
{actor.map(character => (
<li>{character}</li>
))}
</ul>);
}
//you can also do this with component
function App() {
const actor = ["bruce", "robert", "will"];
return (
<ul>
{actor.map(character => (
<Character character={character} />
))}
</ul>);
}
function Character(props) {
return <li>{props.character}</li>
}
//render
const rootNode = document.getElementById("root");
ReactDOM.render(<App />, rootNode);
key
- Unique identifier to do some change only the needed once.
function App() {
const actor = ["bruce", "robert", "will"];
// here I have use indexes
// you can also use some package for it.
return (
<ul>
{actor.map((character, i) => (
<Character key={i} character={character} />
))}
</ul>);
}
Event Handler
- onClick eventhandler
// you have access to function data with event
function Character(props) {
function handleCharacterClick(event) {
alert(props.character);
console.log(event);
}
return <li onClick={handleCharacterClick}>{props.character}</li>
}
- onChange eventhandler
function handleInputChange(event) {
const inputValue = event.target.value;
console.log(event);
return (
<input onChange={handleInputChange} />
)
}
In Part 2 - I wil post all about Hooks all the post written in my blog are from my knowledge feel free to oppose it?.
This content originally appeared on DEV Community and was authored by Gobinath Varatharajan
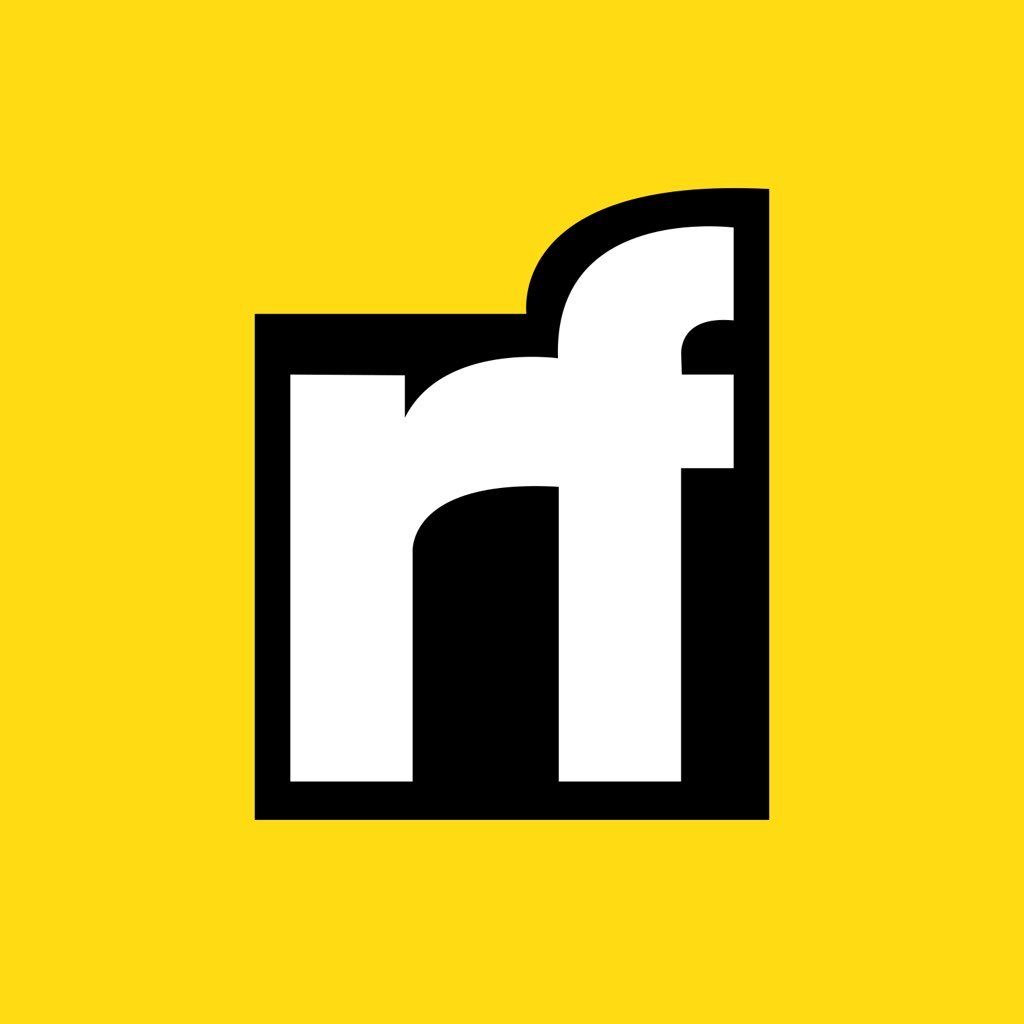
Gobinath Varatharajan | Sciencx (2021-07-26T17:33:31+00:00) Essential React Concept – Part 1. Retrieved from https://www.scien.cx/2021/07/26/essential-react-concept-part-1/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.