This content originally appeared on Bits and Pieces - Medium and was authored by Ravidu Perera
Understanding the various methods for handling Time Zones in JavaScript.
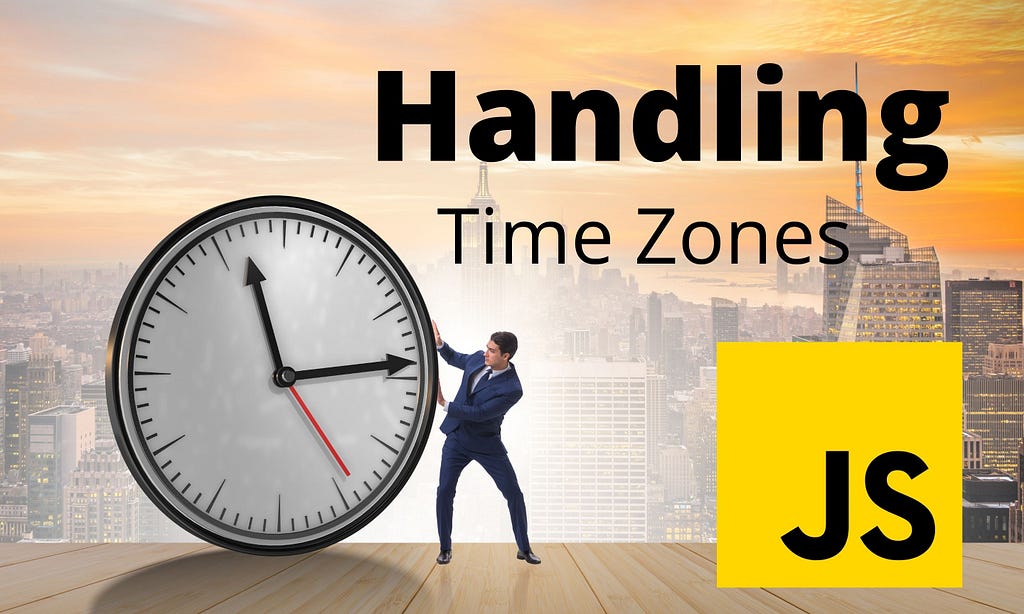
When building web applications, we typically consider two types of time zones. The most common one is server time, which act’s as the reference for date and time tracking, which is pretty straightforward.
However, there are instances where we need to track the end user’s date and time. In these situations, we need to capture the time from the user’s browser using JavaScript. Besides, these users could be from different time zones, and handling it could be tricky.
This article will discuss the concepts relevant to time zones and the methods to handle them using JavaScript.
Time Zones GMT, Offset, UTC
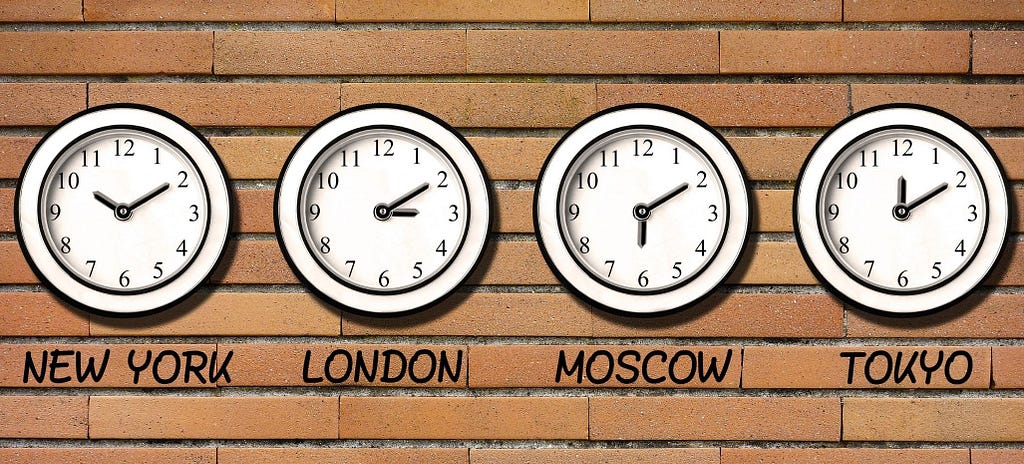
Before jumping into details, let’s understand some terminology around time zones.
1. GMT — Universal Reference
The world is divided into time zones, each with its offset. This offset is the number of minutes added to the world time to obtain each countries time zone.
