This content originally appeared on DEV Community and was authored by Menard Maranan
Converting a static page or site into React App is actually simpler than what some might think. The gist is that you'll only restructure and format things out. Just plug the data to it, or with an API to make it Dynamic or feel more like an App.
In this quick blog, I'll be going through the simple steps on how to convert a static page into a React App.
Prerequisite
This assumes you already have React installed into your machine, and of course, you know the gist of the library.
So before we start the conversion, let's do a simple create-react-app to initialize our project. I'll be using npx
here:
npx create-react-app My_App
# wait for it to finish...
cd My_App # or code My_App if you have VS code
Remove the unnecessary files and the boilerplate code. Now we're ready to go.
Conversion
Turn page/s into Parent Components
If you only have one page, you can create a folder called components
under the src
folder. Then, create a single .jsx
file there like index.jsx
. Create a React Component within index.jsx
, then copy and paste the body of your static HTML file to the return statement of that Component.
And if you have multiple pages, I recommend creating a separate pages
folder under src
folder and create .js
file for each (HTML) page of your static site. Again, create React components for each file (or page) and copy-paste the body of the HTML files into the react components.
Fix Syntax
The next thing we'll be doing is correct the syntax of our plain HTML code into JSX. More specifically, change the following:
- change
class
toclassName
- change
style
props from string to objects, and change theBBQ-casing
of CSS props tocamelCase
(i.e.style="text-align: center;"
tostyle={textAlign: 'center'}
). - End the self-closing tags (i.e.
<img>
to<img />
, or<br>
to<br />
.
Add CSS
Now, it's time to add your CSS to the components. Create a styles
folder under src
folder, and drop there all your CSS
files. Then, import the corresponding CSS for each pages (if applicable).
Dependencies
Installing the dependencies of your page (i.e. Bootstrap, Font-Awesome, etc.) that was recently delivered via CDN is recommended to be installed via npm
or yarn
(if there's any). Try to find the corresponding React module for your component and install them within your React App:
# installing bootstrap for react via npm/yarn instead of cdn
npm i react-bootstrap
# or
yarn add react-bootstrap
Then, import them to their corresponding components/pages.
Decompose Page/s
This is the part where we truly take advantage of React, which is by breaking down each page into smaller, reusable components.
For this step, create a components
folder if you haven't yet. If your website is written with Semantics in mind, then the conversion would be a breeze.
Understand the structure of your pages. Think about what sections makes up a page, what smaller components or sub components builds up which area of the page.
An example would be:
<main>
<h1>Welcome to Homepage!</h1>
<article>
<header>
<h1>Headline 1</h1>
<p>
Lorem Ipsum dolor...
</p>
</header>
<section>
<p>
Lorem ipsum dolor sit amet...
</p>
</section>
<section>
<p>
Lorem ipsum dolor sit amet...
</p>
</section>
<section>
<p>
Lorem ipsum dolor sit amet...
</p>
</section>
...
</article>
<article>
<header>
...
</main>
From there, we can clearly see the repeated pattern of:
<section>
<p>
Lorem ipsum dolor sit amet...
</p>
</section>
We can turn that out into a component and prevent ourselves from repeatedly writing them:
// src/components/Section.jsx
export default function Section({ data }) {
return (
<section>
<p>
{data}
</p>
</section>
)
}
That's one, but we can further group this page into hierarchy. Let's get through each of those:
First off, notice that we also have the header? That's also another component:
// src/components/Header.jsx
export default function Header({ headline, description }) {
return (
<header>
<h1>{headline}</h1>
<p>
{description}
</p>
</header>
)
}
And now, by looking on our HTML hierarchy, the parent component where we will put the Header and and Section components will be the Article
, so let's import those components we just created and nest them inside the Article component:
// src/components/Article.jsx
// assuming that we have a data source
// for the contents of the page
import Header from './Header'
import Section from './Section'
export default function Article(props) {
const { headline, description, sections } = props;
return (
<article>
<Header
headline={headline}
description={description}
/>
{
sections.map(section => (
<Section data={section} />)
)
}
</article>
)
}
jsx
Now we're cooking!
Alright, for the last restructuring, noticed that on our static HTML, there are more Article
tags with similar structure that follows? Guess what, we can also the same thing here:
// src/pages/Main.js
import Article from '../components/Article.jsx'
export default function Main() {
const someData = ...
return (
<main>
<h1>Welcome to Homepage!</h1>
{
someData.map(data => {
const { headline, description, sections } = data
return (
<Article
headline={headline}
description={description}
sections={sections}
/>
)
})
}
</main>
)
}
But wait, have you noticed a common problem that occurs on React App? If you're an Eagle-eyed reader, then yes, we committed prop-drilling
here. We can use Redux or just simply the Context API, but that's another topic for another blog, so bear with me for now.
