This content originally appeared on DEV Community and was authored by Google Developer Student Clubs - NUML
In this article, we are going to learn the basic concepts of Object Oriented Programming also called the core of java. OOP is a method used for designing a program using classes and objects. Object Oriented Programming is a model that provide different type of concepts. OOP concepts in java is to improve code readability and reusability by defining a java program efficiently. An object-oriented programming is a way programming which enables programmers to think like they are working with real-life entities (a thing with distinct and independent existence) or objects. In real-life, people have knowledge and can do various works. In OOP, objects have fields to store knowledge/state/data and can do various works methods.
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Encapsulation:
First talking about encapsulation The main purpose of encapsulation is you would have full control on data by using the code.
In encapsulation, the variables of a class can be made hidden from other classes, and can be accessed only through the methods of their current class. Therefore, it is also known as data hiding.
Still didn’t understand?
Encapsulation can be described as a protective barrier that prevents the code and data being randomly accessed by other code defined outside the class. Access to the data and code is tightly controlled by a class.
Data Hiding/Data Encapsulation:
Encapsulation leads to data hiding. We use access specifiers to hide the data(properties) and methods. Use of private and public comes under this.
The user can only perform a restricted set of operations on the hidden members of the class by executing special functions commonly called methods.
Consider a real life example of encapsulation, like in a company there are different sections like the accounts section, finance section, sales section etc.
The finance section handles all the financial transactions and keep records of all the data related to finance. Similarly, the sales section handles all the sales related activities and keep records of all the sales. Now there may arise a situation when for some reason an official from finance section needs all the data about sales in a particular month. In this case, he is not allowed to directly access the data of sales section. He will first have to contact some other officer in the sales section and then request him to give the particular data. This is what encapsulation is. Here the data of sales section and the employees that can manipulate them are wrapped under a single name called “sales section”.
We use access modifiers (public and private keywords) for encapsulating data:
// java program on encapsulation
class EncapsulationExample
{
private int ID;
private String stuName;
private int stuAge;
// getter and setter methods
public int getStudentID()
{
return ID;
}
public String getStudentName()
{
return stuName;
}
public int getStudentAge()
{
return stuAge;
}
public void setStudentAge(int number)
{
stuAge = number;
}
public void setStudentName(String number)
{
stuName = number;
}
public void setStudentID(int number)
{
ID = number;
}
}
public class ExampleForEncapsulation
{
public static void main(String[] args)
{
EncapsulationExample student = new EncapsulationExample();
student.setStudentName("Virat");
student.setStudentAge(5);
student.setStudentID(2353);
System.out.println("Student Name: " + student.getStudentName());
System.out.println("Student ID: " + student.getStudentID());
System.out.println("Student Age: " + student.getStudentAge());
}
}
Inheritance:
Now coming towards inheritance, Inheritance is an integral part of Java OOPs which lets the properties of one class to be inherited by the other, as we know In OOP, computer programs are designed in such a way where everything is an object that interacts with one another It basically, helps in reusing the code and establish a relationship between different classes.
Like a child inherits the properties from his parents. A similar concept is followed in OOP Java , where we have two classes:
- Parent class ( Super or Base class )
- Child class ( Subclass or Derived class )
A class which inherits the properties is known as Child Class whereas a class whose properties are inherited is known as Parent class.
An extends keyword is used with child class indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality as it has excess to the objects of parent class.
Inheritance relationships often are shown graphically in a UML class diagram, with an arrow with an open arrowhead pointing to the parent class
Inheritance should create an is-a relationship, meaning the child is a more specific version of the parent.
Multiple classes can inherit from the same parent class, forming a tree-like hierarchy structure. Inheriting classes can add features beyond those inherited from the parent class to allow for unique behavior.
For example, an animal is the parent class having all breads of animals and the similarity between all animals is that they can eat and drink else different animals have different shapes and behavior but all animals can eat drink and pop in the same way so we write it as:
class animal {
void eat()
{
}
Void drink()
{
}
}
class Dog extends Animal
{
void bark()
{
}
}
class Cat extends Animal
{
void Meow()
{
}
}
Polymorphism:
The word polymorphism means having many forms. In simple words, we can define polymorphism as the ability of a message to be displayed in more than one form.
For example, a person at the same time can have different characteristic. Like a man at the same time is a father, a husband, an employee. So the same person possesses different behavior in different situations. This is called polymorphism.
Polymorphism is a feature of the object-oriented programming language, Java, which allows a single task to be performed in different ways (one name, multi shapes). In the technical world, polymorphism in java allows one to do multiple implementations by defining one interface.
There are two types of Polymorphism:
- Overloading ( Compile time polymorphism)
- OverRiding ( Run time polymorphism)
Overloading:
When there are multiple functions with same name but different parameters then these functions are said to be overloaded. Functions can be overloaded by change in number of parameters and change in type of parameter to change load.
Class Sum {
void Sum(int a, int b) {
System.out.println("The sum is:"+a);
}
void Sum(int a, int b, int c) {
System.out.println("");
}
}
PSVM ()
{
Sum obj =new Sum();
Obj.add(1, 10);
Obj.add(1,2,3);
}
Abstraction:
Abstraction is the concept of object-oriented programming that “shows” only essential attributes and “hides” unnecessary information. It’s the partial hiding of function or partial method hiding, the main purpose of abstraction is hiding the unnecessary details from the users. Abstraction is selecting data from a larger pool to show only relevant details of the object to the user. It helps in reducing programming complexity and efforts. It is also known as runtime Polymorphism.
