This content originally appeared on DEV Community and was authored by $dkelxldk
In this article, I just wanna share my experience on handling hundreds of concurrent request on sql transaction using sequelize.
At first, when i build my application. There was no problem during the test. The problem comes when the third party that I want to integrate with is testing my app with a stress test.
And in a result, my app can't handle hundreds of concurrent request. There was no problem with the nodejs. The problem was when the app trying to get a connection from a database pool, but there was no released connection on the pool. Because it was all in use.
My db pool config was :
pool: {
max: 38, // maximum number of pool connection
min: 0,
acquire: 60000, // 60 seconds acquire time
idle: 5000, // 5 seconds idle time
},
And here's the example code :
await Promise.all([
User.findByPk(1, { transaction }),
Gamelog.bulkCreate(gamelog, { transaction }),
Balancelog.bulkCreate(balancelog, { transaction })
]);
The solution was quite simple, it was an issue from the sequelize itself. https://github.com/sequelize/sequelize/issues/11024
pool.max transactions can run simultaneously, and the (pool.max + 1) transaction will cause a deadlock which completely blocks new transactions.
So we can't use async/await on this scenario, rather we use promises.
sequelize.transaction(async transaction => {
User.findByPk(1, { transaction });
Gamelog.bulkCreate(gamelog, { transaction });
Balancelog.bulkCreate(balancelog, { transaction });
})
.then(() => {
return true
})
.catch(err => return false)
This content originally appeared on DEV Community and was authored by $dkelxldk
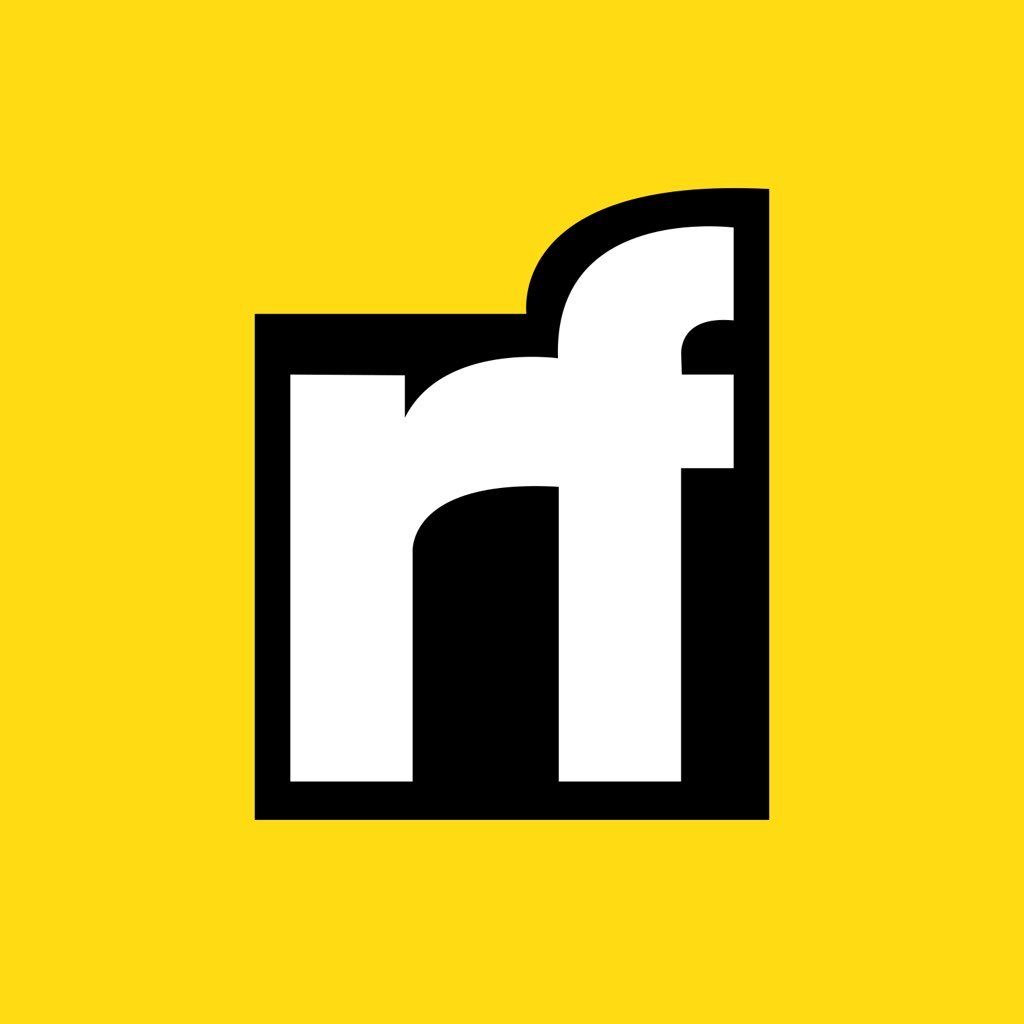
$dkelxldk | Sciencx (2022-02-07T08:05:12+00:00) Handling concurrent mysql transaction request with sequelize. Retrieved from https://www.scien.cx/2022/02/07/handling-concurrent-mysql-transaction-request-with-sequelize/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.