This content originally appeared on DEV Community and was authored by Cody Ogden
A recent project had me working with the Login by Auth0 again. It's a great, simple, "does one thing and doesn't try to take over the WordPress dashboard" plugin. In my opinion, this is the best kind of plugin. And while it has its issues around documentation, I was presented with some challenges around building custom login buttons that bypass the included Auth0 login screen.
Ideally, the user could click "Sign In with Google" or "Sign In with Apple," and they would be immediately sent to the services' login flow avoiding clicking through the example-tenant-domain.auth0.com login page. While Auth0's documentation for this plugin could be better, I was able to create separate buttons that act much more like a native SSO login experience.
How to skip the Auth0 Login page while using the Auth0 WordPress plugin?
The Auth0 plugin has a built-in way to auto-direct users to a specific login provider (i.e. Users can specify google-oauth2
and the login will automatically redirect to Google). We're going to co-opt this ability to build our own, automatically redirecting login URLs.
First, let's set a valid list of subscribers. This simple function will return a filterable array of SSO provider slugs that match our settings in Auth0.
function wpa0_sso_connections() {
$connections = array(
'google-oauth2',
'apple',
);
return apply_filters( 'wpa0_sso_connections', $connections );
}
Next, we'll build a function that can potentially replace all (or some) of the wp_login_url()
function. We can use this function in templating when we create specific buttons for provider logins, or a drop-in replacement where we'd normally use wp_login_url
. It takes three, optional arguments:
-
$connection
: The Auth0 provider slug. -
$redirect
: The URL the user should be redirected to after they successfully login. -
$force_reauth
: Allows us to force reauthorization even if a cookie is present (force a login on the user).
If no arguments are passed, it will simply return the default wp_login_url
, which will redirect users to the Auth0 login page.
function wpa0_sso_login_url( $connection = '', $redirect = '', $force_reauth = false ) {
$login_url = wp_login_url( $redirect, $force_reauth );
return apply_filters( 'wpa0_sso_login_url', (!empty($connection)) ? add_query_arg('connection', $connection, $login_url) : $login_url );
}
If you pass a provider slug to the $connection argument, you'll find that the wp_login_url
is returned with an extra query var. (e.g. https://example.com/wp-login.php?connection=google-oauth2
). This query var is key to letting us tell Auth0 to which provider it should automatically forward the user.
How to use auth0_get_auto_login_connection to redirect users?
Auth0 provides a filter called auth0_get_auto_login_connection
that does exactly what you might think: it returns the connection specified by a user in the plugin's settings. This filter is run during a user accessing the login link we generated above. We can return the connection slug we provided in the login link above, and the user will be automatically redirected to that provider's SSO.
add_filter( 'auth0_get_auto_login_connection', 'wpa0_auto_login_filter' );
function wpa0_auto_login_filter($connection) {
if (isset($_GET['connection']) && !empty($_GET['connection']) && in_array($_GET['connection'], array_keys(wpa0_sso_connections()))) {
return $_GET['connection'];
}
return $connection;
}
This function checks to ensure we have a connection query var and that it's not empty, then it checks to ensure the query var that was passed is valid by checking it against our wpa0_sso_connections
function. If all those conditions pass, it returns the connection query var, triggering the user's login attempt to be immediately forwarded to the provider.
How to create Auth0 automatic redirect login button?
Let's put it all together. In our fake templating file, let's pretend we have a link to Sign In with Apple.
<a>Sign In with Apple</a>
Let's add our wpa0_sso_login_url
function with the Apple connection slug, and then let's redirect users to /wp_admin
.
<a href="<?php wp_sso_login_url($connection = 'apple', admin_url()); ?>">Sign In with Apple</a>
The above will output:
<a href="https://example.com/wp-login.php?redirect_to=https%3A%2F%2Fexample.com%2Fwp-admin%2F&connection=apple">Sign In with Apple</a>
Notice the connection
query variable? This will be detected by our wpa0_auto_login_filter
when a user clicks. Since apple
is a valid Auth0 connection slug in our wpa0_sso_connections
function, the user will automatically be sent to the Apple sign in screen and then redirected to /wp-admin
.
