This content originally appeared on DEV Community and was authored by Gustavo Castillo
In this article we will learn how to add a version to our binaries in Go using a Makefile
file and a third party package to print our logo in ASCII code.
Having a version in our binaries is very important since with it, we could know which version of our application we are running on production, in addition our users could report errors for specific versions and thus be able to fix them.
Makefile to create the binary add version, and build time
# Get version from git hash
git_hash := $(shell git rev-parse --short HEAD || echo 'development')
# Get current date
current_time = $(shell date +"%Y-%m-%d:T%H:%M:%S")
# Add linker flags
linker_flags = '-s -X main.buildTime=${current_time} -X main.version=${git_hash}'
# Build binaries for current OS and Linux
.PHONY:
build:
@echo "Building binaries..."
go build -ldflags=${linker_flags} -o=./bin/binver ./main.go
GOOS=linux GOARCH=amd64 go build -ldflags=${linker_flags} -o=./bin/linux_amd64/binver ./main.go
After this we will only need to execute the make build
command from our terminal to create the binary with its respective version and build time.
Create the main file in Go
package main
import (
"flag"
"fmt"
"os"
"github.com/morikuni/aec"
)
var (
buildTime string
version string
)
const binverFigletStr = `
_ _
| |__ (_)_ ____ _____ _ __
| '_ \| | '_ \ \ / / _ \ '__|
| |_) | | | | \ V / __/ |
|_.__/|_|_| |_|\_/ \___|_|
`
func printASCIIArt() {
binverLogo := aec.LightGreenF.Apply(binverFigletStr)
fmt.Println(binverLogo)
}
func main() {
displayVersion := flag.Bool("version", false, "Display version and exit")
flag.Parse()
if *displayVersion {
printASCIIArt()
fmt.Printf("Version:\t%s\n", version)
fmt.Printf("Build time:\t%s\n", buildTime)
os.Exit(0)
}
}
Repository and video
If you want to see the complete code or the video where I explain how to do it step by step I leave you here the links:
- GitHub repository
- Video tutorial (In Spanish).
This content originally appeared on DEV Community and was authored by Gustavo Castillo
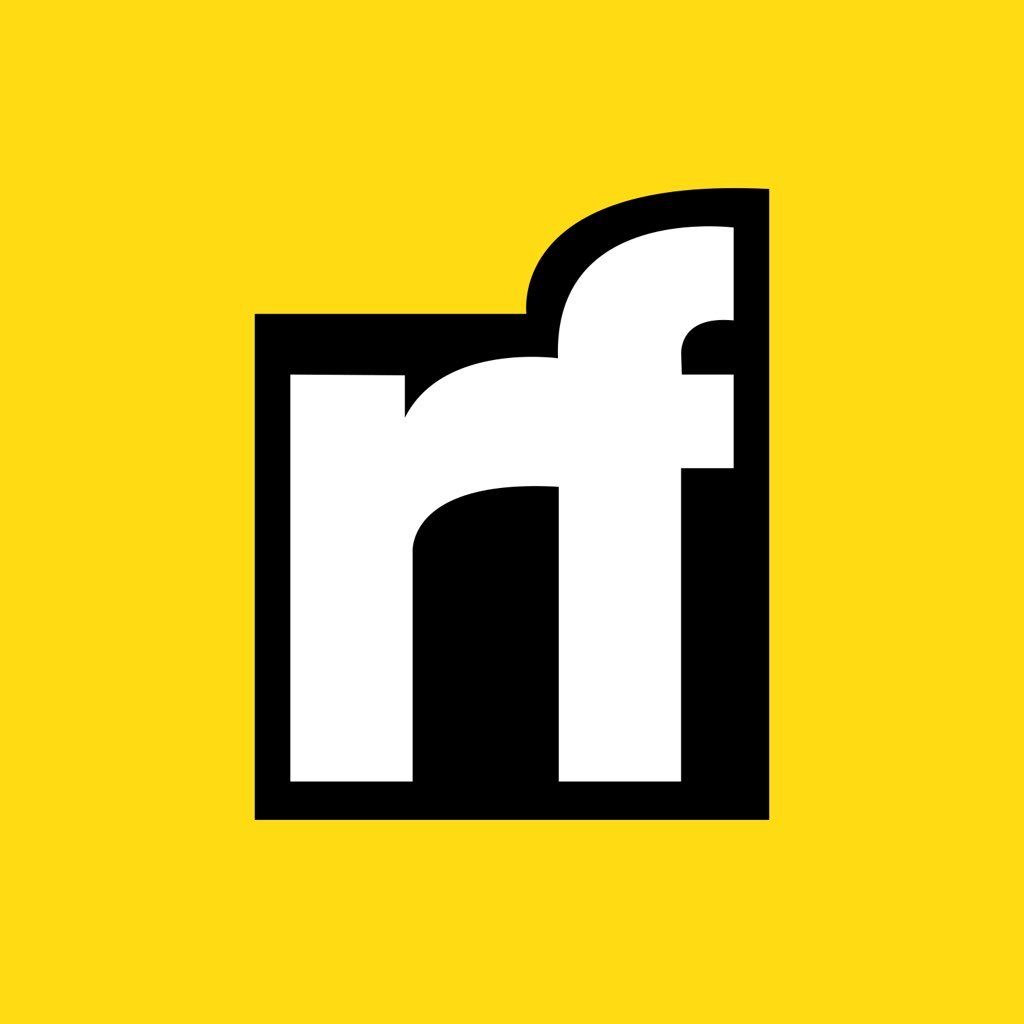
Gustavo Castillo | Sciencx (2021-09-16T22:23:28+00:00) Versioning binaries in Go. Retrieved from https://www.scien.cx/2021/09/16/versioning-binaries-in-go/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.