This content originally appeared on DEV Community and was authored by Mina
In Next js, data fetching is a strategy. Next.js uses getStaticProps or getServerSideProps to enhance different fetching capabilities. Even though both are used to pre-render data, the former uses static generation whilst the latter uses server side rendering.
Hmm... ok but do I need page pre-rendering?
Does the data you want to display change frequently? Does your data need to be updated frequently like a stock/crypto exchange dashboard or an online store showing its real time available stocks.
Does your page carry private data like user's personal information? Is it important for your data to appear on search engine results?
Do you prefer your page to be immediately available even with some partially available data rather than waiting for the data to be fully ready?
If you are ticking any of the questions above, it might be better just to stick to client-side fetching for better performance.
If you have decided to use GetStaticProps or GetServerSideProps, how would you use them differently?
Next.js doesn’t allow both Static Generation and SSR to be configured on the same page but you can opt to use either on each page to have different data fetching solutions for your application.
GetStaticProps and GetServerSideProps are async functions that need to be named exactly as they are and return an object that is passed as props and exported on a page like so.
export async function getStaticProps() {
const data = await fetch('https://.../products')
const products = await data.json()
return { props: { products } }
}
export async function getServerSideProps() {
const data = await fetch('https://.../products')
const products = await data.json()
return { props: { products } }
}
The code within the function only gets executed on the server and pre-renders the page. However, GetStaticProps pre-renders the data at build time, whereas getServerSideProps runs at request time.
GetStaticProps is best suited for data that doesn’t change frequently such as blog posts or news articles because unlike GetServerSideProps, when data is fetched, it generates a static HTML and stored in a JSON file so it can be cached and immediately available before loading. However, for less static and more dynamic pages, this can be leveraged by using an Incremental Static Generation feature. By simply adding another key value pair, a key named “revalidate” with its value set to any time in seconds in the returned statement, it tells the page to regenerate at every given second and updates the data even after the build time without deploying it again.
export async function getStaticProps() {
const data = await fetch('https://.../products')
const products = await data.json()
return {
props: { products },
revalidate: 10
}
}
Unlike getStaticProps, getServerSideProps doesn’t cache any data. It fetches new data on every request which often results in a slower performance but it has access to incoming requests or user’s specific data.
params is a parameter object that can be destructured from the context prop. It gives you access to the route, but in the getStaticProps function it only works for a default route. In order for it to work for a dynamic route, the getStaticPaths function has to come into play. It must return an object which includes two keys, a ‘paths’ key with an array that gives pre-generated route instances to be pre-rendered ahead of time and a ‘fallback’ key that can be set to false if all possible route instances are provided. The other option is to set the fallback to true if not all possible page routes are provided, which means it can have a fallback to render rarely visited pages. In case of the latter, a fallback state is needed (as in the condition below) so it can show a loading indicator before the data is fetched. Alternatively, you can set the fallback to a value in a string “blocking”, in this case your app will wait until the page gets fully generated before rendering data without any loading signs.
export async function getStaticPaths() {
return {
paths: [
{params: {product: ‘item1’ }},
{params: {product: ‘item2’ }},
{params: {product: ‘item3’ }},
],
fallback: false
}
}
export async function getStaticProps(context) {
const { params } = context
const data =
await fetch(`https://.../products/${params.product}`)
const product = await data.json()
return {
props: { product },
revalidate: 10
}
}
function Product (props) {
const { product } = props
if (!product) {
return <p>loading…</p>
}
return <div>
<p>{product.name}</p>
<p>{product.description}</p>
...
</div>
}
params is also supported in getServerSideProps and in fact, it is much simpler as it can extract both default and dynamic routes. In addition to that, the context props in getServerSideProps can give access to the req and res object that you can write server side code with.
export async function getStaticProps(context) {
const { params, req, res } = context
const data =
await fetch(`https://.../products/${params.product}`)
const product = await data.json()
return {
props: { product },
}
}
Next.js offers powerful pre-rendering data fetching options to optimize your app but as always, it is not the solution for all. Now you might be very tempted to use them but sometimes, it is just as good using the client side fetching to give better user experience - so planning what works best for you is key because it will save your time! Oh boy, how many times have I tried these fetching methods, only to realize that I am better off with the old traditional client side fetching.
