This content originally appeared on Level Up Coding - Medium and was authored by Tara Prasad Routray
Learn the basics about Node.js by creating a simple web application.
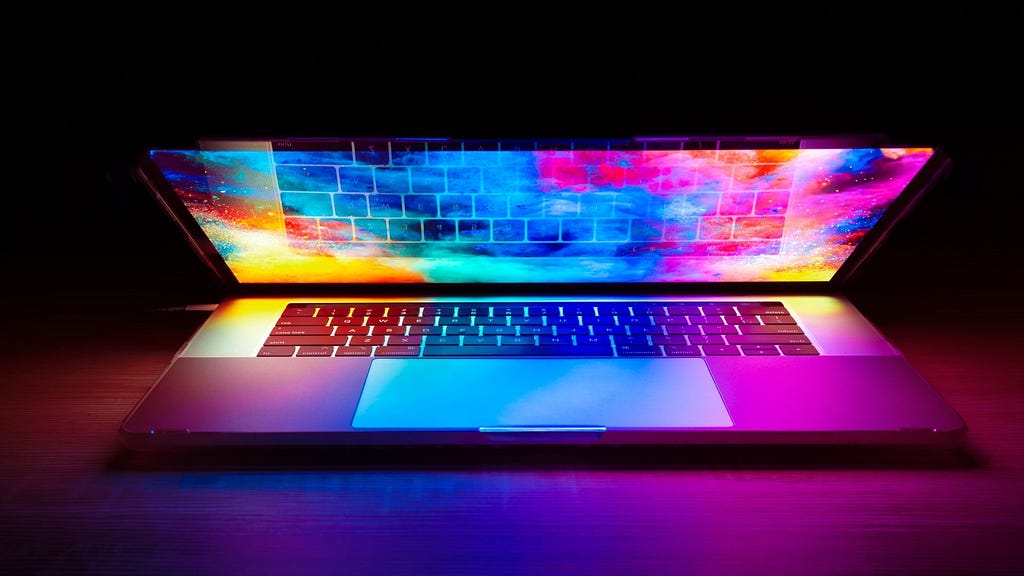
Node.js is one of the hottest server-side technologies you can learn these days. It is an open-source, cross-platform, backend JavaScript runtime environment. Ryan Dahl (the creator of Node.js) made the initial release on May 27, 2009. And now the most recent LTS (long-term support) version is 14.17.6.
Node.js has become a popular technology across the globe, especially in Silicon Valley. It is an excellent skill to have in your resume. If you’re a software developer, it will expose you to spectacular career opportunities. You can develop numerous cool applications on top of it, like social media apps, real-time video and messaging apps, online multiplayer games, real-time tracking apps and much more.
Talking about the core, Node.js runs on Google Chrome’s V8 engine. This engine compiles the JavaScript code of Node.js to machine code. Thus, allowing Node.js to be able to run on servers and to be used as a backend technology for our apps.
Here is the outlook of what you’re going to learn ahead:
- Introduction to Node.js
- Popular Companies That Use Node.js
- Install Node.js and Node Package Module (NPM)
- Create a Node Server
- Handle Request and Send Response
- Install Nodemon for Auto-restarts
- What’s next?
Introduction to Node.js
Since you’re reading this, you must be knowing JavaScript. It is a popular programming language that is used in web browsers to manipulate the DOM (Document Object Model) of websites. It can also be in several ways, like display a confirmation message, notification, modal, or implement any visual animations and transitions to an element. All these features belong to the client-side or frontend.
However, JavaScript does not stop being used on the client-side only. It can also be used on the servers, and Node.js makes this possible through the V8 engine.
Node.js is superfast. Because it achieves low latency and high throughput by using a “non-blocking” approach to serve requests. It doesn’t waste time and system resources by waiting for I/O requests. It is asynchronous and single-threaded. This means that all I/O operations don’t block any other operations. Because of that you can send emails, read files, run queries on the database, and do a lot of stuff all at the same time.
Popular Companies That Use Node.js
Node.js is new when compared to other programming languages. But there is a piece of good news. Fortune 500 companies favour Node.js over any other frameworks, and for many good reasons. They all came to the conclusion that Node.js was worth it.
The list of companies is big, so naming a few of them:
- LinkedIn (https://www.linkedin.com)
- Netflix (https://www.netflix.com)
- Uber (https://www.uber.com)
- Trello (https://trello.com)
- PayPal (https://www.paypal.com)
- NASA (https://www.nasa.gov)
- eBay (https://www.ebay.com)
- Medium (https://medium.com)
- Groupon (https://www.groupon.com)
- Walmart (https://www.walmart.com)
- Mozilla (https://www.mozilla.org)
- GoDaddy (https://www.godaddy.com)
- Yandex (https://yandex.com)
- Upwork (https://www.upwork.com)
- Yahoo (https://yahoo.com)
I know, you must be excited reading this. So, without any delay, let’s start by installing Node.js and NPM.
