This content originally appeared on DEV Community and was authored by Hassib Moddasser
JSON stands for JavaScript Object Notation. It's a lightweight format for storing and transporting data similar to XML or YAML.
In this article, I'll summarize everything you need to know about JSON in a simple describing language and then I'll provide coding snippets on how you can send/receive JSON data using JavaScript.
Let's dive in!
Introduction
JSON which stands for JavaScript Object Notation is lightweight, easy to read and write, and language-independent data interchange format. It is used widely across the internet for almost every single API as well as for config files and many other places. Even VS Code (Visual Studio Code) stores all of your configurations in a settings.json
file.
JSON is based on a subset of the JavaScript Programming Language Standard ECMA-262 3rd Edition - December 1999. It is easy to read and write compared to something like XML because it has a much cleaner and simpler syntax. It is a text format that is completely language-independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, Java, etc. JSON
Douglas Crockford, the writer of JavaScript: The Good Parts originally specified the JSON format in the early 2000s. Wikipedia
JSON filenames have the extension of .json
at the end.
JSON Syntax
- Data is in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
- Keys & string typed values should be wrapped in double quotes
Look at the example below that how a common JSON object looks like:
{
"id": 27,
"name": "Hassib Moddasser",
"twitter": "https://twitter.com/hassibmoddasser",
"verified": true,
"skills": [
{
"id": 1,
"name": "JavaScript"
},
{
"id": 2,
"name": "React.js"
},
{
"id": 3,
"name": "Node.js"
}
]
}
Data types
Data types that can be used in JSON are:
- Number
- String
- Null
- Object (JSON Object)
- Boolean
- Array
The following data types are INVALID in JSON:
- Function
- Date
- undefined
JSON has eclipsed XML
JSON has eclipsed XML as the preferred data interchange format for web applications and web services. Here’s why:
- Easy mapping into data structures
- Almost all programming languages have libraries or functions that can read and write structures of JSON
- Simple and compact
- It was made to be user-friendly for both people and computers
- It’s flexible
Note: XML is a markup language much like HTML but it was designed to store and transport data.
Let's look at the example below that how a common JSON object looks like in an XML markup:
<user>
<id>27</id>
<name>Hassib Moddasser</name>
<twitter>https://twitter.com/hassibmoddasser</twitter>
<verified>true</verified>
<skills>
<skill>
<id>1</id>
<name>JavaScript</name>
</skill>
<skill>
<id>2</id>
<name>React.js</name>
</skill>
<skill>
<id>3</id>
<name>Node.js</name>
</skill>
</skills>
</user>
Yes, the JSON format is much easy to read and write. Aside from that, it takes much less space.
How to parse received JSON data?
Every language has its own API (Application Programming Interface) for sending/receiving JSON data. Let's look at how JavaScript does it:
When receiving data from an API, that data is always a string. In order to use it, you should parse the data with the JSON.parse
method and the data becomes a JavaScript object.
Look at the example below:
// Received data from an API
const response = '{"id":27,"name":"Hassib","verified":true}';
// Parsing received data
const user = JSON.parse(response);
console.log(user.id);
// Output: 27
console.log(user.name);
// Output: Hassib
That's it! You've just parsed a JSON string.
How to send JSON data to an API?
When sending data to an API or web server, the data has to be a string.
You can convert a JavaScript object using the JSON.stringify
method into a string in order to send it to an API or a web server.
Look at the example below:
const user = {
id: 27,
name: "Hassib",
verified: true
};
let userJSON = JSON.stringify(user);
console.log(userJSON);
// Output: '{"id":27,"name":"Hassib","verified":true}'
Yes, you can send JSON data that simply!
Before you leave
If you would love to read even more content like this, feel free to visit me on Twitter and LinkedIn.
I'd love to count you as my ever-growing group of awesome friends! :)
This content originally appeared on DEV Community and was authored by Hassib Moddasser
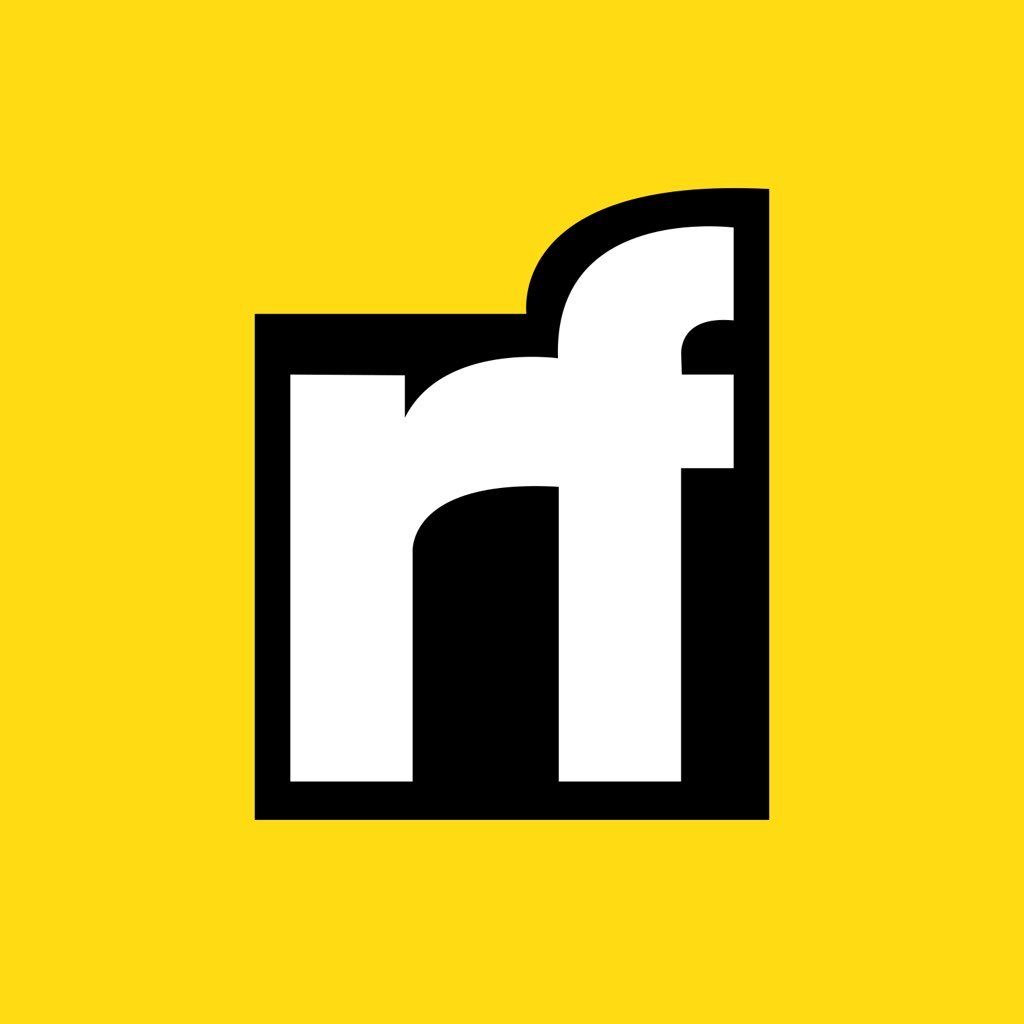
Hassib Moddasser | Sciencx (2021-11-10T19:36:18+00:00) What is JSON? — Everything you need to know about. Retrieved from https://www.scien.cx/2021/11/10/what-is-json-everything-you-need-to-know-about/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.