This content originally appeared on DEV Community and was authored by Bertug Korucu
Both shell_exec()
and exec()
do the job - until they don't.
If your command crash for some reason, you won't know - shell_exec()
and exec()
don't throw Exceptions. They just fail silently. 😱
So, here is my solution to encounter this problem:
use Symfony\Component\Process\Process;
class ShellCommand
{
public static function execute($cmd): string
{
$process = Process::fromShellCommandline($cmd);
$processOutput = '';
$captureOutput = function ($type, $line) use (&$processOutput) {
$processOutput .= $line;
};
$process->setTimeout(null)
->run($captureOutput);
if ($process->getExitCode()) {
$exception = new ShellCommandFailedException($cmd . " - " . $processOutput);
report($exception);
throw $exception;
}
return $processOutput;
}
}
It utilises Symfony's Process (which comes out of the box to Laravel). ✨
With this way, I can throw a custom exception, log the command and the output to investigate, report it to my logs to investigate, etc.
No more failing silently 😇
Hope you like this little piece! If you do, please give it a ❤️
This content originally appeared on DEV Community and was authored by Bertug Korucu
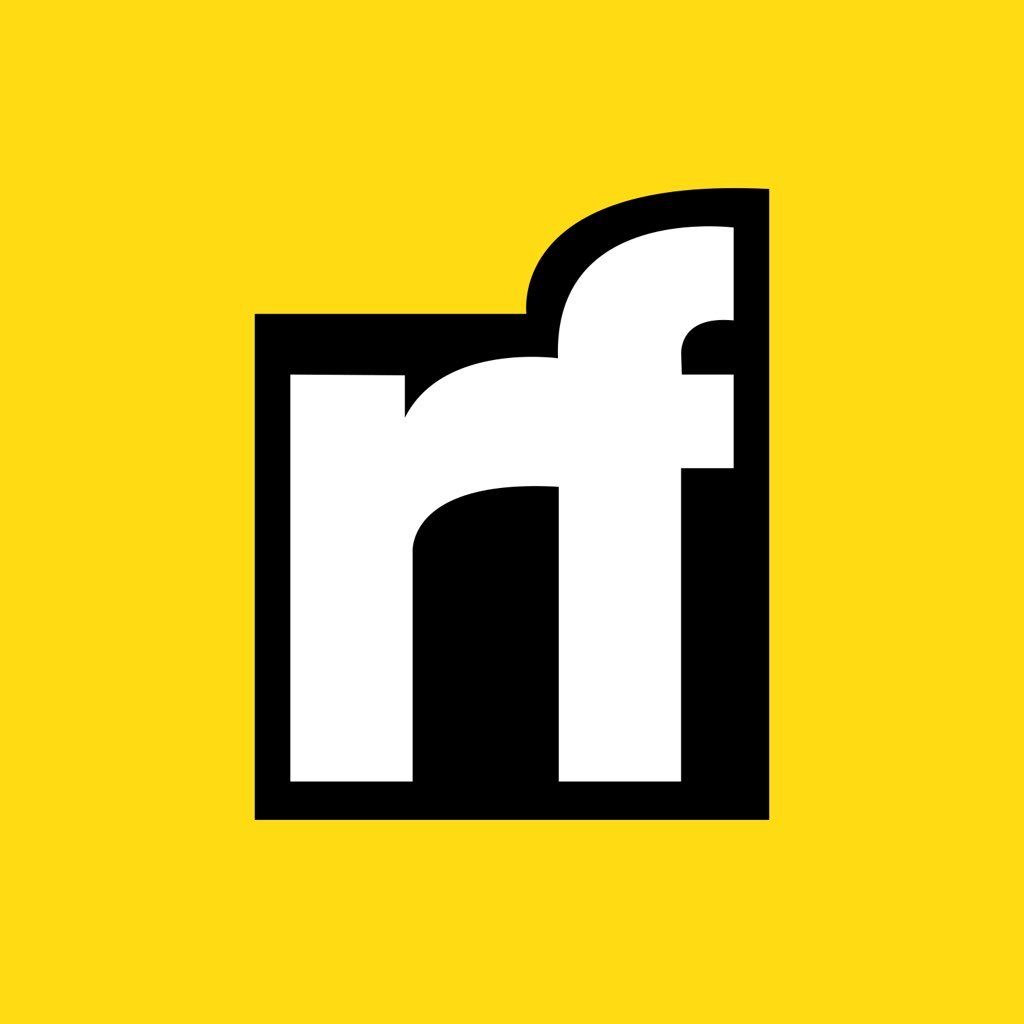
Bertug Korucu | Sciencx (2021-11-17T21:45:20+00:00) Executing Shell Commands In Laravel. Retrieved from https://www.scien.cx/2021/11/17/executing-shell-commands-in-laravel/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.