This content originally appeared on DEV Community and was authored by Hiep Le
Click ⭐ if you like the project. Pull Requests are highly appreciated ❤️
Github link: https://github.com/hieptl/netflix-clone/tree/main/advanced/netflix-react-hook-form
I'm Hiep. I work as a full-time software engineer. Most of my open-source projects are focused on one thing - to help people learn ?.
The repository helps you learn react hook form by building Netflix. It means that you are learning react hook form by building a real-life project. I will explain concepts in details. This post is the eighth part in my series and it is suitable for beginners.
If you feel the repository is useful, please help me share the post and give me a Github ⭐. It will make me feel motivation to work even harder. I will try to make many open sources and share to the community.
Preface
This course will help you to learn react hook form by building Netflix. It means that you are learning by doing a real-life project.
Table of Contents
No. | Topics |
---|---|
0 | How to Run the Project. |
1 | Live Demo. |
2 | Introduction about the Creator. |
2.1 | Greenwich University. |
2.2 | Hitachi Vantara Vietnam. |
3 | Prequisites. |
3.1 | Softwares. |
3.2 | Technical Skills. |
3.3 | Materials. |
4 | Purposes of the Course. |
4.1 | Final Project. |
4.2 | Job. |
5 | React Hook Form. |
6 | Apply React Hook Form to Netflix. |
7 | Conclusion. |
8 | References. |
Table of Images.
No. | Topics |
---|---|
1 | Figure 1: Apply React Hook Form to Netflix. |
0. How to Run the Project.
Step 1: Clone the project by using git clone or download the zip file.
Step 2: Open "terminal" / "cmd" / "gitbash" and change directory to "netflix-clone" and run "npm install" to install dependencies.
Step 3: Run "npm start" to run the fron-end project.
1. Live Demo.
- https://i3fgt.csb.app/login
- Username: demo@gmail.com
- Password: 123456
The login function was implemented by using React Hook Form. You can try to use the account above in order to test the feature. The result will be shown on the console log.
2. Introduction about the Creator.
2.1. Greenwich University.
Valedictorian.
GPA 4.0 / 4.0.
Machine Learning paper - Recommendation System - IEEE/ICACT2020.
Co-Founder / Mentor IT club.
2.2. Hitachi Vantara Vietnam.
Employee of the year.
Second prize - innovation contest.
Techlead - HN branch.
One of CoE Leaders (Center of Excellence).
3. Prequisites.
3.1. Softwares.
Install NodeJS.
An IDE or a text editor (VSCode, Intellij, Webstorm, etc).
3.2. Technical Skills.
Basic programming skill.
Basic HTML, CSS, JS skills.
Basic React skill. (If you want to learn about React, you can refer Learn React by Building Netflix: https://dev.to/hieptl/learn-react-by-building-netflix-1127).
3.3. Materials.
Html, css, js (source code) was prepared because I want to focus on React and share knowledge about React. Building html and css from scratch would take a lot of time.
README.md (the md file will contain everything about the course).
Netflix data will be used to import to Firebase. In this course, we use Firebase as our back-end service.
4. Purposes of the Course.
4.1. Final Project.
The course would help you have understanding about React and React Hook Form.
You could build the final project with end-to-end solution (front-end solution using React and back-end solution using Firebase).
4.2. Job.
- After finishing the course, you could get a job with fresher / junior position.
5. React Hook Form.
According to the documentation, performant, flexible and extensible forms with easy-to-use validation.
Reducing the amount of code you need to write, and removing unnecessary re-renders are some of the primary goals of React Hook Form
6. Apply React Hook Form to Netflix.
Figure 1. Apply React Hook Form to Netflix.
It is time to understand React Hook Form by applying it to our Netflix application. React hook form will be applied to validate the user's credentials and help us to get the input values.
Step 1: Install react-hook-form by running the statement npm install react-hook-form.
Step 2: Import useForm hook from react-hook-form in LoginForm component.
// import react hook form.
import { useForm } from "react-hook-form";
- Step 3: useForm provides many methods such as register, trigger, getValues.
...
const { register, trigger, getValues } = useForm();
...
1st NOTE:
register: is used to register input elements. We need to register the input elements and then we can perform actions on them such as validating or get values from them.
trigger: is used to validate input elements.
getValues: is used to get values from the input elements.
- Step 4: Update login function with the following code.
/**
* handle event when the user clicks on "Login" button.
