This content originally appeared on DEV Community and was authored by Scott Gordon
# client_server_chat
# This is a program to demonstate a chat between client and server using UDP.
# Run this program and add either client or server as an argument.
# Once you have run both client and server you can chat between them.
# by Scott Gordon with help from Educative.com
import argparse, socket
MAX_SIZE_BYTES = 65535 # Mazimum size of a UDP datagram
def server(port):
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
hostname = "127.0.0.1"
s.bind((hostname, port))
print("Listening at {}".format(s.getsockname()))
while True:
data, clientAddress = s.recvfrom(MAX_SIZE_BYTES)
message = data.decode("ascii")
print("The client at {} says {!r}".format(clientAddress, message))
msg_to_send = input("Input message to send to client:")
data = msg_to_send.encode("ascii")
s.sendto(data, clientAddress)
def client(port):
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
host = "127.0.0.1"
while True:
s.connect((host, port))
message = input("Input message to send to server:")
data = message.encode("ascii")
s.send(data)
data = s.recv(MAX_SIZE_BYTES)
text = data.decode("ascii")
print("The server replied with {!r}".format(text))
if __name__ == "__main__":
funcs = {"client": client, "server": server}
parser = argparse.ArgumentParser(description="UDP client and server")
parser.add_argument("functions", choices=funcs, help="client or server")
parser.add_argument(
"-p", metavar="PORT", type=int, default=3000, help="UDP port (default 3000)"
)
args = parser.parse_args()
function = funcs[args.functions]
function(args.p)
Photo by Kelvin Ang on Unsplash
This content originally appeared on DEV Community and was authored by Scott Gordon
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
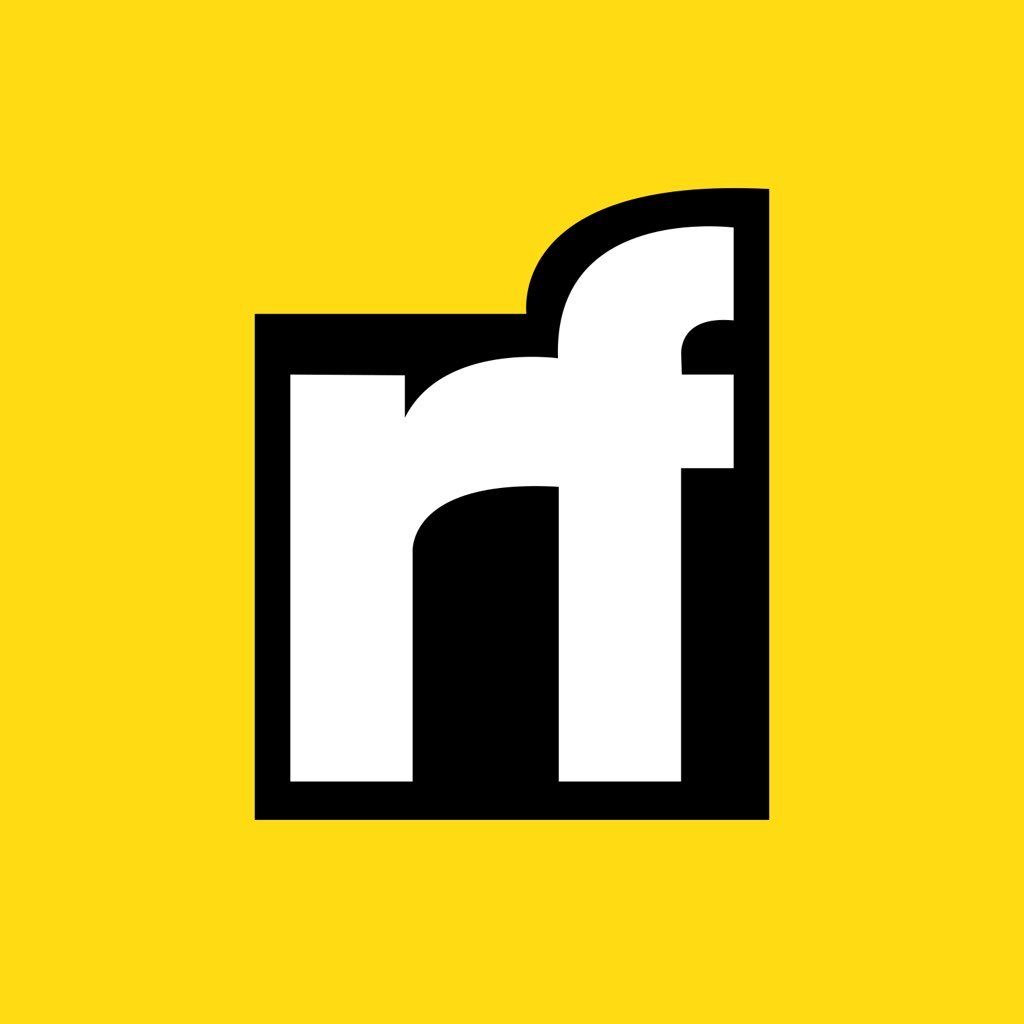
APA
MLA
Scott Gordon | Sciencx (2021-11-26T18:50:14+00:00) Client / Server Chat. Retrieved from https://www.scien.cx/2021/11/26/client-server-chat/
" » Client / Server Chat." Scott Gordon | Sciencx - Friday November 26, 2021, https://www.scien.cx/2021/11/26/client-server-chat/
HARVARDScott Gordon | Sciencx Friday November 26, 2021 » Client / Server Chat., viewed ,<https://www.scien.cx/2021/11/26/client-server-chat/>
VANCOUVERScott Gordon | Sciencx - » Client / Server Chat. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2021/11/26/client-server-chat/
CHICAGO" » Client / Server Chat." Scott Gordon | Sciencx - Accessed . https://www.scien.cx/2021/11/26/client-server-chat/
IEEE" » Client / Server Chat." Scott Gordon | Sciencx [Online]. Available: https://www.scien.cx/2021/11/26/client-server-chat/. [Accessed: ]
rf:citation » Client / Server Chat | Scott Gordon | Sciencx | https://www.scien.cx/2021/11/26/client-server-chat/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.