This content originally appeared on Level Up Coding - Medium and was authored by Randula Koralage
Passing data from the parent to a modal and getting a response from the modal to parent are very common situations we face when developing. This article gives a beginner-level demo but feel free to skip to the topics directly before it gets boring 🤠.
- How to pass data from parent to modal
- How to pass data from modal to parent
In this example, I use an item list component as the parent and add item form as the modal component 😉.
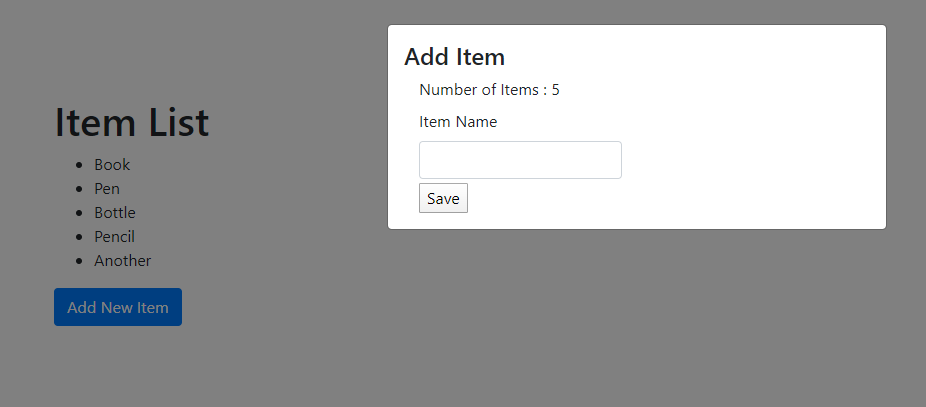
Initiate the project
First set up a new angular project and install ngx-bootstrap modal.
ng add ngx-bootstrap — component modals
Create a simple component ItemList to display a list of items added to an Array.
ng g c itemList
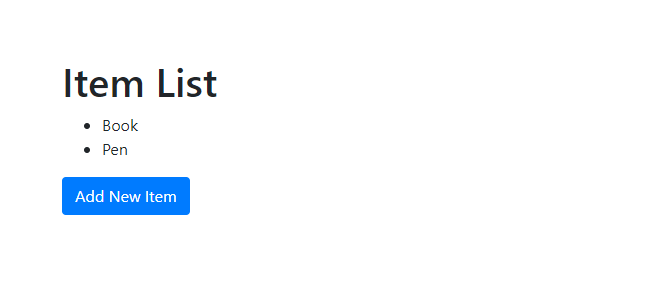
Let’s create another component to use as the modal.
ng g c itemAdd
The second component to add a new item, which will use as a modal in the future. Also, I have written a simple function called saveToList() which will also implement in the future. For now, I have put a simple console log, just to display the value in the input field.
numberOfItems will be the count of items in the existing array.
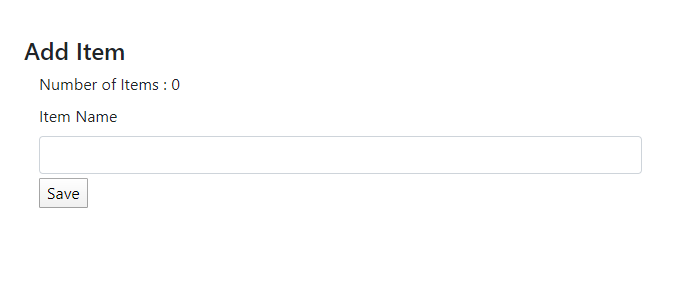
Add a modal
Let's change the code to open add item form as a modal when clicking the Add New Item button.
Don't forget to add ItemAddComponent into entryComponents list in app.module.ts
providers: [],
entryComponents: [ItemAddComponent],
Pass values from parent to modal
We can simply use initialState to pass an initial value into the modal 😊
To read this value from add Item modal,
Initialize a list inside modal, item-add.component.ts
list: any[] = [];
Then the initialState list can access as “list[]”.
We are sending a sample data “test” and it can be accessed as list[0] from item-add.component.html
<label>Number of Items : </label> {{list[0]}}
Think that we want to pass an object from the item list into modal.