To do that we need a reference which is the Greenwich Mean Time (GMT). This travels from the North Pole to the South Pole, going past the Greenwich district of London’s Old Royal Observatory.
If you are east of the Greenwich Meridian, your local time is usually ahead of GMT (for example, local time in China is GMT +8 hours). Conversely, local time is behind GMT west of the Greenwich Meridian (e.g. local time in New York is GMT -5 hours in winter and GMT -4 hours in summer).
2. Offset — Difference from the Reference
A time zone’s offset the number of hours or minutes it is ahead of or behind GMT.
Because of Daylight Saving Time, the offset of a time zone might shift throughout the year. In addition, laws can alter a time zone’s offset or daylight savings schedule. For example, when we state that Indiana switched from Central to Eastern Time, Indiana's time zones shifted from one offset to another.
It is typical for countries to name their time zones after themselves. For example, Korea’s time zone is known as KST (Korea Standard Time), and it has a specific offset value of KST = UTC+09:00.
2. UTC — Better alternative to GMT
Many people mistakenly believe that GMT and UTC are the same things, often used interchangeably. But what if I tell you that they are not the same?
UTC was created in 1972 to address the Earth’s rotational slowing problem. The caesium atomic frequency is used to set the time standard in this time system based on International Atomic Time. To put it another way, UTC is a better substitute for GMT. But, the difference is hardly noticeable for everyday development.
var d = new Date();
var n = d.getTimezoneOffset(); // Returns UTC Offset in Minutes
Using Date Time Processing with JavaScript in Practice
JavaScript provides Date objects with various methods to perform date and time-related calculations. However, you have the choice of using it or use more enhanced libraries to perform Date time processing in your code. Let’s first look at what we get with the JavaScript date object.
Date Time Operations — Using Pure JavaScript
The Date object in JavaScript manages time data using absolute numbers, such as Unix time, internally. Constructors and methods like parse(),getHour(),setHour().
However, it’s important to note that it is influenced by the client’s local time zone (the time zone of the OS running the browser, to be exact). As a result, generating a Date object from user input data will represent the client’s local time zone. Date object keeps track of time in UTC internally, but it usually accepts and outputs data in the local time of the computer it’s running on.
However, in JavaScript there are limited methods available natively when working with date time calculations across different time zones.
But, there is one operation that the date object can do with non-local time zones.
ISOString — It can parse a text containing any time zone’s numeric UTC offset. The output Date object does not keep the original local time and offset.
var d = new Date();
d.toISOString() //=> "2021-09-06T13:52:23.770Z"
d.valueOf() //=> 1630936367439 (this is what is actually stored in the object)
Date Time Operations — Using Third-Party Libraries
There are many libraries out there that provide a wide range of date formatting and manipulation functions. We can divide these libraries into two types depending on whether they support ECMAScript Internationalization API or not.
Intl-based Libraries
New development should use one of these time zone implementations, which use the Intl API for their data:
- Luxon (successor to Moment.js)
- date-fns-tz (extension for date-fns )
Non-Intl Libraries
These libraries are kept up to date, but they are responsible for packaging their time zone data, which can be substantial.
- js-joda/timezone (extension for js-joda)
- date-fns-timezone (extension for older 1.x of date-fns)
- BigEasy/TimeZone
- tz.js
Also, it’s essential to avoid some of the discontinued libraries for new projects.
Discontinued Libraries
These libraries have been decommissioned and should not be used anymore.
Out of these, I’m sure most of you are familiar with Moment but, the Moment team now recommends future development with Luxon.
When you try to use an inbuilt library, my recommendation is to attempt to work with its documentation. Then, after you get some understanding of the basics, try to apply it to your code.
My Favorite — Date-fns
Date-fns is a small library having a simple API with a lot of little functions to play with. With almost 140 functions, Date-fn is referred to be Lodash for dates.
Date-fns can have the following advantages :
- Immutable and pure
- Native date
- Modular
- Fast
- Documentation
- I18n
- Typescript and Flow
The npm packages collection includes Date-fns. You may install date-fns with the command. npm install date-fns.
Date-fns is compatible with both CommonJS and ES modules.
You need to import the functions you need to start parsing and displaying dates; you don’t need to import the entire API (only what you need). Let’s only show the current date.
//Import the function initially
const {format} = require('date-fns');
//today's date
const today =format(new Date(),'dd.MM.yyyy');
console.log(today);
Working with Time Zones
To work with UTC or ISO date strings, Date-fns supports time zone data. This will allow you to display the date and time in your users’ local time. The challenge comes when working with multiple time zones. Let’s look at how we can handle these situations using an example.
