This content originally appeared on Bits and Pieces - Medium and was authored by Alicia Jones
Learn what IIFEs are in 6 minutes
What are IIFEs?
IIFE is the abbreviation for Immediately Invoked Function Expression. Although the term looks long and confusing, it is a quite simple concept. You can easily understand this concept by understanding what each term means.
- Immediately Invoked — runs immediately
- Function — a typical javascript function
- Expression — a javascript expression is a piece of code that simply evaluates to a value
According to MDN Docs, an IIFE is a JavaScript function that runs as soon as it is defined. This would make perfect sense if you look at the three terms I’ve listed above.
Let’s have a look at an IIFE to get a better understanding.
(function () {
//statement
})();
What you see above is a typical IIFE. This syntax contains two major parts.
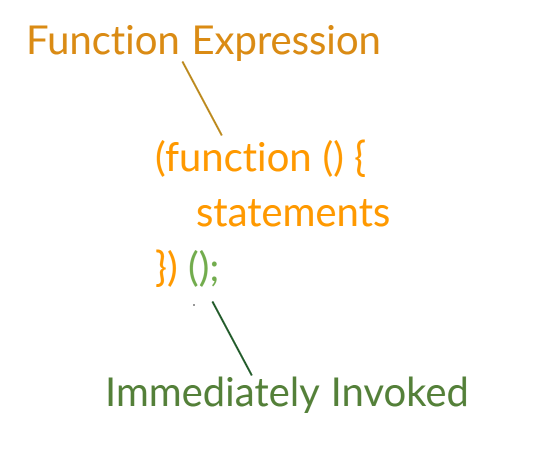
Function Expression
The component in orange is the function expression which is simply an anonymous function wrapped within the Grouping Operator().
Immediately Invoked
The component in green creates the immediate invocation of the function expression by using the invocation operator which is simply (). In other words, this basically calls the function expression just like how you would call any other function.
In the above code snippet, we call the getName function by using the invocation operator.
Why use the grouping operator?
Although functions are expressions, you will run into problems when you directly invoke a function at the time of declaration. The reason for this behavior is that certain expressions in JavaScript can behave as a statement under certain contexts. Functions also behave in a similar manner. A function definition would be an expression when it is on the right-hand side of an assignment, but a statement when it is at the start of a file.
To simply resolve this behavior, we get the help of the grouping operator which would force JavaScript to treat the function definition within the () as an expression.
IIFEs are also referred to as Self Executing Anonymous Functions and Self Invoked Anonymous Functions.
Tip: Build & share independent JS components with Bit
Bit is an extensible tool that lets you create truly modular applications with independently authored, versioned, and maintained components.
Use it to build modular apps & design systems, author and deliver micro frontends & microservices, or simply share components between applications
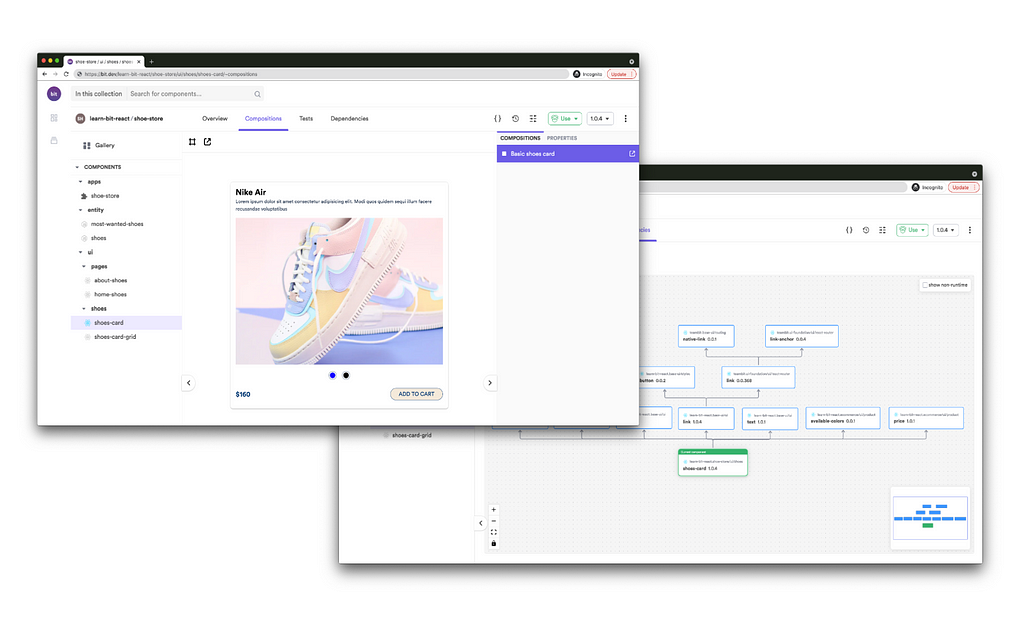
Bit: The platform for the modular web
Syntax Variations
Although the above-discussed syntax is the most commonly used, there are still more possible variations that can be found.