Wrapping Up
You can place all these functions in your theme's functions.php
file or inside your custom plugin. Here's a Gist with doc comments that summarizes this post.
Thanks for reading! Follow me on Twitter! Originally published on my blog.
This content originally appeared on DEV Community and was authored by Cody Ogden
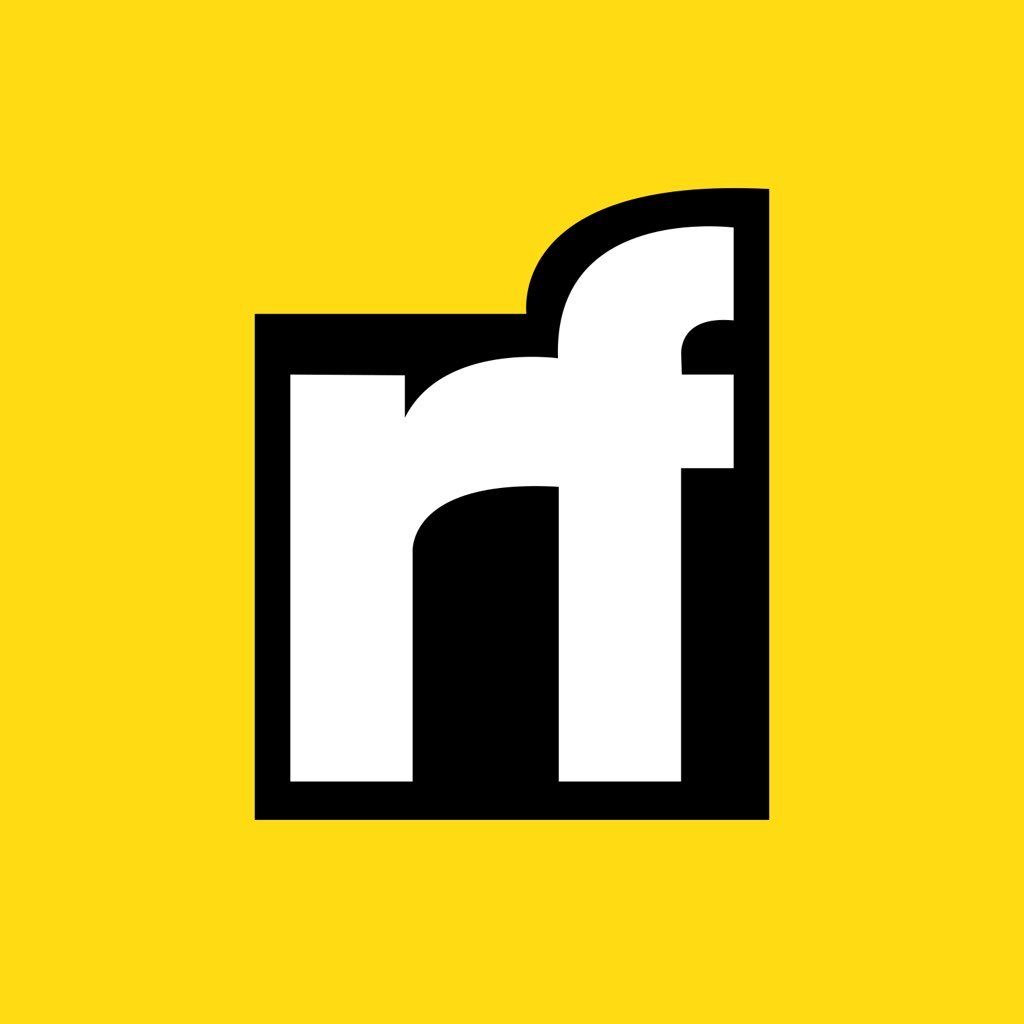
Cody Ogden | Sciencx (2021-09-30T22:17:08+00:00) Automatically Redirect Auth0 WordPress Users to SSO Login. Retrieved from https://www.scien.cx/2021/09/30/automatically-redirect-auth0-wordpress-users-to-sso-login/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.