- The reference — First, a timestamp, UTC, or ISO date string represents a fixed point in time.
- Specific time zone — Second, the time zone descriptor (e.g. America/Los Angeles) is usually an offset or an IANA time zone name.
Note: You need to install the date-fns-tz library using npm install date-fns-tz.
- Zoned time to UTC
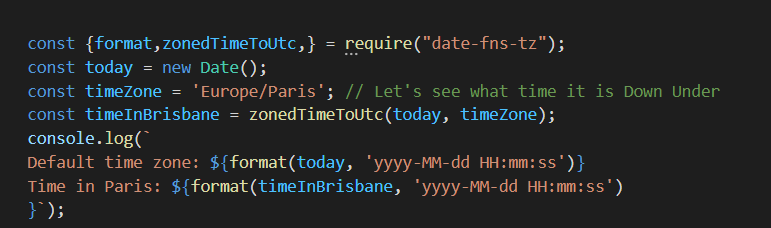
2. UTC to zoned time
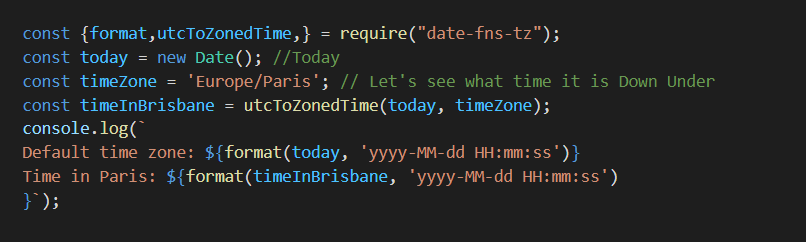
Conclusion
Several libraries can be used to work with time zones. They usually implement the standard IANA time zone database and provide functions for using it in JavaScript, although they can’t make the Date object behave any differently.
Modern libraries use the Intl API’s time zone data, while older libraries often have overhead, especially if running in a web browser, because the database might get rather large.
However, if you choose a library for a new project, select one that supports Intl API, which will likely be future-proof. Otherwise, if you are dealing with only a few Date time operations, using the native Date object is also fine.
I hope the article helped you determine multiple options to consider when working with time zones in JavaScript. If you have any other suggestions, please provide them in the comments below.