These variations replace the grouping operator with the unary operators +,-,~ and ! .
Arrow Function in IIFEs
With the introduction of arrow functions in ES6, another variation in IIFE syntax was possible. This variation used the arrow function instead of an anonymous traditional function. The arrow function was wrapped around with the grouping operator. But you must note that you would get a syntax error if you try to replace the grouping operator with a unary operator in this scenario. The arrow function variation of the IIFE can only be used with a grouping operator.
Async and Named in IIFEs
You can replace ordinary functions with async functions in IIFEs. You can also use named functions in IIFEs. But you will not be able to call the function again after initial invocation.
The unary operators can be used to replace the grouping operator similar to previous examples. But as noted earlier, arrow functions must be coupled with the grouping operator.
Use Cases
There are several use cases for IIFEs and Marius has done a great job in compiling a list of them.
Avoiding Global Scope Pollution
One of the well-known uses of IIFEs is avoiding global scope pollution. Local variables declared using var keyword are scoped to the closest containing function. And if there is no function that exists, the variables will be global and would pollute the global scope. To avoid this, we simply wrap the var variables in an IIFE such that they are scoped within and isolated from the outside world.
To avoid this pollution, simply wrap them up in an IIFE.
But after the introduction of let and const keywords, this use case lost its popularity as the newer keywords allowed an easier implementation to scope local variables to the nearest block. But when you are working with older browsers that do not support the newer keywords, you might have to get back with IIFEs.
Closures and Private Data
IIFEs enable you to create closures that can maintain private data. This can be done by wrapping a local variable and have it accessed by a function that will be returned by the IIFE. This implementation provides you with a closure that enables the function to access the local variable even when that function is executed outside of the IIFE’s lexical scope. Below is an example given by Marius.
If you observe the above snippet, you will notice that the count variable cannot be accessed or modified from outside the scope making it truly private. The only way to access to the variable would be through the function being returned by the IIFE.
You can further expand this example by returning several functions wrapped within an object that can be called from the outside. This implementation heavily influenced the revealing module pattern.
Renamed Variables (Aliasing)
You might face situations where two or more libraries use the same global variable names. This naming conflict would cause issues as shown in the first use case. To resolve this naming conflict without much effort, you should wrap the piece of code with an IIFE that passes one of the global variables as an argument. Within the IIFE, you can access the value by any parameter you wish — even the previously used name which caused the naming conflict. This is because of IIFE having its own local scope.
A classic example of this use case would be where use jQuery and another library which also has assigned the $ symbol to its global variable. You will have to use IIFE to get out of this issue.
jQuery provides a native way of resolving similar naming conflicts. You can read more about it here. This does not rule out this use case as there are other libraries that might not provide a native implementation of handling naming conflicts.
Global Object Capture
Another IIFE use case mentioned by Marius is global object capture. If you have been working with JavaScript across the platform, you would be aware that the global object will be different on each platform. On the web, you will be able to use window, self, or frames but in web workers, only self will work. None of these would work on Node and you must use global. Below is an implementation by Todd that helps you write universal code.
(function(global) {
// ...
})(this);
Note: There are several issues with this implementation. The this keyword will be undefined in modules and functions running in strict mode.
To provide a standard way of accessing the global regardless of the environment, ES2020 introduced globalThis. This property is supported in most browsers and the new standard way of accessing the global object.
Enable the Use of Await Keyword Outside of Async Functions
This is one of my favorite use case of IIFEs. If you use the await keyword outside of an async function, you will receive a Syntax Error. As a workaround, developers have been using async IIFEs for quite some time. This implementation was widely prevalent in Node js applications. One let down of async IIFEs is that you are responsible for handling the errors within. Let’s have a look at an example below.
(async()=>{
await Promise.resolve("resolved");
})();
//Uncaught errors
(async()=>{
await Promise.reject("rejected");
})();
//Catching errors
//Method 1
(async () => {
try {
await Promise.reject("rejected");
} catch (err) {
console.log(err)
}
})();
//Method 2
(async () => {
await Promise.reject("rejected");
})().catch(err => {
console.log(err);
});
The top-level await proposal would remove the need for async IIFEs coupled with await keywords. You can read more about the proposal here.
