This content originally appeared on DEV Community and was authored by Aaron Wolf
Intro
This is going to be an ongoing "documentation" of cool little hacks that I've learned. Each entry will consist of what I did originally and what the better version is.
Hack 1: Using or
instead of conditional (React)
Context:
While iterating through a list of phone numbers, show <PhoneIcon />
next to only the first phone number.
Original code: Using a ternary operator
{phoneNumbers.map((phoneNumber, i) => (
<div>
{i === 0 ? <PhoneIcon /> : ''}
{phoneNumber}
</div>
)
Better: Using an or statement
contact.phones?.map((phoneNumber, i) => (
<div>
{!i && (<PhoneIcon />)}
{phoneNumber}
</div>
)
Explanation:
This works because the truthiness value of 0 is false. So while iterating through the phone numbers, if the index is 0 we can conditionally show the <PhoneIcon />
with !i && (<PhoneIcon />)
Hack 2: Joining an array
Context:
Sometimes there is an object with empty values, and I don't want this object to display if the values are empty. The problem is, empty values can be either null
, or empty strings ''
.
Example:
{ firstName: null,
lastName: null,
phone: '',
location: null,
favoriteColor: ''
}
Original code:
const emptyChecker = (obj) => {
const nulls = Object.values(obj).filter(val => val === null)
const empties = Object.values(obj).filter(val => val === '')
// checks length of nulls && empties against length of the whole
return nulls.concat(empties).length === Object.values(obj).length
}
Iterate through the values of the object and if they're null
add them to an array. Do it again, but with empty strings. Concat the 2 arrays and compare the length to the original.
Better:
const emptyChecker = (obj) => {
const empties = Object.values(obj).filter(val => !val)
// checks length of nulls && empties against length of the whole
return empties.length === Object.values(obj).length
}
Explanation:
This works because instead of iterating through the object values twice we convert the values to booleans as they're being iterated over. This works because null
has a truthiness value of false
as does an empty string ''
. Therefore .filter(val => !val)
only adds false values to the array and then we can compare the length to to the original.
This content originally appeared on DEV Community and was authored by Aaron Wolf
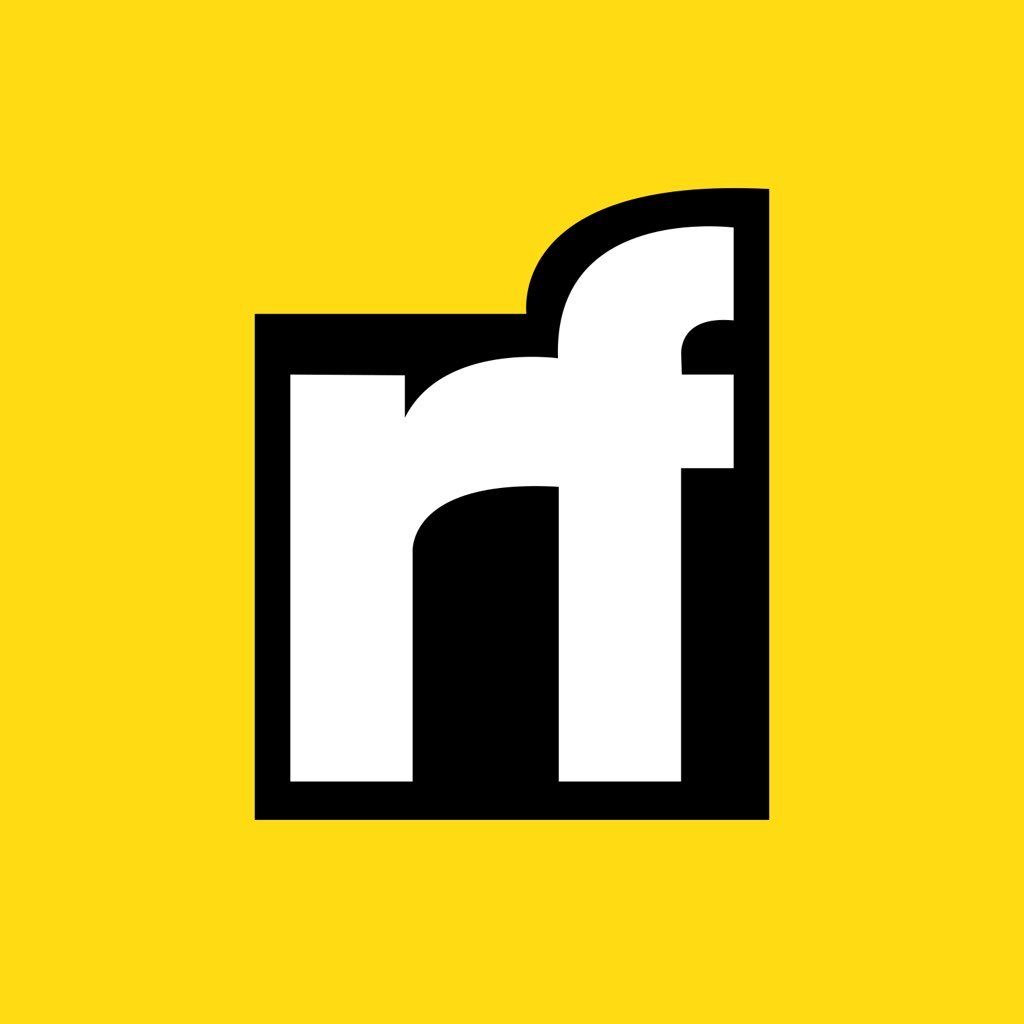
Aaron Wolf | Sciencx (2022-02-10T11:20:23+00:00) Some cool little hacks I’ve learned.. Retrieved from https://www.scien.cx/2022/02/10/some-cool-little-hacks-ive-learned/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.