This content originally appeared on DEV Community and was authored by Mina
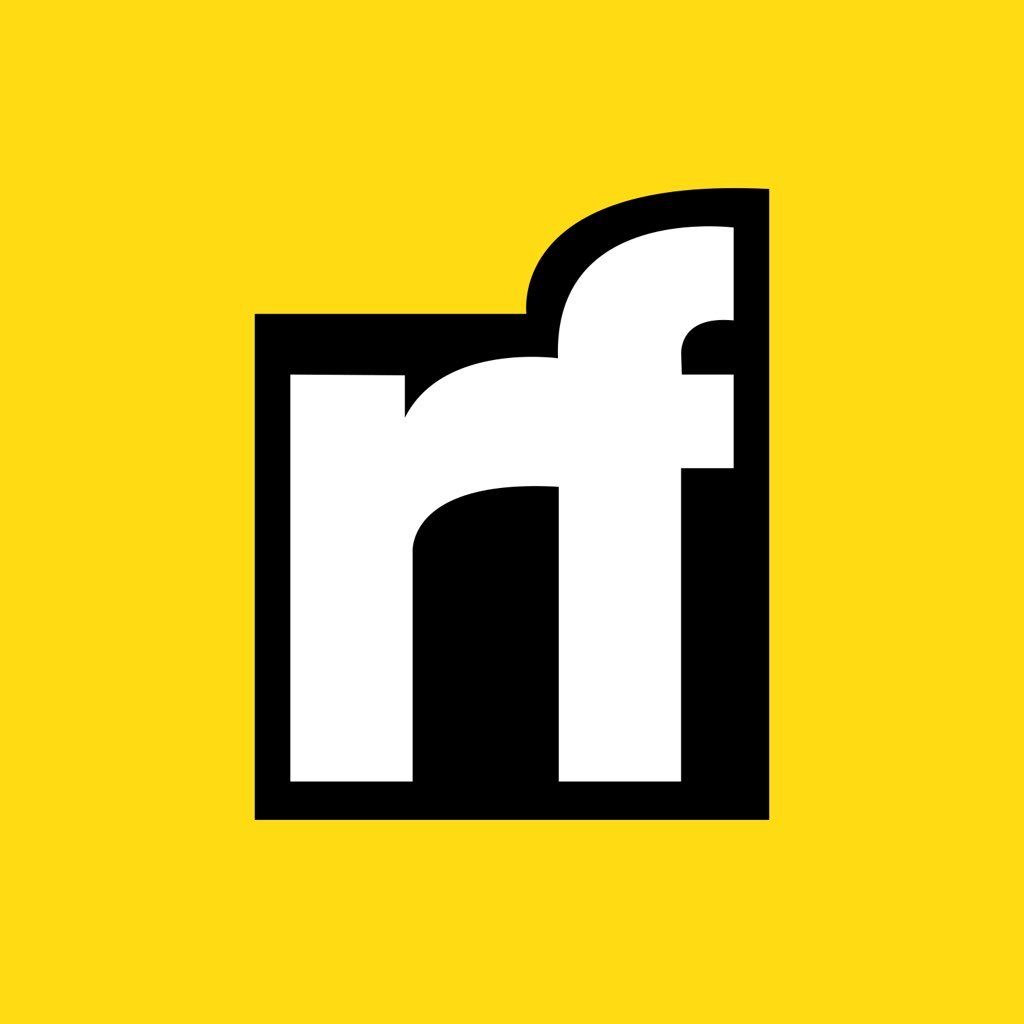
Mina | Sciencx (2022-02-09T11:47:28+00:00) A Simple Guide to fetch data using getStaticProps and getServerSideProps in Next.js. Retrieved from https://www.scien.cx/2022/02/09/a-simple-guide-to-fetch-data-using-getstaticprops-and-getserversideprops-in-next-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.