Ok, just take note that the Main
component we just created is saved under the pages
folder as it's actually representing an entire page already in itself.
Routing
This step is optional (only if you have multiple pages), and we can leverage React Router
so that we don't need to refresh the page.
You can install react-router-dom
via npm
or yarn
and start routing from App.js
which is in the top level of inside the src
folder.
Still confused?
If things are still confusing to you, then please don't because you don't have to. Instead, let me help you with that and I'll just do it for you. Yah, that's right, let me handle it for ya'. I offer a service in Fiverr and I offer my service FREE for the first 3 orders, and after that, it's $10 dollars. I will convert your static page into a React App completely FREE OF CHARGE for the first 3 orders in exchange of an honest review. Just visit https://www.fiverr.com/menardmaranan/convert-html-and-css-to-react-components and I'll handle the conversion instead for Free.
Till' next time, see ya'!
Follow me on Twitter
This content originally appeared on DEV Community and was authored by Menard Maranan
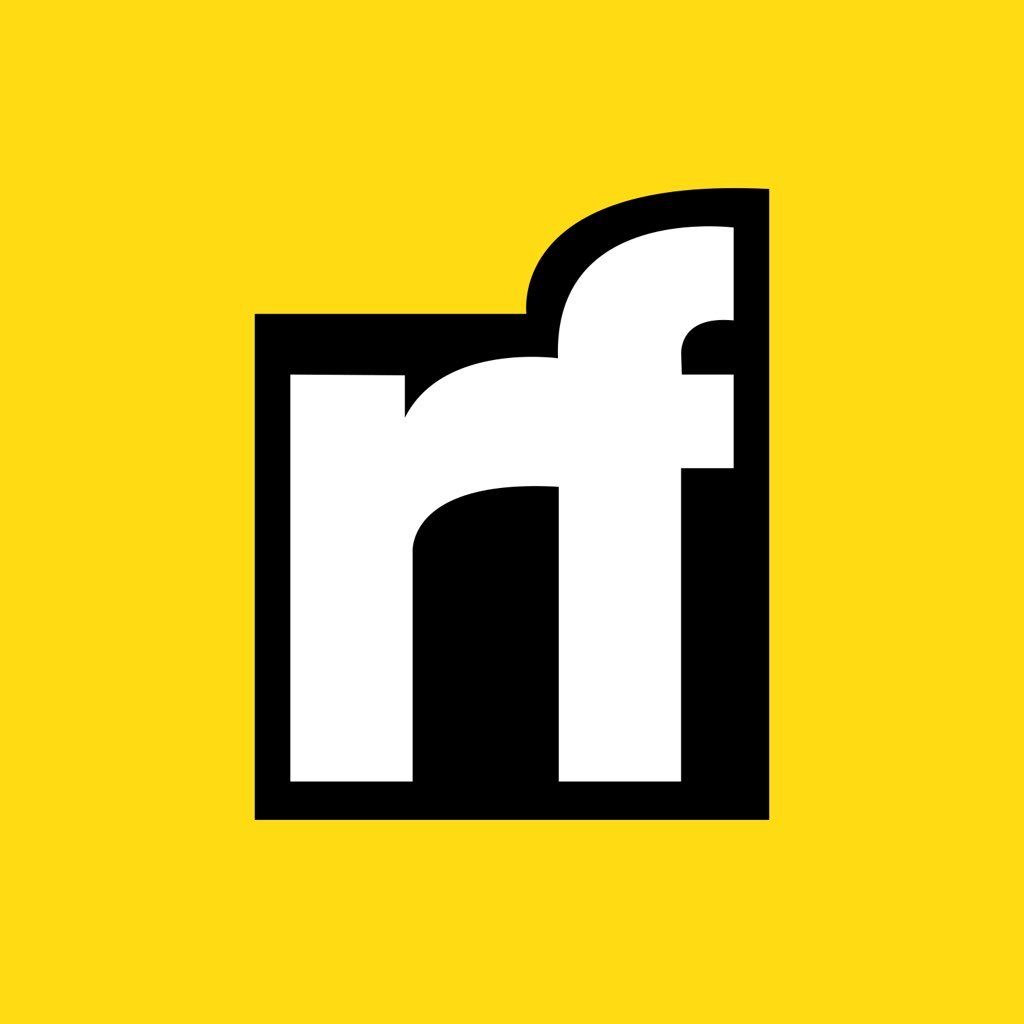
Menard Maranan | Sciencx (2021-08-11T01:11:25+00:00) Converting static HTML/CSS site to React App. Retrieved from https://www.scien.cx/2021/08/11/converting-static-html-css-site-to-react-app/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.