<label>Number of Items : </label> {{list[0].value}}
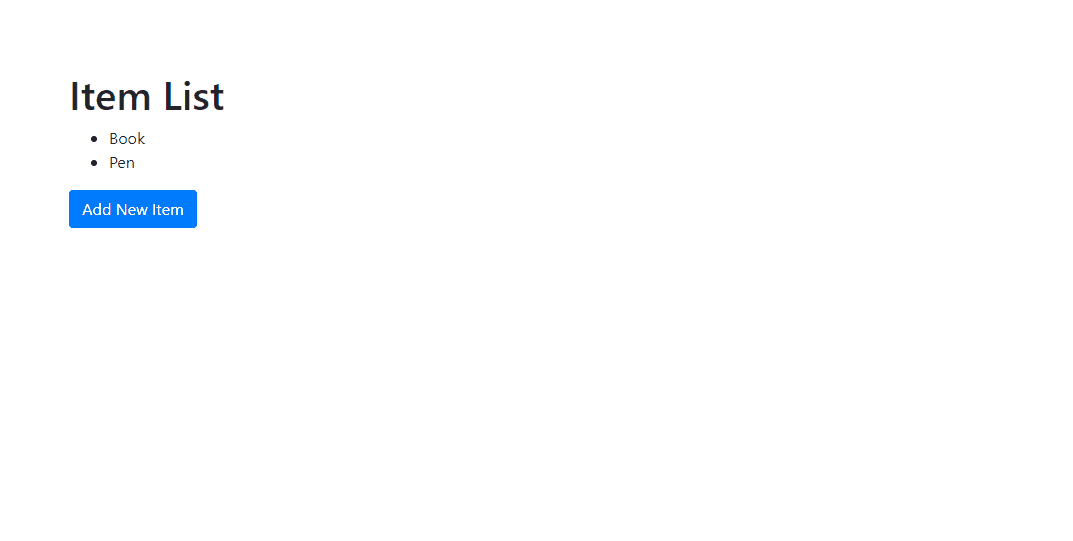
Pass values from modal to child
Let's see how to pass a value to the item list from the add item form. Declare an EventEmitter inside the item-add.component.ts
public event: EventEmitter<any> = new EventEmitter();
Emit an event when saving the item.
triggerEvent(item: string) {
this.event.emit({ data: item , res:200 });
}
And inside the item-list.component.ts we can subscribe to the event emitter.
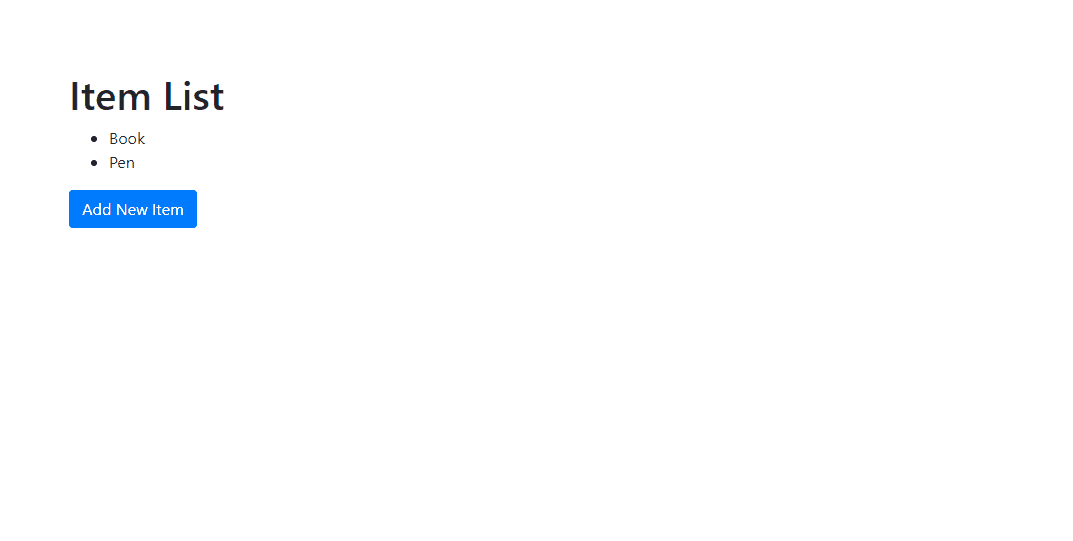
Full code can find here.