Install Node.js and Node Package Module (NPM)
Before we start writing actual code, we first need to install Node.js and NPM on our workstation (computer). It is recommended to download the LTS (Long-term Support) version, as it is more stable than the latest version. Click on the following link to go to the download page.
Note: NPM comes with the Node.js installer. Thus, there is no need to install that separately.
Or, you can click on the appropriate link below according to the operating system you’re using.
Windows
https://nodejs.org/dist/v14.17.6/node-v14.17.6-x64.msi
Linux
https://nodejs.org/dist/v14.17.6/node-v14.17.6.tar.gz
Mac
https://nodejs.org/dist/v14.17.6/node-v14.17.6.pkg
Once it is installed, we can start using it. The fastest way to verify whether Node.js has been installed successfully, and is ready for use, is by using your default system terminal on Mac/Linux or the command prompt on Windows. Run the following command to see the version of Node.js you have downloaded and installed:
node -v
If the above command executes successfully, we know that we are ready to start working with it. You can also verify the NPM installation by running the following command:
npm -v
What’s next? Let’s start creating a node server and set up a basic app.
Create a Node Server
Let’s create a new folder in our file system with the name “node-starter-app”. You can change the name as per your choice. Then navigate into the folder and create a new file with the name “app.js”.
This “app.js” file will act as the root file for our Node.js application. Now, in this file, we will set up a server. Before doing that, let me tell you that there are a couple of modules Node.js ships with. Naming a few:
http
The HTTP module can create a server that listens to server ports and gives a response back to the client.
https
The HTTPS module can create a server and provide a way to transfer data over HTTP SSL/TLS protocol, which is the secure HTTP protocol.
fs
The FS module or File System module allows you to work with the file system on your computer. Common uses are:
- Read files
- Create files
- Update files
- Delete files
- Rename files
path
The Path module provides a way of working with directories and file paths. Some methods are:
- basename()
Returns the last part of a path. - dirname()
Returns the directories of a path. - extname()
Returns the file extension of a path. - isAbsolute()
Returns true if a path is an absolute path, otherwise false. - format()
Formats a path object into a path string.
os
The OS module provides information about the computer’s operating system. Some methods are:
- arch()
Returns the operating system’s CPU architecture. - cpus()
Returns an array containing information about the computer’s CPUs. - freemem()
Returns the number of free memory of the system. - hostname()
Returns the hostname of the operating system. - networkInterfaces()
Returns the network interfaces that have a network address.
Now, we will be using the methods from the HTTP module which ships with Node.js to create a server. The module is not available globally by default. We need to import it to use it and the methods it provides. Refer to the following code snippet to completely set up your node server:
If you’ve created the above file inside the folder “node-starter-app”, then navigate to the folder and fire up your terminal or command prompt. And execute the following command:
node app.js
What this will do? Well, it will start the node server on the PORT you have mentioned. And, in this example, it is PORT 3000. Now go ahead and visit this URL on your web browser:
http://localhost:3000
For now, you will not see any output on the screen, as we have just used console.log(). Thus, the output is only visible on the terminal or the command prompt. Now, stop the server by quitting that terminal. In the next section, you will know how to handle those requests and send back responses to be printed on the screen.
Handle Request and Send Response
Let’s have a look at the “request” object that we used in the above example inside the console.log() statement. This “request” object holds all the data of the incoming requests when we visit the URL: http://localhost:3000.
In the “request” object, we have a key with the name “headers”. This holds the information added to a request. Out of them, there is one important field we need right now to figure out the current URL. That is “req.url”. Refer to the following code snippet to handle a request and send back a response.
Execute the following command to start your server again:
node app.js
Now, revisit the following URL through your web browser:
http://localhost:3000
You will be able to see a welcome message printed on your screen.
Through the above gist, you have an idea about how you can send a response for a requested page or URL. This will become a lot easier when you will start to learn Express.js: A back end web application framework for Node.js.
You noticed, I told you to stop and restart the server. Well, how can we solve that problem, and tell the Node server to auto-restart when there is a change made to our code. Let’s learn that in the next section.
Install Nodemon for Auto-restarts
Nodemon is a command-line interface (CLI) utility developed by rem(https://twitter.com/rem) that wraps your Node app, watches the file system, and automatically restarts the process.
