This content originally appeared on DEV Community and was authored by ynwd
This is a modification of the App.tsx
and App.test.tsx
CRAs. It uses useEffect
to get the text from the Golang API.
- Source code: https://github.com/ynwd/mnrp
- Live demo: https://fastro-319406.firebaseapp.com/
Backend
Golang API
package internal
import (
"context"
"github.com/fastrodev/fastrex"
)
func CreateApp() fastrex.App {
ctx := context.Background()
app := fastrex.New()
app.Ctx(ctx)
app.Get("/api", func(req fastrex.Request, res fastrex.Response) {
res.Send("The best interface is no interface")
})
return app
}
Entry point
package serverless
import (
"net/http"
"github.com/ynwd/mnrp/internal"
)
func Main(w http.ResponseWriter, r *http.Request) {
internal.CreateApp().Serverless(true).ServeHTTP(w, r)
}
Frontend
App.tsx
import React, { useEffect, useState } from 'react';
import logo from './logo.svg';
import './App.css';
function App() {
const [value, setValue] = useState("");
useEffect(() => {
async function getText() {
let response = await fetch('/api')
const d = await response.text()
setValue(d)
}
getText()
}, [value]);
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<h3>
{value}
</h3>
</header>
</div>
);
}
export default App;
App.test.tsx
import React from 'react';
import { rest } from 'msw';
import { setupServer } from 'msw/node';
import { render, screen } from '@testing-library/react';
import App from './App';
const server = setupServer(
rest.get('/api', async (req, res, ctx) => {
return res(ctx.text("The best interface is no interface"));
})
);
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
test('loads and displays greeting', async () => {
render(<App />);
const linkElement = await screen.findByText('The best interface is no interface');
screen.debug()
expect(linkElement).toBeInTheDocument();
});
This content originally appeared on DEV Community and was authored by ynwd
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
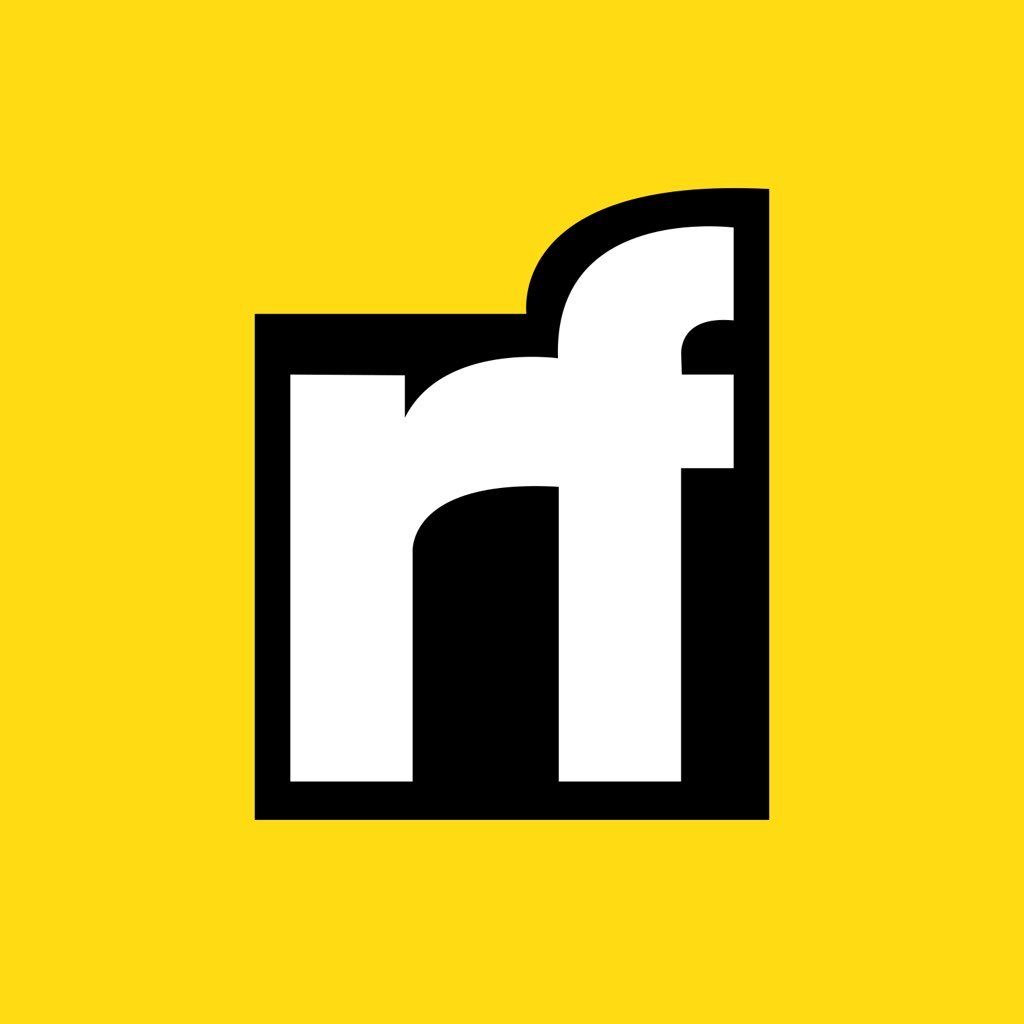
APA
MLA
ynwd | Sciencx (2021-11-07T08:38:47+00:00) UseEffect React Testing. Retrieved from https://www.scien.cx/2021/11/07/useeffect-react-testing/
" » UseEffect React Testing." ynwd | Sciencx - Sunday November 7, 2021, https://www.scien.cx/2021/11/07/useeffect-react-testing/
HARVARDynwd | Sciencx Sunday November 7, 2021 » UseEffect React Testing., viewed ,<https://www.scien.cx/2021/11/07/useeffect-react-testing/>
VANCOUVERynwd | Sciencx - » UseEffect React Testing. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2021/11/07/useeffect-react-testing/
CHICAGO" » UseEffect React Testing." ynwd | Sciencx - Accessed . https://www.scien.cx/2021/11/07/useeffect-react-testing/
IEEE" » UseEffect React Testing." ynwd | Sciencx [Online]. Available: https://www.scien.cx/2021/11/07/useeffect-react-testing/. [Accessed: ]
rf:citation » UseEffect React Testing | ynwd | Sciencx | https://www.scien.cx/2021/11/07/useeffect-react-testing/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.