Why Should You Use Top-level Await in JavaScript?
IIFEs are an awesome piece of functionality that still has its advantages even in the modern era of JavaScript.
Thank you for reading & happy coding!
Learn More
- 4 New Exciting Features Coming in ECMAScript 2021
- JavaScript Finally Has Support for Native Private Fields and Methods
- Build Scalable React Apps by Sharing UIs and Hooks
Resources
MDN Docs
A drip of JavaScript
Marius Schulz’s blog
Mastering JS
Stackabuse
Understanding JavaScript IIFEs Like a Boss was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Alicia Jones
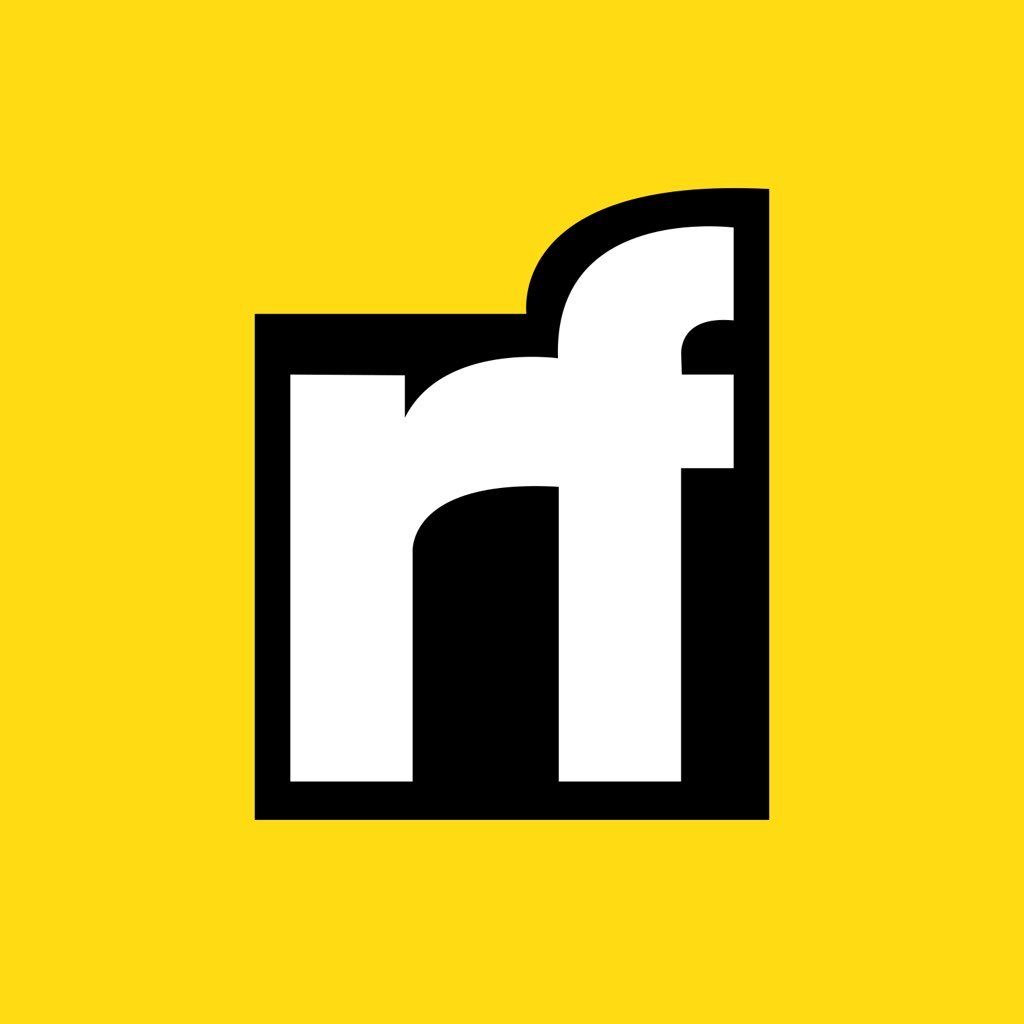
Alicia Jones | Sciencx (2021-08-17T14:11:14+00:00) Understanding JavaScript IIFEs Like a Boss. Retrieved from https://www.scien.cx/2021/08/17/understanding-javascript-iifes-like-a-boss/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.