Let’s take a daily life example to understand it completely, if a student wants to get admission in a particular university first he goes to check the prospectus of university that contain the important information about university, like which courses this university offers and how many departments are there, His concern is with the course title not with the outline of the courses. Same like he knows the departments not the exact location of departments and classes in the university means he has access to the limited information about university and prospectus omit unnecessary information same concept is use in Abstraction it shows only essential attributes and hide unnecessary information.
In the program below I’ve explain the concept by taking an example of mobile phone because we know more about phones as in this era all our work is done with the help of phone, so there are a lot of mobile phone companies but iPhone and Samsung are on the top and they have many amazing features, but call and messaging is the same in all the mobiles so we take them as an abstract method. Camera is also in both iPhone and Samsung but the features and properties are different and they are discussed in functions respectively.
// abstract class
abstract class S_Mobile phone
{
// abstract methods
Void call(){
}
Void Msg(){
}
abstract void camera();
}
class Sumsung extends S_MobliePhone
{
Void camera()
{
Return 13mp;
}
class iphone extends S_MobilePhone
{
Void camera()
{
Return 18mp;
}
}
Written by: Aiman Khalid
This content originally appeared on DEV Community and was authored by Google Developer Student Clubs - NUML
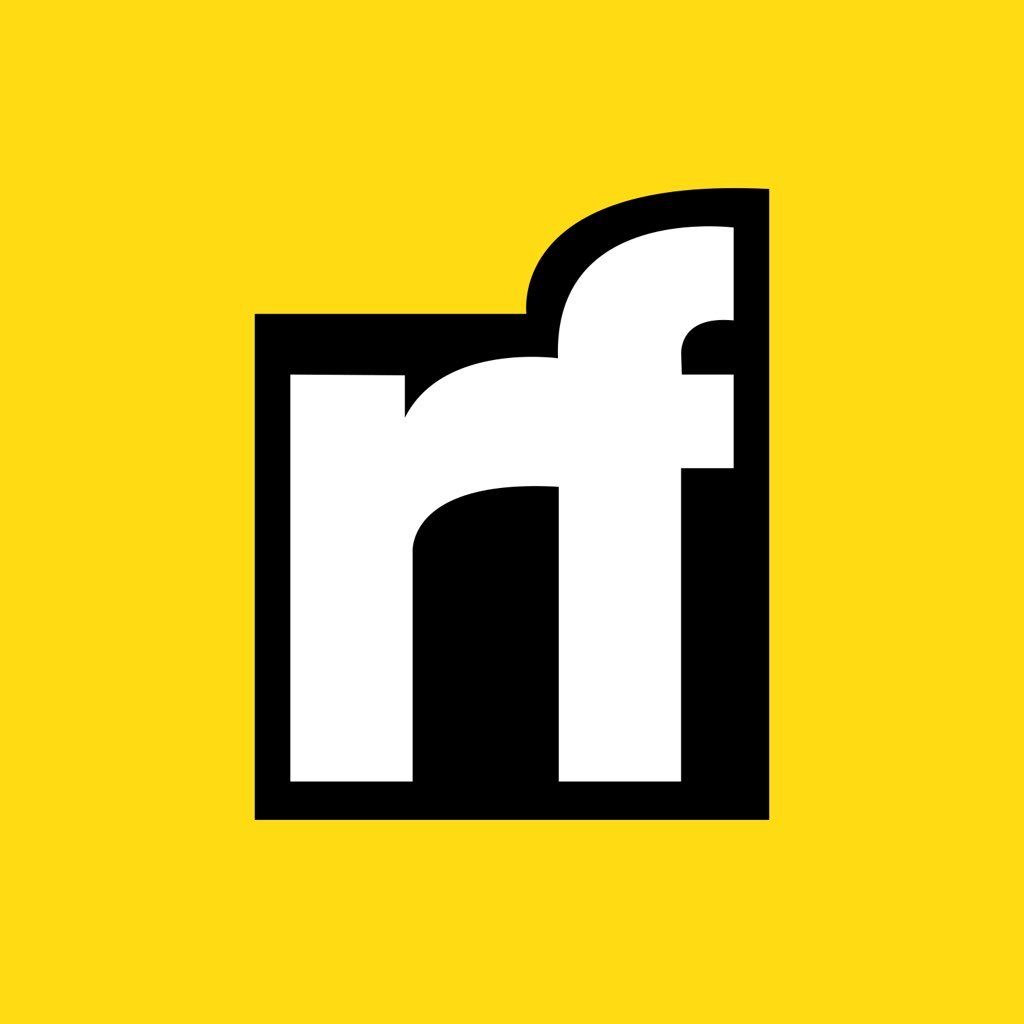
Google Developer Student Clubs - NUML | Sciencx (2022-02-12T12:28:51+00:00) Object Oriented Programming with java- an overview. Retrieved from https://www.scien.cx/2022/02/12/object-oriented-programming-with-java-an-overview/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.