Here you will learn how to install, set up, and configure nodemon.
You can install nodemon globally with NPM:
npm install nodemon -g
Or, you can also install nodemon locally. While performing a local installation, we can install nodemon as a dev dependency:
npm install nodemon --save-dev
Once installed, we can use nodemon to start a Node script. Since we have an “app.js” file in our project, we can start it and watch for changes like this:
nodemon app.js
Now you have completed setting up Nodemon for your Node.js project.
What’s next?
Kudos! You have got an introduction to Node.js. You can go ahead and start exploring other features that Node.js provides. Once you mastered the basic features of Node.js, give Express.js (A back end web application framework for Node.js) a try. I’m sure you would love that.
If you enjoyed reading this post and have learnt something new, then please give a clap, share it with your friends, and follow me to get updates for my upcoming posts. You can connect with me at LinkedIn.
The Ultimate Guide To Get Started With Node.js was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Tara Prasad Routray
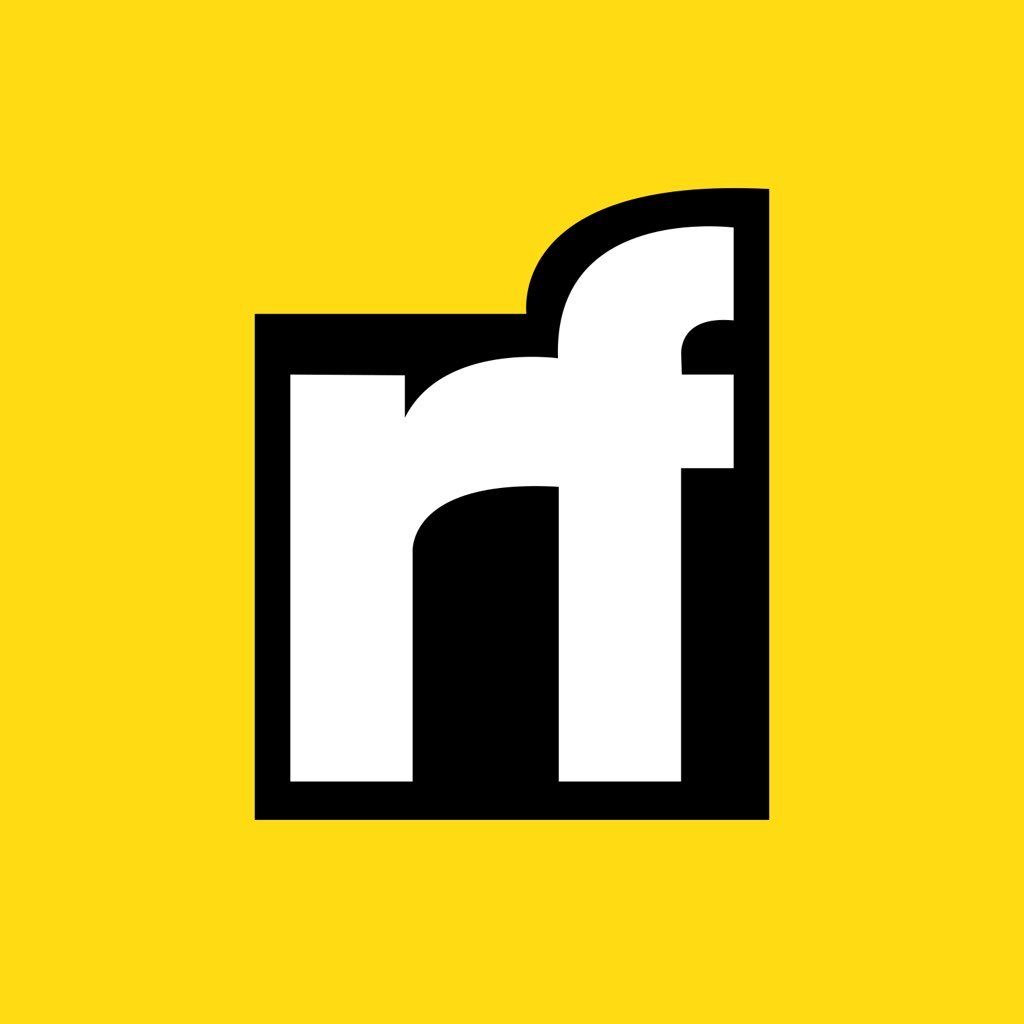
Tara Prasad Routray | Sciencx (2021-09-19T11:31:42+00:00) The Ultimate Guide To Get Started With Node.js. Retrieved from https://www.scien.cx/2021/09/19/the-ultimate-guide-to-get-started-with-node-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.