This content originally appeared on DEV Community and was authored by Fikri Mulyana Setiawan
Hi, Do you know that about 1 month ago (to be exact 20 days ago) I made a game? What? You haven't tried it yet? Try playing the game first here.
Interesting, right? Actually, the code of this game is quite difficult for me to explain here. Therefore, I will not discuss the code, but only the algorithm.
The Algorithm
Coordinate System
For this game, I used the Cartesian coordinate system that we often learn in school. However, to save variable usage in javascript, I use array (vector) format to store coordinate data. I stored the coordinate data for spaceship in an array called poss
and coordinate data for UFO in an array called posu
.
Controller
First of all, I created 4 buttons for 4 different movements, left, right, up, and down. This button is useful for moving the spaceship to the left, right, up, and down. To set the position of the spaceship, we can use CSS, with the code:
.spaceship {
position: absolute;
left: __px;
top: __px;
}
With javascript, we can change the value of top
and left
. I used spaceship.style.left
to set the x-coordinate, and spaceship.style.top
to set the y-coordinate, and set the speed with setInterval
. Okay, I think this is pretty easy. We're done with the spaceship controller.
Shoot
In this game, i use the image from flaticon.com as the bullet.
If we shoot a bullet, logically we know that the initial coordinates of the bullet are the same as the initial coordinates of our body (spaceship). Therefore, when the shot
button is pressed, the first command that must be executed by this game is to find out, what are the coordinates of the spaceship. After the spaceship coordinates are known, then set these coordinates as the initial coordinates of the bullet, then fire it with bullet.style.top
.
Collision
If the spaceship collides with UFO, then the player's life (spaceship) will be reduced by 25%. Well, as we learned in school (mathematical geometry), 2 objects collide if the coordinates of each object are the same. So if the first object and the second object collide, then it should be x1=x2 and y1=y2 (x1=position x object 1). This is easy. However, there is a slight problem here. This rule applies if the colliding object is a point object (you've studied physics, haven't you?), while our object (spaceship and UFO) is a rigid body. Therefore, we need a little modification. In CSS, we know the concept of CSS box model. Simply put, this concept explains that each element in html is "wrapped" in a box. With this concept, we can modify the previous rule.
xs=x_position of spaceship and xu=y_position of UFOBy paying attention to the graph above, we can modify the condition of the spaceship when it collides with a UFO. A spaceship collides with a UFO if xu<xs+spaceship_width and xu>xs and yu<ys and yu>ys-spaceship_height
. We have also managed to solve the issue of the spaceship collides with UFO. This concept also applies to the event of a bullet hitting a UFO.
UFO Movement
If you pay attention to the game, you will know that UFO actually move randomly. To make the UFO move, I use setInterval
again, and to make the movement random, I use Math.random
.it's easy, right?
How It Works ?
The gameplay is like this :
at first the UFO moves randomly while the spaceship (player) doesn't move. The spaceship will only move if the controller button (right, left, up, down) is pressed. The UFO moves from left to right, and if the UFO has reached the edge of the screen, the UFO will return to the left side. We call this 1 cycle. In each cycle, the UFO will fire 1 bullet at random. If the bullet hits the player, the player's life will be reduced by 25%. Otherwise, the game will continue without reducing the player's life. Players can also fire at UFO. If the bullet hits the UFO, then the player will get a score +1. Every time you reach score which is the multiple of 5, the bullet speed of the UFO will increase and also increasing your chances of losing. Simple, right?
Github Repository
If you want to see the code of this game, please jump in to the github repository below and go to javascriptproject-menu/IntergalacticWar
.
fikrinotes
/
fikrinotes.github.io
Website fikri
Or, you can also go to this link. Thank you for read this article.
This content originally appeared on DEV Community and was authored by Fikri Mulyana Setiawan
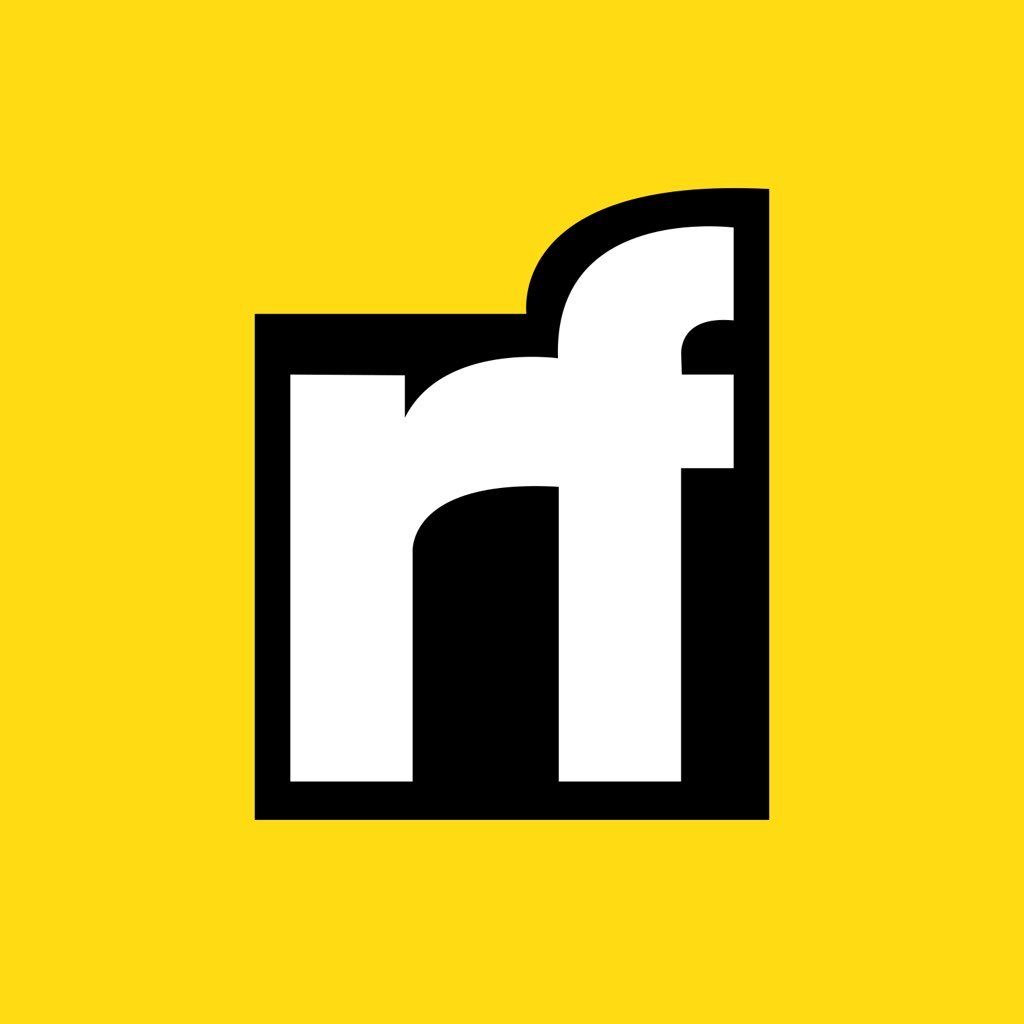
Fikri Mulyana Setiawan | Sciencx (2021-09-03T07:32:12+00:00) How I Build Intergalactic War Game In Pure JavaScript. Retrieved from https://www.scien.cx/2021/09/03/how-i-build-intergalactic-war-game-in-pure-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.