This content originally appeared on DEV Community and was authored by Aya Bouchiha
What's POST request
POST: is a request that is used for sending information or data to a specific server.
POST request using then and catch
const postTodo = (todo) => {
fetch('https://jsonplaceholder.typicode.com/posts',{
method:'POST',
body:JSON.stringify(todo),
headers:{
'header-name':'header-value'
}
}).then(response => response.json())
.then(data => console.log(data) /* {id:101} */)
.catch(e => console.log('something went wrong :(', e))
}
const data = {
title: 'buy food',
body: "buy healthy food",
userId: 8,
};
postTodo();
POST request using async and await
const postTodo = async (todo) => {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts',{
method:'POST',
headers:{
'header-name': 'header-value'
},
body:JSON.stringify(todo)
})
const data = await response.json();
console.log(data); // {id:101}
}catch(e){
console.log('something went wrong :(', e)
}
}
const data = {
title: 'buy food',
body: "buy healthy food",
userId: 8,
};
postTodo(data);
Suggested Posts
- Sending GET Request Using Fetch
- Making GET And POST Request Using Axios In React.js
- Making PUT & DELETE Request Using Axios In React.js
- Youtube Courses, Projects To Master Javascript
- Your Essential Guide To Map Built-in Object In Javascript
- All JS String Methods In One Post!
To Contact Me:
- email: developer.aya.b@gmail.com
- telegram: Aya Bouchiha
Happy codding!
This content originally appeared on DEV Community and was authored by Aya Bouchiha
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
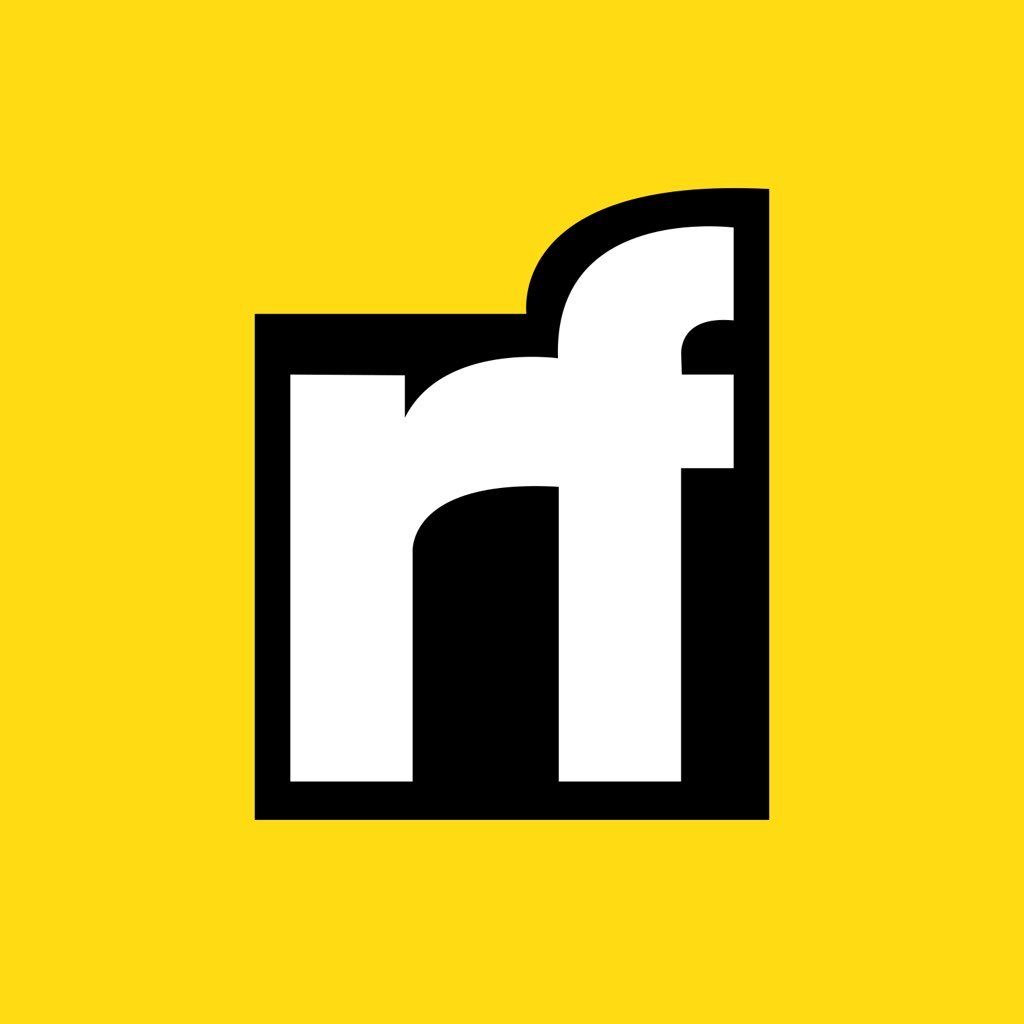
APA
MLA
Aya Bouchiha | Sciencx (2021-08-18T23:44:14+00:00) Sending POST Request In JS Using fetch. Retrieved from https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/
" » Sending POST Request In JS Using fetch." Aya Bouchiha | Sciencx - Wednesday August 18, 2021, https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/
HARVARDAya Bouchiha | Sciencx Wednesday August 18, 2021 » Sending POST Request In JS Using fetch., viewed ,<https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/>
VANCOUVERAya Bouchiha | Sciencx - » Sending POST Request In JS Using fetch. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/
CHICAGO" » Sending POST Request In JS Using fetch." Aya Bouchiha | Sciencx - Accessed . https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/
IEEE" » Sending POST Request In JS Using fetch." Aya Bouchiha | Sciencx [Online]. Available: https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/. [Accessed: ]
rf:citation » Sending POST Request In JS Using fetch | Aya Bouchiha | Sciencx | https://www.scien.cx/2021/08/18/sending-post-request-in-js-using-fetch/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.