This content originally appeared on DEV Community and was authored by Dylan Muraco
I've been working on a new project recently (CodeComponents if you wanna check it out) and struggled a little bit figuring out server side rendering because typescript wants to know what is being passed to the pages so I wanted to share how to do it.
so lets say we have a helper function that just returns all posts from the database
const prisma = new PrismaClient()
async function getPosts() {
const posts = await prisma.post.findMany()
return posts
}
Static Site Generation
export const getStaticProps: GetStaticProps<{
posts: Prisma.PromiseReturnType<typeof getPosts>
}> = async () => {
const posts = await getPosts()
return {
props: {
posts
}
}
}
const Home: NextPage<InferGetStaticPropsType<typeof getStaticProps>> = (
props: InferGetStaticPropsType<typeof getStaticProps>
) => {
return (
<div>
...
{/* do whatever with posts here */}
</div>
)
}
export default Home;
Server Side Rendering
Server Side rendering is pretty similar we just have to change the type of props passed to page function
export const getServerSideProps: GetServerSideProps<{
posts: Prisma.PromiseReturnType<typeof getPosts>
}> = async () => {
const posts = await getPosts()
return {
props: {
posts
}
}
}
const Home: NextPage<InferGetServerSidePropsType<typeof getServerSideProps>> = (
props: InferGetServerSidePropsType<typeof getServerSideProps>
) => {
return (
<div>
...
{/* do whatever with posts here */}
</div>
)
}
export default Home;
Hope this was helpful
This content originally appeared on DEV Community and was authored by Dylan Muraco
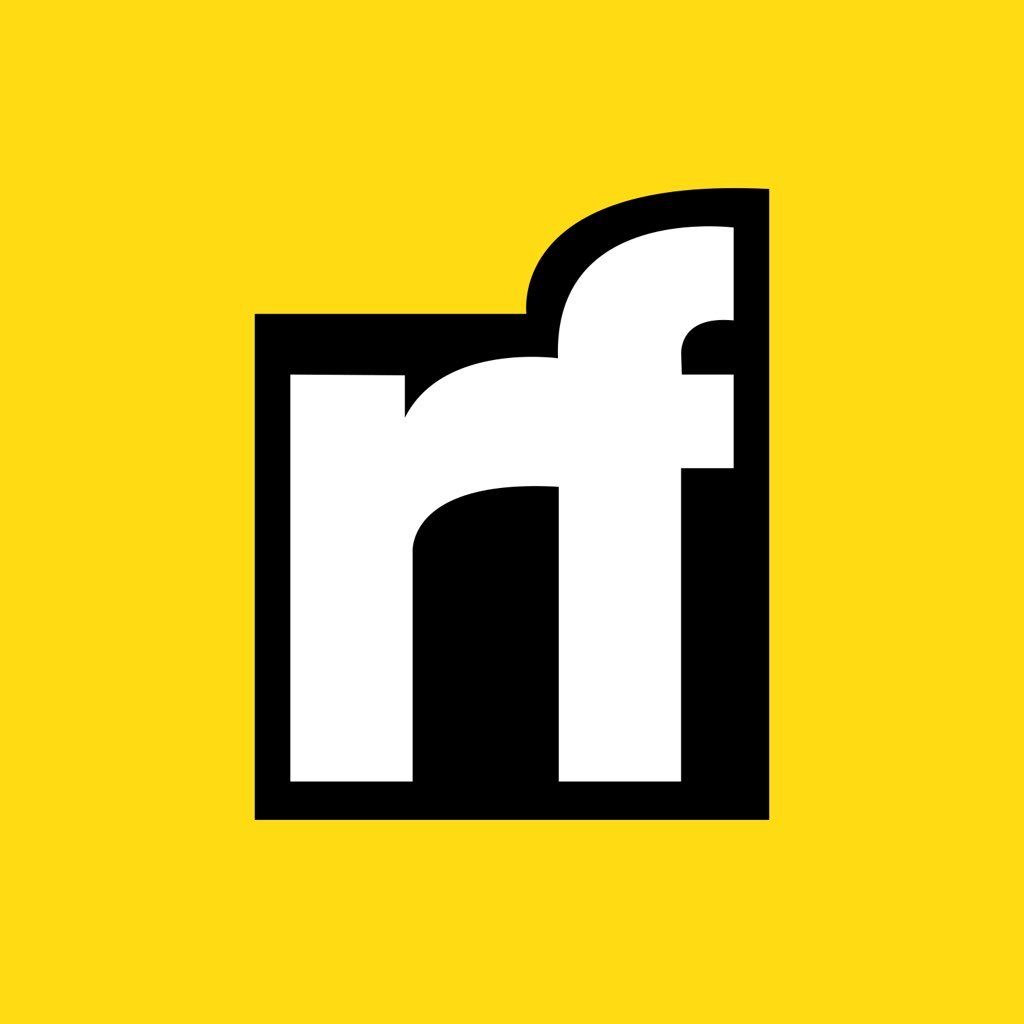
Dylan Muraco | Sciencx (2021-12-25T07:24:10+00:00) Server Side Rendering, Prisma + Next.js + TypeScript. Retrieved from https://www.scien.cx/2021/12/25/server-side-rendering-prisma-next-js-typescript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.