Thanks for reading !!
Build with independent components, for speed and scale
Instead of building monolithic apps, build independent components first and compose them into features and applications. It makes development faster and helps teams build more consistent and scalable applications.
OSS Tools like Bit offer a great developer experience for building independent components and composing applications. Many teams start by building their Design Systems or Micro Frontends, through independent components.
Give it a try →
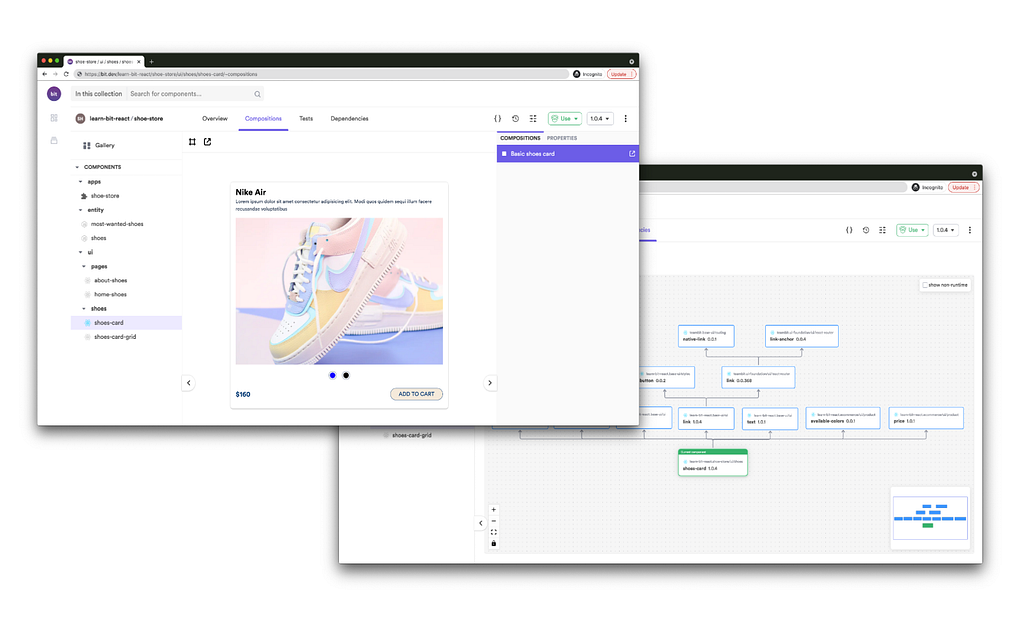
Learn More
- Date-fns vs MomentJS: Choosing the Right Date Utility Library
- 9 Javascript Time and Date Manipulation Libraries for 2019
- JavaScript Temporal API- A Fix for the Date API
How to handle Time Zones in JavaScript was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Ravidu Perera
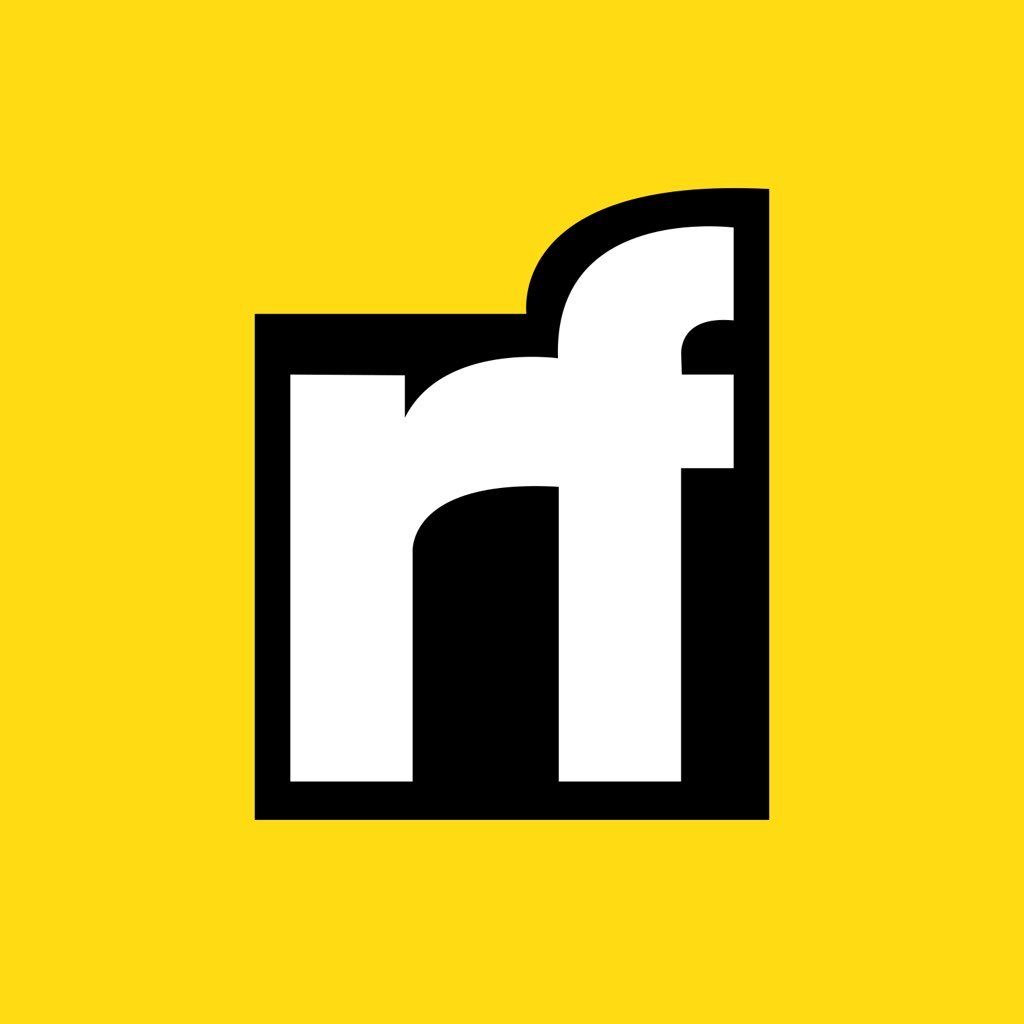
Ravidu Perera | Sciencx (2021-10-05T00:28:51+00:00) How to handle Time Zones in JavaScript. Retrieved from https://www.scien.cx/2021/10/05/how-to-handle-time-zones-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.