This content originally appeared on DEV Community and was authored by Natalie Taktachev
In this post I will explain how to solve the longest word algorithm problem. This challenge asks to: return the longest word in a sentence as a string. If there are multiple words of the same length, return array.
Step 1.
Remove punctuation (like commas and periods). We will use .match on this to return a filtered array.
function longestWord(sentence) {
const words = sentence.match(/[a-z0-9]+/g) //global so it doesn't stop at first
}
Step 2. Sort by Length
We will use .sort and compare the words based on length in each iteration. They will be returned in sorted order.
function longestWord(sentence) {
const words = sentence.match(/[a-z0-9]+/g)
const sorted = words.sort(function(one, two){
return two.length - one.length
});
const longestArray = sorted.filter(function(word){
return word.length === sorted[0].length;
});
}
Step 3. Handle multiple words by putting them into array.
function longestWord(sentence) {
const words = sentence.match(/[a-z0-9]+/g)
const sorted = words.sort(function(one, two){
return two.length - one.length
});
const longestArray = sorted.filter(function(word){
return word.length === sorted[0].length;
});
}
Step 4. Check array length, and return based on length.
function longestWord(sentence) {
const words = sentence.match(/[a-z0-9]+/g)
const sorted = words.sort(function(one, two){
return two.length - one.length
});
const longestArray = sorted.filter(function(word){
return word.length === sorted[0].length;
});
}
This content originally appeared on DEV Community and was authored by Natalie Taktachev
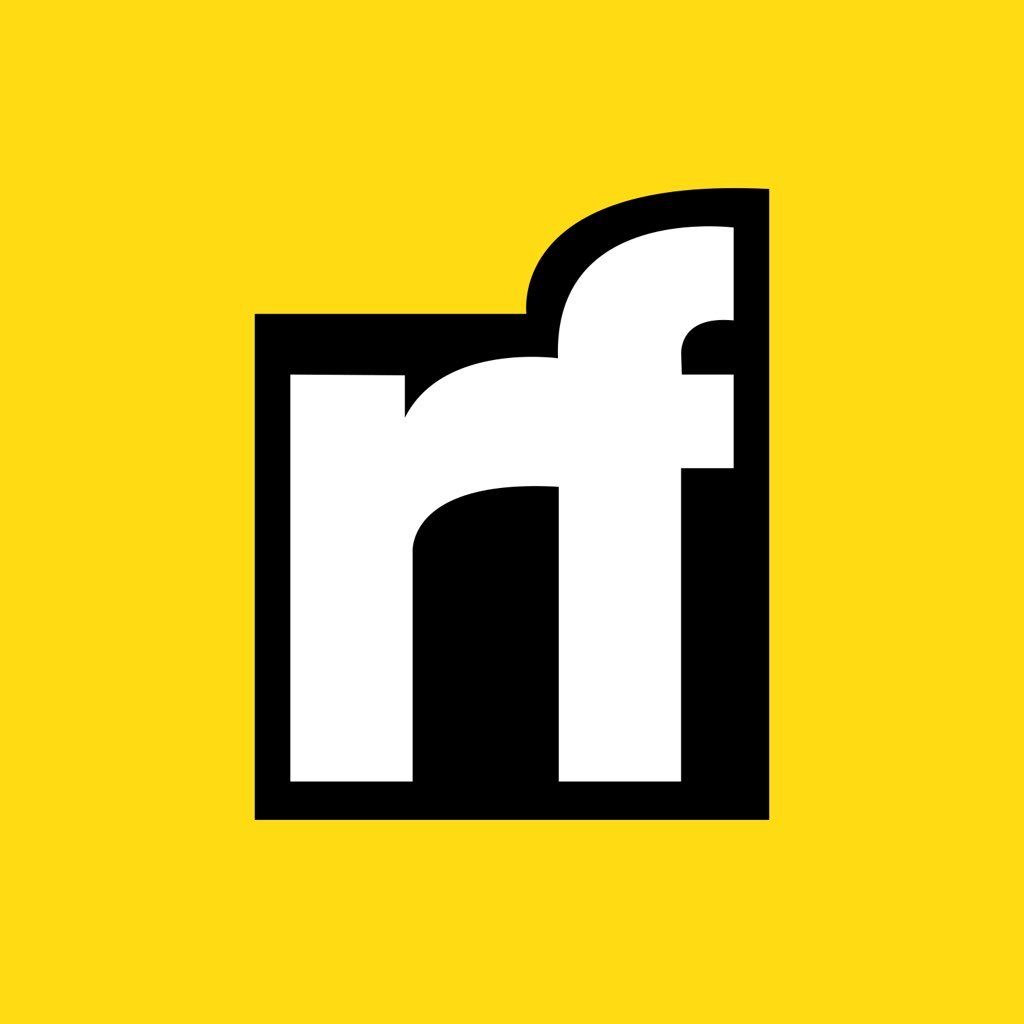
Natalie Taktachev | Sciencx (2022-01-03T00:07:25+00:00) Longest Word Algorithm. Retrieved from https://www.scien.cx/2022/01/03/longest-word-algorithm/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.