This content originally appeared on DEV Community and was authored by Abayomi Ogunnusi
We'll learn how to conduct a search filter in React utilizing React Hooks and axios as our data fetching data source today.
Steps
Create a react app npx create-react-app .
Install axios: npm i axios
Create a component and name it SearchFilter
In your SearchFilter.jsx
import React from "react";
const SearchFilter = () => {
return (
<div>
<h1>Search filter page</h1>
</div>
);
};
export default SearchFilter;
In your App.js
import "./App.css";
import SearchFilter from "./SearchFilter";
function App() {
return (
<div className="App">
<SearchFilter />
</div>
);
}
export default App;
Run: npm start
Let's create an input to handle our search function in the SearchFilter
import React from "react";
const SearchFilter = () => {
return (
<div>
<h1>Search filter page</h1>
<input type="text" placeholder="enter search term ....." />
</div>
);
};
export default SearchFilter;
Now, let's visit [site]https://www.mockaroo.com/) to get our mock data.
Note: Ensure you select the JSON
option
Import your mock data into your project.
Let's flesh out the function to fire on every onchange.
import JSONDATA from "./MOCK_DATA.json";
import { useState } from "react";
const SearchFilter = () => {
const [searchTerm, setSearchTerm] = useState("");
return (
<div>
<input
type="text"
placeholder="enter search term ....."
onChange={(event) => {
setSearchTerm(event.target.value);
}}
/>
{JSONDATA.filter((val) => {
if (searchTerm === "") {
return val;
} else if (
val.first_name
.toLocaleLowerCase()
.includes(searchTerm.toLocaleLowerCase())
) {
return val;
}
}).map((val, key) => {
return (
<div className="user" key={key}>
<p>{val.first_name}</p>
</div>
);
})}
</div>
);
};
export default SearchFilter;
Explanation:
- We import the mock data gotten.
- Then imported our
useState
Hook. - initiated the state to an empty string.
- Created an onchange function
onChange={(event) => {
setSearchTerm(event.target.value);
}}
- To acquire our value, we used the filter and map functions, and we converted the filtered value to lowercase to avoid case sensitive errors.
Result:
Background Color from coolors.co
Source Code Link: Click
Conclusion
Thanks for reading, and be sure to check out my post on React Conditional Rendering here.
Resource
This content originally appeared on DEV Community and was authored by Abayomi Ogunnusi
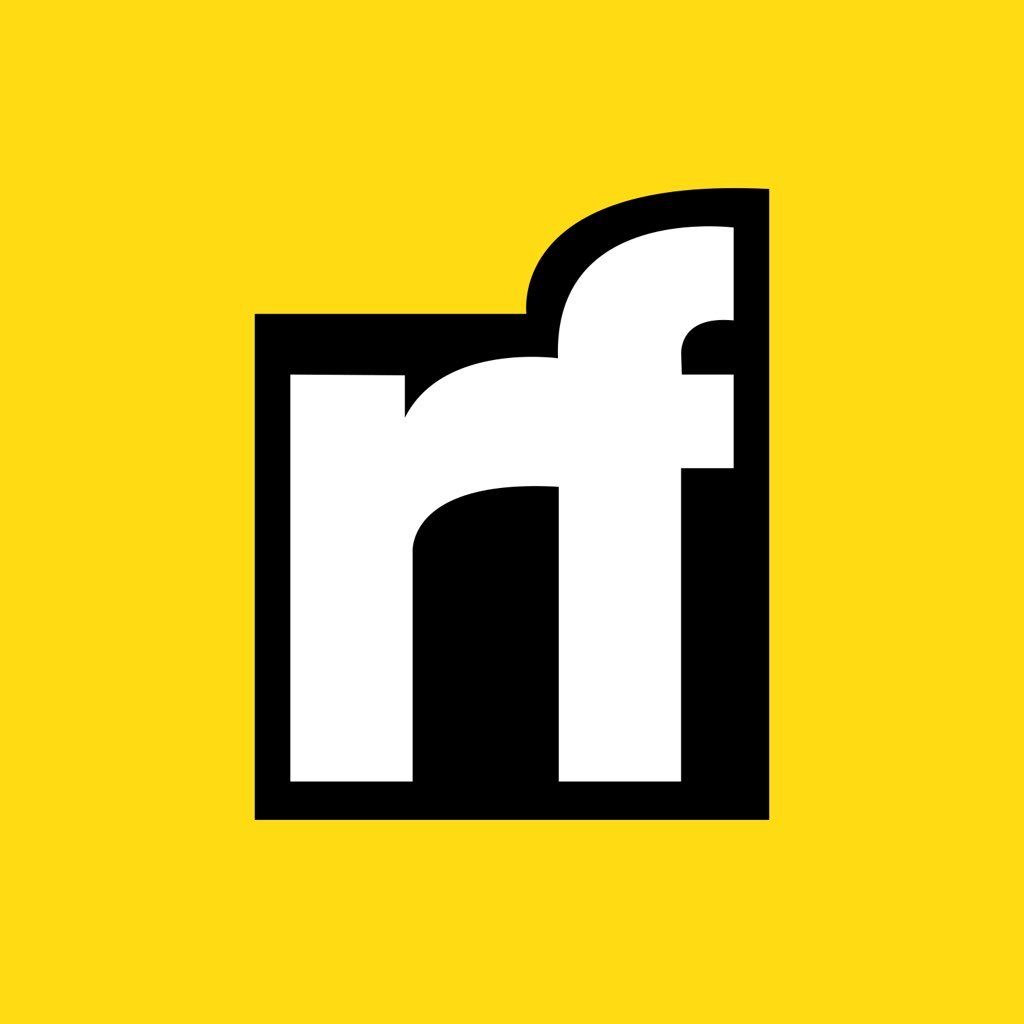
Abayomi Ogunnusi | Sciencx (2022-01-24T08:37:56+00:00) React Search Filter. Retrieved from https://www.scien.cx/2022/01/24/react-search-filter/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.