*/
const login = async () => {
try {
// validate user's information.
const isUserCredentialsValid = await trigger();
if (isUserCredentialsValid) {
// get user's information.
const { email, password } = getValues();
// call firebase authentication service.
const userCredentials = await firebaseAuth.signInWithEmailAndPassword(
email,
password
);
console.log(userCredentials);
if (userCredentials) {
console.log(userCredentials);
}
}
} catch (error) {
console.log(JSON.stringify(error));
}
};
2nd NOTE:
As mentioned before, trigger will be used to validate the user's credentils. This function return true / false and we assign the returned value to isUserCrendentialsValid variable.
If the user's information is valid. we will get the input email and password from getValues and then use them to call Firebase authentication service.
Conclusion
In this course, we have learn about react hook form by building Netflix. I hope that you can apply react hook form to your projects. If you feel the project is useful, please help me share it to the community and give me Github ⭐
References
[1]. https://react-hook-form.com/
This content originally appeared on DEV Community and was authored by Hiep Le
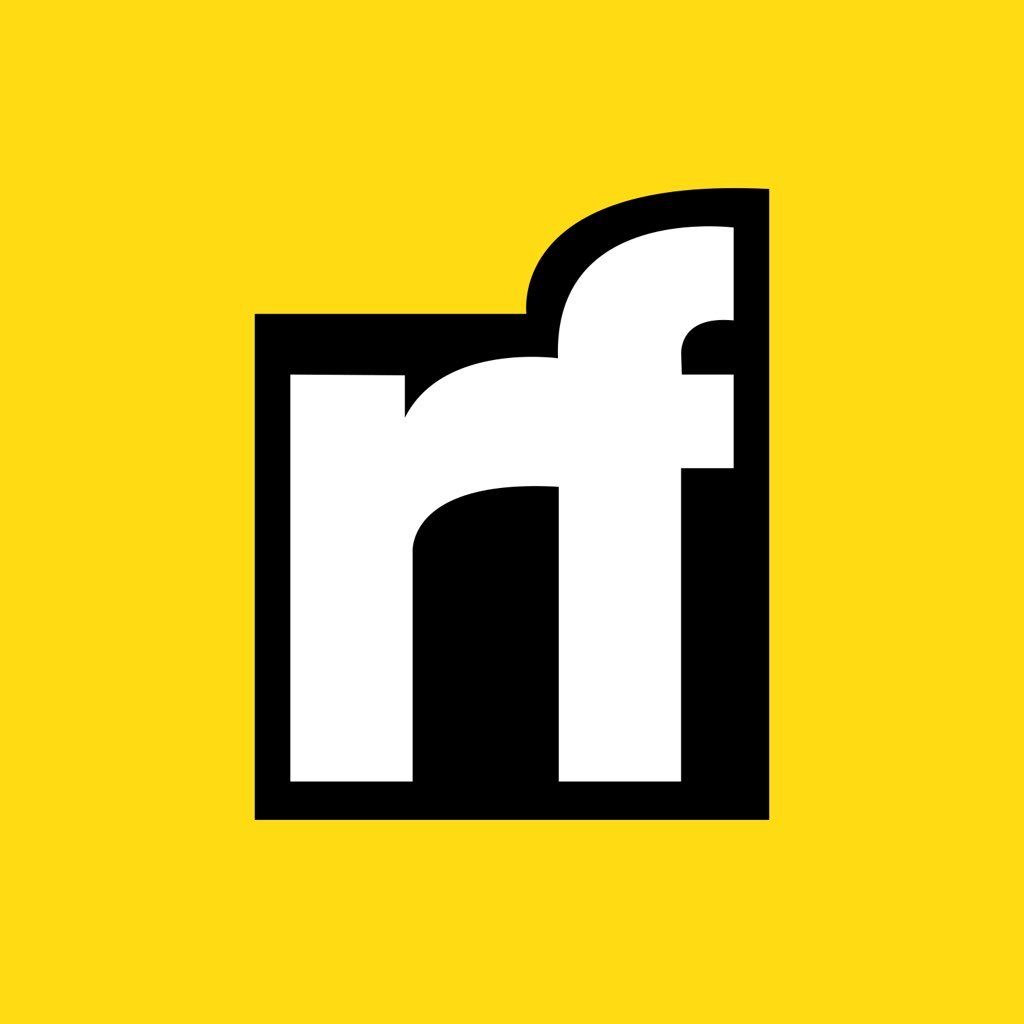
Hiep Le | Sciencx (2021-07-28T14:19:48+00:00) Learn React & React Hook Form By Building Netflix. Retrieved from https://www.scien.cx/2021/07/28/learn-react-react-hook-form-by-building-netflix/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.