This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
This post is part of a new series where we build a clone of Airbnb with Next.js. See the first post here.
- Part 1: Let’s start by installing Next.js
- Part 2: Build the list of houses
- Part 3: Build the house detail view
- Part 4: CSS and navigation bar
- Part 5: Start with the date picker
- Part 6: Add the sidebar
- Part 7: Add react-day-picker
- Part 8: Add the calendar to the page
- Part 9: Configure the DayPickerInput component
- Part 10: Sync the start and end dates
- Part 11: Show the price for the chosen dates
- Part 12: Login and signup forms
- Part 13: Activate the modal
- Part 14: Send registration data to the server
- Part 15: Add postgres
- Part 16: Implement model and DB connection
- Part 17: Create a session token
- Part 18: Implement login
- Part 19: Determine if we are logged in
We have a missing piece now. If we try to login, we are not immediately logged in - we must do a page reload, which is not nice.
Let’s login the user immediately after the login process is successful.
In components/LoginModal.js
we need to import the useStoreActions
object from easy-peasy
:
import { useStoreActions } from 'easy-peasy'
then inside the LoginModal
component we initialize setLoggedIn
and setHideModal
:
const setLoggedIn = useStoreActions((actions) => actions.login.setLoggedIn)
const setHideModal = useStoreActions((actions) => actions.modals.setHideModal)
Then we call them inside the submit()
function after we get a response:
const submit = async () => {
const response = await axios.post('/api/auth/login', {
email,
password
})
if (response.data.status === 'error') {
alert(response.data.message)
return
}
setLoggedIn(true)
setHideModal(true)
}
Here is the full component source code:
import { useState } from 'react'
import axios from 'axios'
import { useStoreActions } from 'easy-peasy'
export default function LoginModal(props) {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const setLoggedIn = useStoreActions((actions) => actions.login.setLoggedIn)
const setHideModal = useStoreActions((actions) => actions.modals.setHideModal)
const submit = async () => {
const response = await axios.post('/api/auth/login', {
email,
password
})
if (response.data.status === 'error') {
alert(response.data.message)
return
}
setLoggedIn(true)
setHideModal(true)
}
return (
<>
<h2>Log in</h2>
<div>
<form
onSubmit={(event) => {
submit()
event.preventDefault()
}}
>
<input
id="email"
type="email"
placeholder="Email address"
onChange={(event) => setEmail(event.target.value)}
/>
<input
id="password"
type="password"
placeholder="Password"
onChange={(event) => setPassword(event.target.value)}
/>
<button>Log in</button>
</form>
</div>
<p>
Don't have an account yet?{' '}
<a href="javascript:;" onClick={() => props.showSignup()}>
Sign up
</a>
</p>
</>
)
}
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
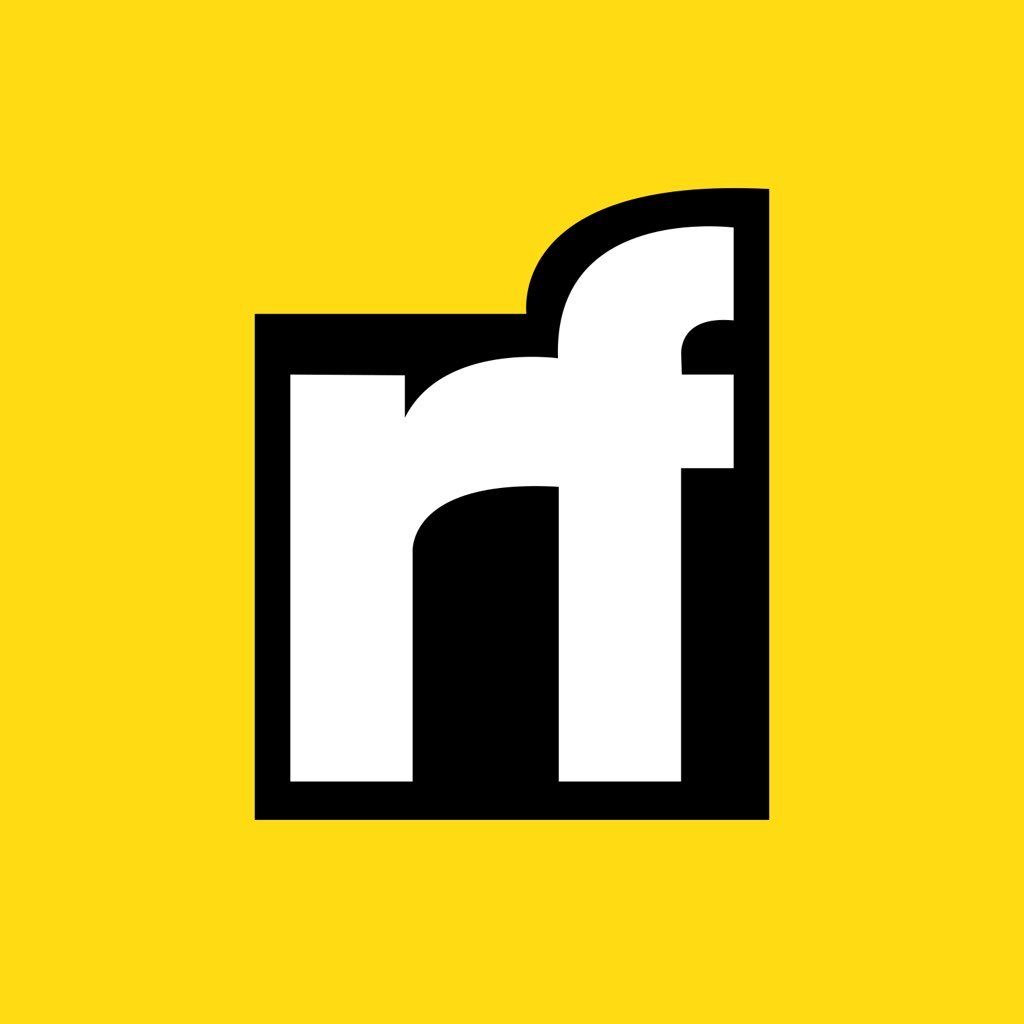
flaviocopes.com | Sciencx (2021-12-20T05:00:00+00:00) Airbnb clone, change state after we login. Retrieved from https://www.scien.cx/2021/12/20/airbnb-clone-change-state-after-we-login/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.