This content originally appeared on DEV Community and was authored by Pratham
In this blog, I will show you how you can get data of 50 users from an Random user API in your React project.
Let's get started!
Prerequisites
- Basic understanding of React and React Hooks ( Optional )
- Node Js Installed in your system
Create your React app.
Open your terminal and type...
npx create-react-app fetch-data-react
This will create a basic React app in your system with the folder name
"fetch-data-react"
Now go into your project
cd fetch-data-react
and run
npm start
You should see this in your browser
Fetching data using Axios
Open a terminal and install Axios
npm install axios
Axios is a simple promise-based HTTP client for the browser and node.js. Axios provides a simple to use the library in a small package with a very extensible interface.
By using Axios it’s easy to send asynchronous HTTP requests to REST endpoints and perform CRUD operations.
Now open App.js from src folder
Edit App.js as follows
import React, { useEffect } from "react";
import axios from "axios";
export default function App() {
const fetchUsers = () => {
return axios
.get("https://randomuser.me/api/?results=50")
.then((res) => console.log(res.data));
};
useEffect(() => {
fetchUsers();
}, []);
return (
<div>
<h1>Hello World</h1>
</div>
);
}
Let me explain the code,
-On line 2 you can see we imported axios.
-The following function fetchUsers fetches the data of 50 random users from an API by sending a GET request.
-useEffect() starts a fetch request by calling fetchUsers() async function after the initial mounting.
Now open your browser and check your console
You can see data of 50 users
You can now use this data in your React project.
Next Steps?
Now you know how to fetch data from an API using Axios.
What if I tell you you can get data of every user on GitHub using this GitHub API https://api.github.com/users. Amazing, right?
Your task if you want to practice what you learned
try creating this project (GitHub profile Card)[https://codepen.io/warmsprings27/pen/PGkJAW] using React and Axios.
Thank you for reading
IF YOU LIKED THE POST, THEN YOU CAN BUY ME MY FIRST COFFEE EVER, THANK YOU
You can dm me on Twitter if you need help related to web development
This content originally appeared on DEV Community and was authored by Pratham
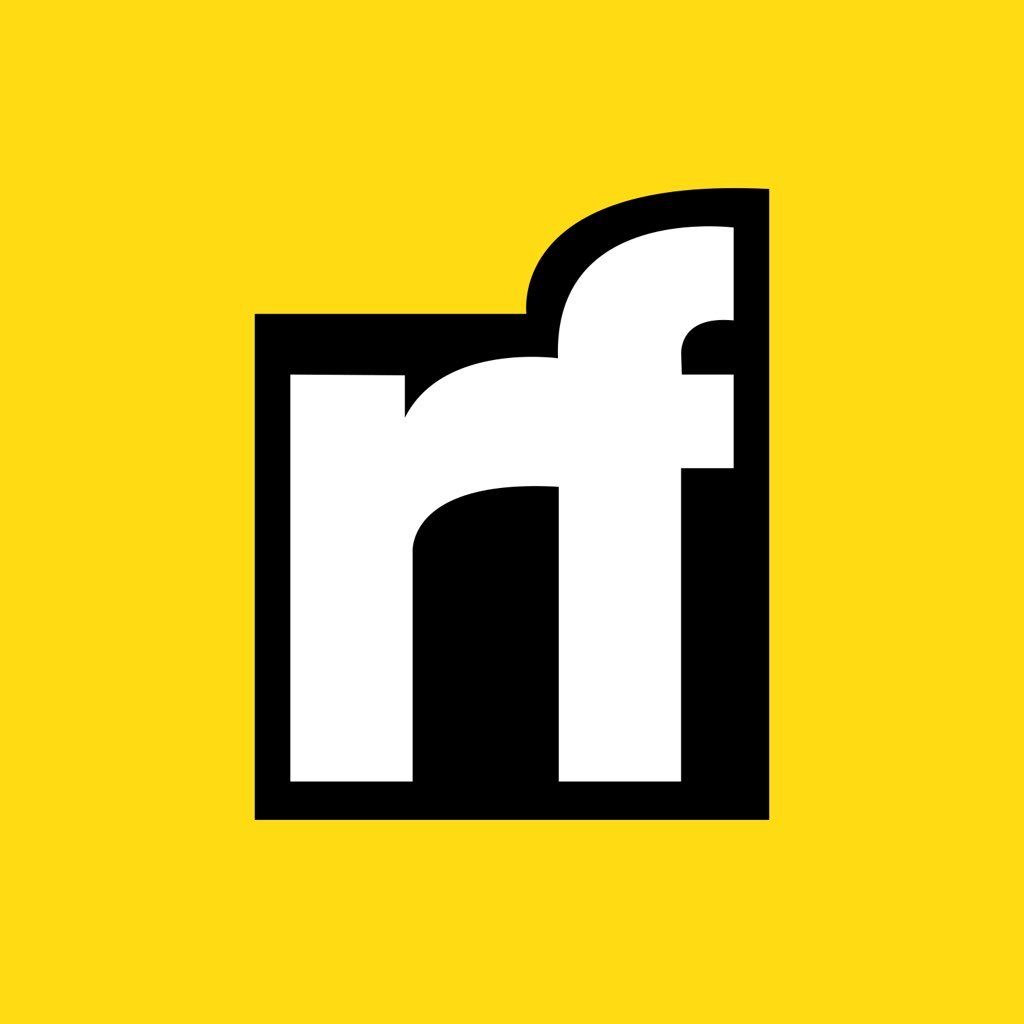
Pratham | Sciencx (2021-08-16T14:33:24+00:00) How to fetch data from an API in React Project. Retrieved from https://www.scien.cx/2021/08/16/how-to-fetch-data-from-an-api-in-react-project/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.