This content originally appeared on DEV Community and was authored by Suresh Ayyanna
**
Program to find Duplicate String and Letters from a Given string - Java(Collections)
**
package Test;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
import java.util.Set;
public class Program1 {
public static void findDuplicateWords(String str) {
// 1. Split the String - String Array formation
String[] s = str.split(" ");
// 2. Crate a HashMap to get key-value pair
HashMap<String, Integer> map = new HashMap<String, Integer>();
for (String temp : s) {
if (map.get(temp) != null) {
map.put(temp, map.get(temp) + 1);
} else
map.put(temp, 1);
}
// 3. To find duplicate words from keyValue set
Set<Map.Entry<String, Integer>> data = map.entrySet();
for (Map.Entry<String, Integer> dup : data) {
if (dup.getValue() > 1)
System.out.println("The word : '" + dup.getKey() + "' appeared - " + dup.getValue() + " times");
}
}
public static void findDuplicateLetters(String str) {
//1.Remove whitespaces
str = str.replace(" ", "");
//2.Convert to Lower case all letters
str=str.toLowerCase();
//3. create Hash map to get key-value pair
HashMap<Character, Integer> hmap = new HashMap<>();
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (hmap.get(ch) != null) {
hmap.put(ch, hmap.get(ch) + 1);
} else
hmap.put(ch, 1);
}
Set<Map.Entry<Character, Integer>> data = hmap.entrySet();
for (Map.Entry<Character, Integer> dup : data) {
if (dup.getValue() > 1)
System.out.println("The word : '" + dup.getKey() + "' appeared - " + dup.getValue() + " times");
}
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Enter the Stirng");
String str = input.nextLine();
findDuplicateWords(str);
findDuplicateLetters(str);
}
}
This content originally appeared on DEV Community and was authored by Suresh Ayyanna
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
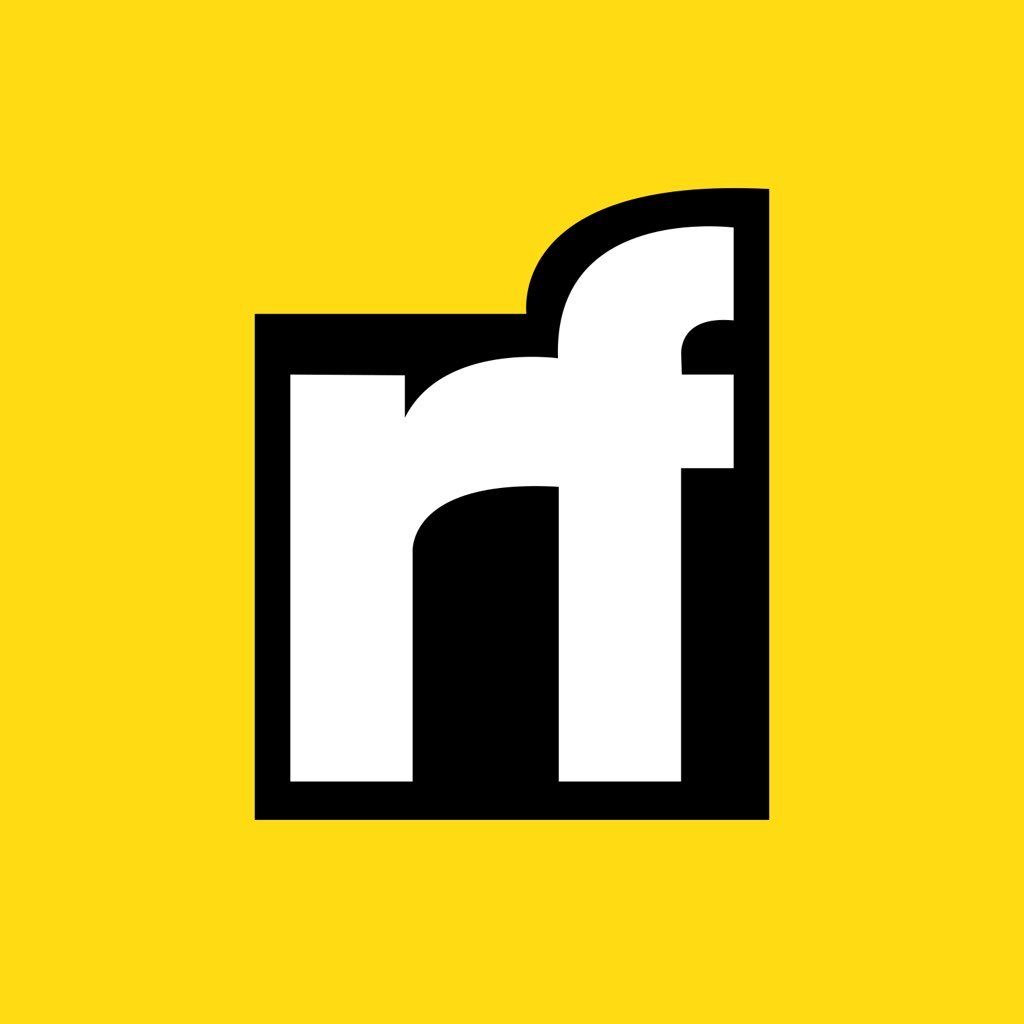
APA
MLA
Suresh Ayyanna | Sciencx (2022-01-22T11:34:31+00:00) Find Duplicate String and Letters from a Given string – Java(Collections). Retrieved from https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/
" » Find Duplicate String and Letters from a Given string – Java(Collections)." Suresh Ayyanna | Sciencx - Saturday January 22, 2022, https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/
HARVARDSuresh Ayyanna | Sciencx Saturday January 22, 2022 » Find Duplicate String and Letters from a Given string – Java(Collections)., viewed ,<https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/>
VANCOUVERSuresh Ayyanna | Sciencx - » Find Duplicate String and Letters from a Given string – Java(Collections). [Internet]. [Accessed ]. Available from: https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/
CHICAGO" » Find Duplicate String and Letters from a Given string – Java(Collections)." Suresh Ayyanna | Sciencx - Accessed . https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/
IEEE" » Find Duplicate String and Letters from a Given string – Java(Collections)." Suresh Ayyanna | Sciencx [Online]. Available: https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/. [Accessed: ]
rf:citation » Find Duplicate String and Letters from a Given string – Java(Collections) | Suresh Ayyanna | Sciencx | https://www.scien.cx/2022/01/22/find-duplicate-string-and-letters-from-a-given-string-javacollections/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.