This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
This post is part of a new series where we build a clone of Airbnb with Next.js. See the first post here.
- Part 1: Let’s start by installing Next.js
- Part 2: Build the list of houses
- Part 3: Build the house detail view
- Part 4: CSS and navigation bar
- Part 5: Start with the date picker
- Part 6: Add the sidebar
- Part 7: Add react-day-picker
Now that we have the react-day-picker
library in place, we can start using it.
Let’s first define a component to hold it. We call it DateRangePicker
. I give it this name since we must define the range of dates for the booking.
Create a components/DateRangePicker.js
file. In it, we import the react-day-picker
component, and we just output a string to make sure our component displays in the page:
import DayPickerInput from 'react-day-picker/DayPickerInput'
export default function DateRangePicker() {
return (
<div>
<DayPickerInput />
</div>
)
}
Import this in the pages/houses/[id].js
file:
pages/houses/[id].js
//...
import DateRangePicker from '../../components/DateRangePicker'
export default function House(props) {
return (
<Layout
content={
<div className='container'>
...
<aside>
<h2>Choose a date</h2>
<DateRangePicker />
</aside>
...
</div>
}
/>
)
)
Here’s the output
But if you click the input element, ooops:
Go back to components/DateRangePicker.js
and also import the component’s CSS file provided in the npm package (not every package has a CSS file, this was mentioned in the package documentation):
components/DateRangePicker.js
import DayPickerInput from 'react-day-picker/DayPickerInput'
import 'react-day-picker/lib/style.css'
export default () => (
<div>
<DayPickerInput />
</div>
)
Now we’ll get the date picker rendered nicely:
Great!
Now let’s go back to our components/DateRangePicker.js
file. We need to have a “check-in” box, and a “check-out” box. We can put them in 2 separate rows, with 2 labels:
export default function DateRangePicker() {
return (
<div className="date-range-picker-container">
<div>
<label>From:</label>
<DayPickerInput />
</div>
<div>
<label>To:</label>
<DayPickerInput />
</div>
</div>
)
}
Let’s add this bit of CSS to display this markup nicely:
import DayPickerInput from 'react-day-picker/DayPickerInput'
import 'react-day-picker/lib/style.css'
export default function DateRangePicker() {
return (
<div className="date-range-picker-container">
<div>
<label>From:</label>
<DayPickerInput />
</div>
<div>
<label>To:</label>
<DayPickerInput />
</div>
<style jsx>
{`
.date-range-picker-container div {
display: grid;
grid-template-columns: 30% 70%;
padding: 10px;
}
label {
padding-top: 10px;
}
`}
</style>
<style jsx global>
{`
.DayPickerInput input {
width: 120px;
padding: 10px;
font-size: 16px;
}
`}
</style>
</div>
)
}
This should be the final result of our lesson:
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
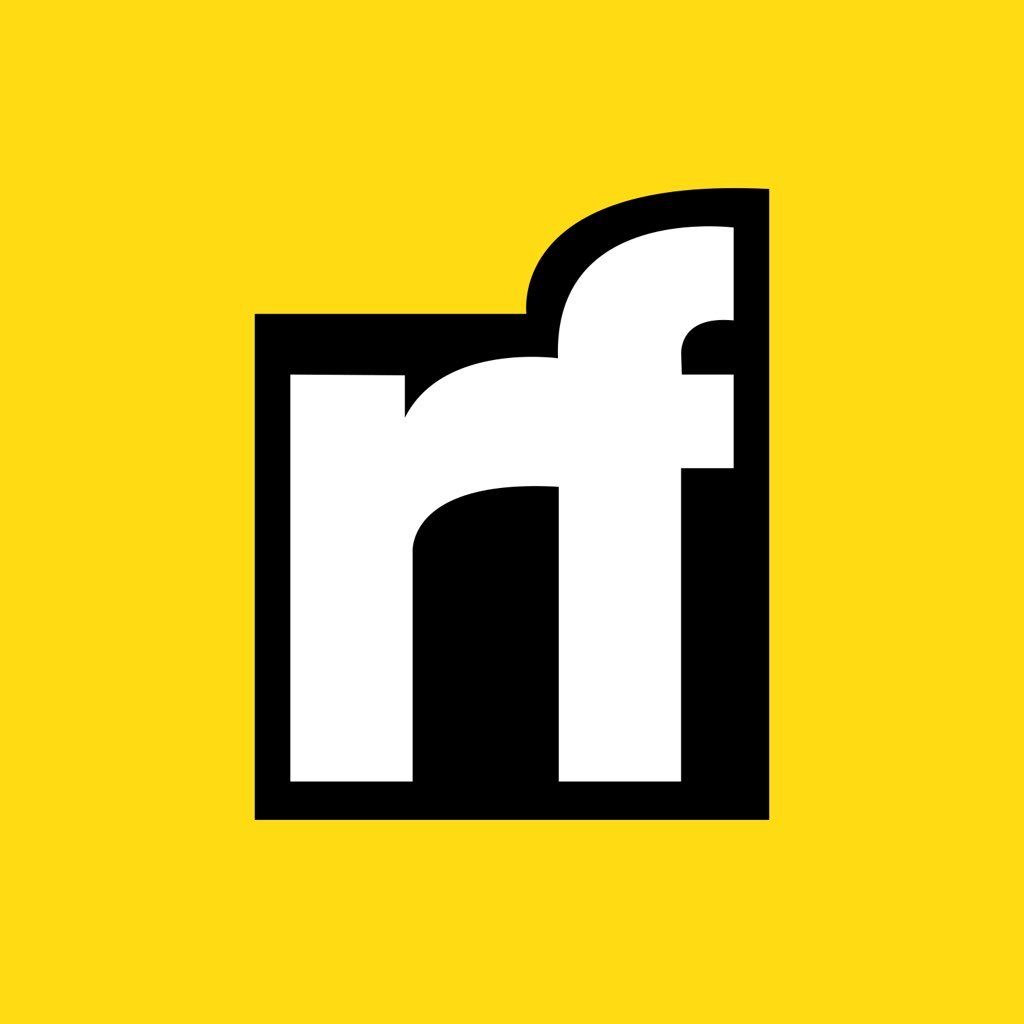
flaviocopes.com | Sciencx (2021-12-08T05:00:00+00:00) Airbnb clone, add the calendar to the page. Retrieved from https://www.scien.cx/2021/12/08/airbnb-clone-add-the-calendar-to-the-page/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.