This content originally appeared on Level Up Coding - Medium and was authored by Manju
Let’s learn today to build a nodeJS application using express framework.
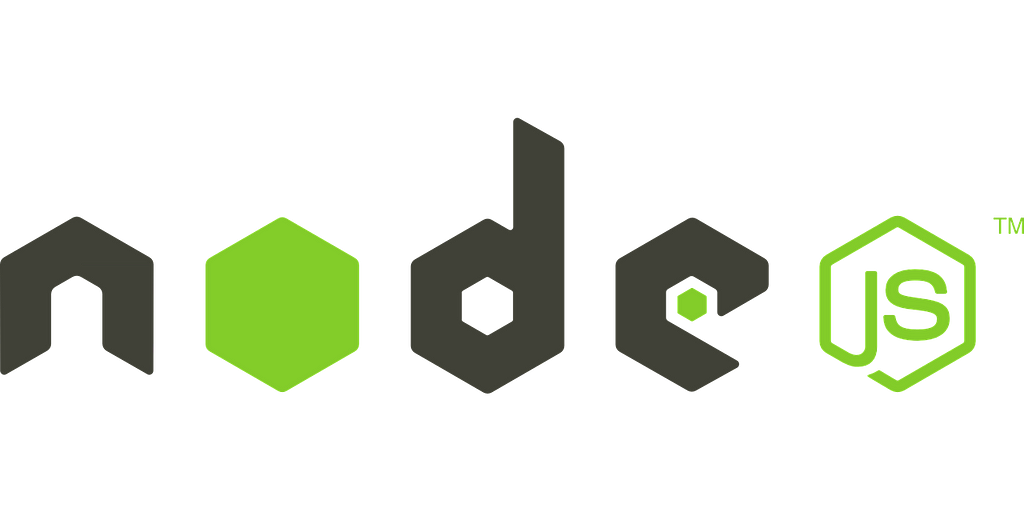
NodeJS is an open-source, cross-platform runtime environment built on the same JavaScript engine used in Google’s Chrome web browser. It allows developers to build server-side application middle tiers, including web servers and web services for platform APIs.
Express is the bare-bones web application framework on top of Node.JS that can be used to quickly build WebApps, RESTFUL APIs etc.
Now let us get started with building our first nodeJS application.
Prerequisites
Node is a must to be installed on our machine and secondly lets make sure that we have a user friendly editor to work on our project.
Installing Node
First we have to make sure that Node is installed on our machine.
We can verify it by running command node --version from the terminal. This command should show installed node version if already installed on the machine.
Otherwise to install node visit Node website and hit the big green install button.
Code Editor
We can use any code editor of our liking. But if you don’t have any code editor already installed on your system then I would highly recommend to use VS Code editor.
1. Setting up Project
First let’s create a directory where we would keep our source code.
mkdir my-nodejs-app
cd my-nodejs-app
Here my-nodejs-app is our project name.
2. Initializing project
Once we are inside our project folder my-nodejs-app, we will use command npm initto create package.json file for our application. This command prompts a few questions related to name, version, entry point for the application. For now we can keep it simple by passing option -y which sets all values to defaults.
npm init -y
Next we will initialise our project with typescript by creating tsconfig.json by using following command:
npx tsc --init
The above command generates a tsconfig.json file in our project directory. This tsconfig.json file allows us to configure and customise how TypeScript and the tsc compiler interact. In this project we will work with default configurations.
3. Installing Dependencies
Now we will install all the dependencies we require in our project by running following commands:
npm install express —S
npm i typescript ts-node nodemon @types/express @types/node -D
The option -D is used to install modules which are only required during development and the option -S is used to install modules required at the runtime.
The modules @types/node and @types/expressare type definitions for node and express modules.
We will use nodemon module for hot-reloading during the development of our application.
4. Create Server file
Now when our project setup is done, we can start implementing our actual server.
Let’s create index.ts file in our project and copy the following code in it.
Here we have imported express and initialised our server on port 8000 with a simple callback to log the status of our application.
We have created a simple GET route with empty base path which should return us a simple string in response.
5. Build and Run the Application
Now let’s update ourpackage.json file. First we will update scripts block by adding scripts to build and run our application.
Also we need to make sure that main which is the entry point of the application is pointing to our index.ts file
Now to build and run the aplication we will use this command,
npm run start
Now when we hit http://localhost:8000 on browser, we should see Welcome to node world!! which is the response returned from our GET API route.
Development with nodemon
Now let’s see how to run and test our application in development phase. We will use nodemon to provide us with hot reloading feature which enables us to simultaneously test as we code. For that lets run following command,
npm run start:dev
Now lets make changes to our route in index.ts
Here we can see that with every change saved in our index.ts file our application is rebuilding and new changes are reflected in our application.