How to pass data between NGX Bootstrap modal and parent was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Randula Koralage
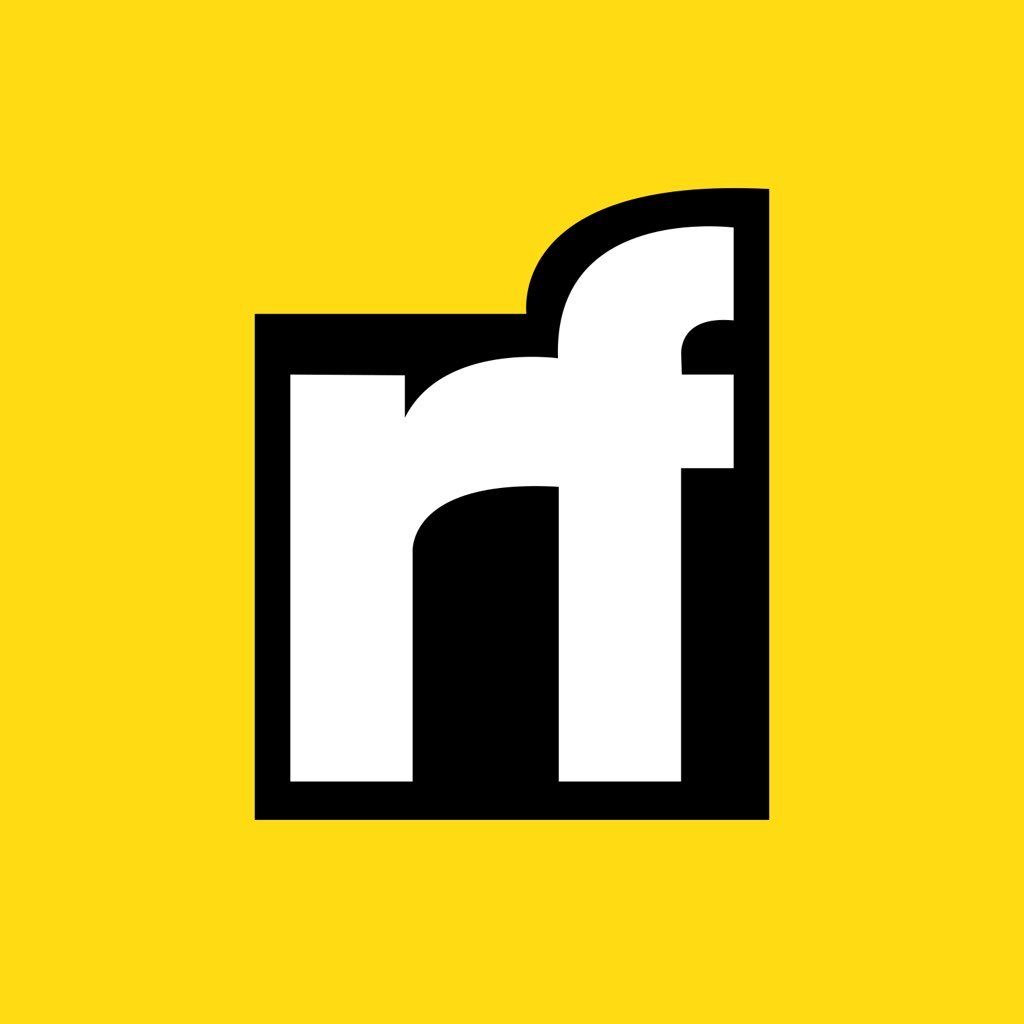
Randula Koralage | Sciencx (2021-12-25T13:15:58+00:00) How to pass data between NGX Bootstrap modal and parent. Retrieved from https://www.scien.cx/2021/12/25/how-to-pass-data-between-ngx-bootstrap-modal-and-parent/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.