This content originally appeared on Level Up Coding - Medium and was authored by Anh Dang
Thought Programmer
Learn new concepts
Python is the fastest-growing programming language and here are a few ways to speed up your python code.
1. LISP Comprehension
Here’s a fantastic concept in python which is taken from LISP. Don’t use any other technique if you can use list comprehension because it takes less time to execute.
- Right way, time taken: 0.00012302398681640625 sec.
L = [i for i in range (1, 1500) if i%5 == 0]
- Wrong way, time taken: 0.00015282630920410156 sec.
L = []
for i in range (1, 1500):
if i%5 == 0:
L.append (i)
2. Use ‘.join’ operator insted of using ‘+=’ operator
Since Python considers a string as mutable, each time a string is assigned to a variable, a new object is created in the memory and assigns the new value.
- Right way, time taken: 3.266334533691406e-05 sec.
concatenated_string = "".join (["Python ", "is ", "fun."])
- Wrong way, time taken: 3.409385681152344e-05 sec.
concatenated_string = "Python " + "is " + "fun."
3. Don’t use ‘.’ operation
- Right way, time taken: 0.0006048679351806641 sec.
from math import sqrt
val = sqrt(100)
- Wrong way, time taken: 0.0006427764892578125 sec.
import math
val = math.sqrt(100)
4. Try to avoid if-else condition
Let’s try new ways to write your code efficiently. For example, you can avoid using if-else conditions in your program.
- Right way
if (not a_condition) or (not b_condition):
raise exception
do_something()
- Wrong way
if a_condition:
if b_condition:
do_something()
else:
raise exception
5. Use List slicing
Normally, list slicing operations are faster than using conventional loops. Here is how to generate odd numbers from a list of natural numbers.
- Right way, time taken: 0.0003399848937988281 sec.
N = [*range(1000)]
print(N[1:len(N):2])
- Wrong way, time taken: 0.0005199909210205078 sec.
N = [*range(1000)]
odd_num = []
for i in N:
if i % 2 != 0:
odd_num.append(i)
print(odd_num)
As we can see that the right way took half the time taken by wrong way.
In this post, I introduced a collection of some best ways that help to improve the performance of the code written in Python. Easy, right? Please apply them to your work.
And here’re top my 10 best articles
- Things I’ve Learned to Avoid in My 15 Years as a Software Engineer
- How to design a system to scale to your first 100 million users
- Famous Quotes About Software Engineer Everyone Should Know
- Stop using the ‘else’ keyword in your code
- How did I classify 50 chart types by purpose?
- Don’t be a STUPID programmer
- Software Architecture: The Most Important Architectural Patterns You Need to Know
- Rethinking SOLID principles in JavaScript
- These Bottlenecks Are Killing Your Code
- Top 20+ Github Repos Every Developer Must Know
Here are some tips to speed up your Python program was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anh Dang
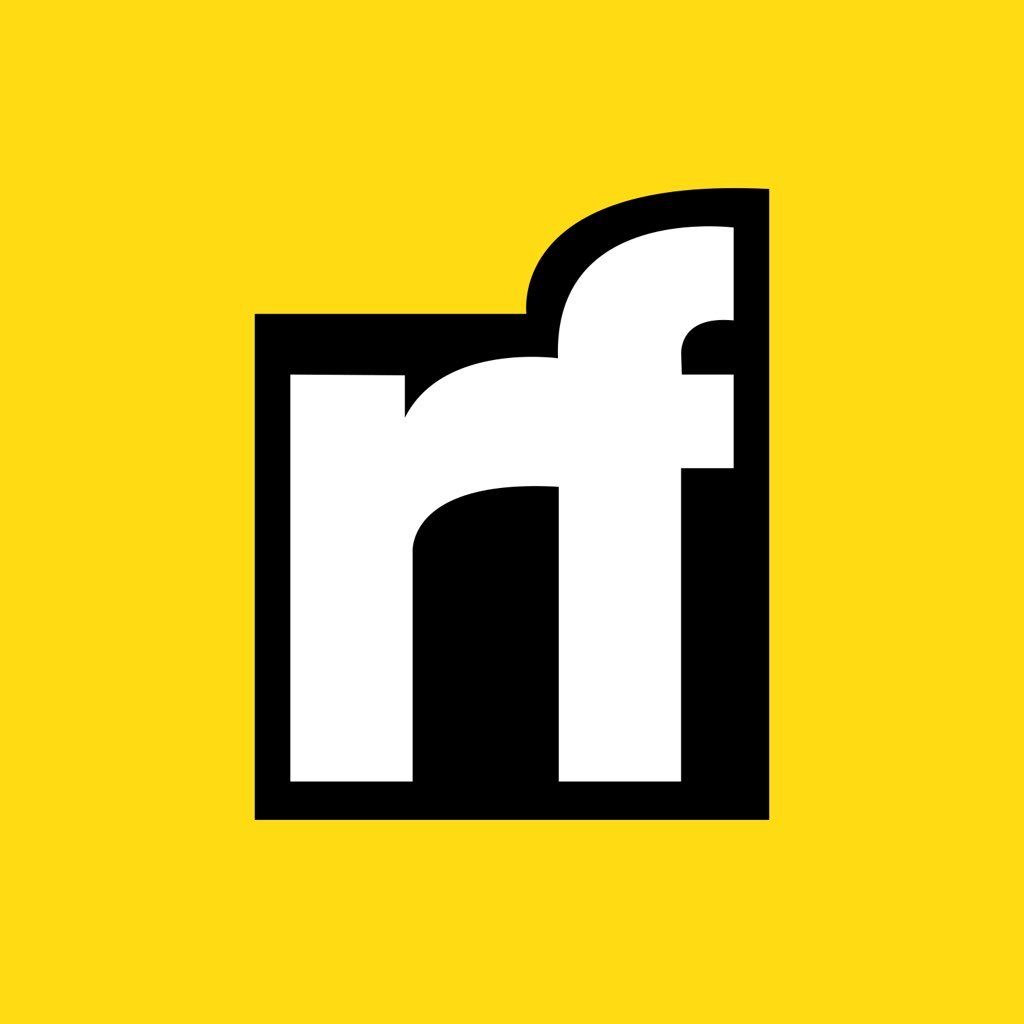
Anh Dang | Sciencx (2021-11-22T18:05:17+00:00) Here are some tips to speed up your Python program. Retrieved from https://www.scien.cx/2021/11/22/here-are-some-tips-to-speed-up-your-python-program/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.