6. Testing Node application
We surely do not want to rely on manual testing for our app to be error free. Here in this section we will learn to incorporate unit tests and integration tests in node application.
Here in this article we will rely on jest and supertest for writing our tests. so let’s go ahead and install the required dependencies.
npm i jest ts-jest supertest @types/supertest @types/jest -D
Unit Test
Unit tests are used to test small pieces of code or functions in isolation. To keep it simple, we will consider a simple add function for testing.
And here we write a unit test for the above mentioned simple add function.
To run the test, add following command to package.json in script block.
“test:unit”: “jest”
Integration Test
Integration tests are used to test multiple, bigger units (components) in interaction which in turn helps to ensure that the system is working properly as a whole.
We will use supertest which is a library that helps to assert network requests through another library superagent.
Below in the integration test we are testing aspects of network requests such as status codes and response data.
We are using jest to run our tests. We can give paths for our test files with jest command to run tests from specific test folder. Now we will update our package.json with script tags for test commands.
And our very first Node.js application is all ready to serve us with a warm welcome to Node world!!
Happy hacking and leave further questions in comments.
Build NodeJS server application with express using typescript was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Manju
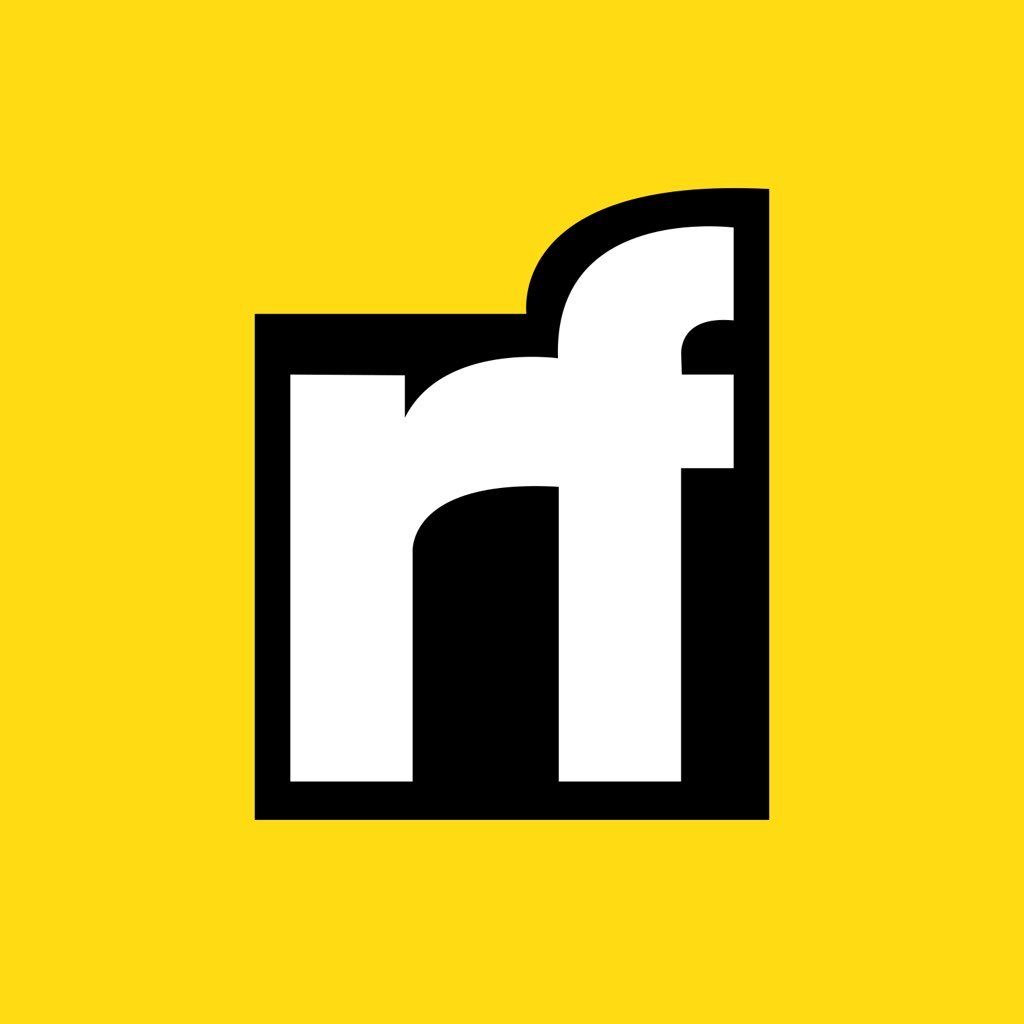
Manju | Sciencx (2021-12-29T23:02:35+00:00) Build NodeJS server application with express using typescript. Retrieved from https://www.scien.cx/2021/12/29/build-nodejs-server-application-with-express-using-typescript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.