This content originally appeared on DEV Community and was authored by dunnyk
Like many other programming languages, Python also has it’s sweet history, Python was conceived in the late 1980s by Guido van Rossum at Centrum Wiskunde & Informatica (CWI) in the Netherlands as a successor to the ABC programming language, which was inspired by SETL, capable of exception handling and interfacing with the Amoeba operating system. Its implementation began in December 1989. Python is a widely used general-purpose, high-level programming language.It was mainly developed for emphasis on code readability, and its syntax allows programmers to express concepts in fewer lines of code.
Variables and Literals
Python has no command for declaring a variable.
A variable is created the moment you first assign a value to it.Variables do not need to be declared with any particular type, and can also change type after they have been set.
Example
a = 5
print("a =", 5)
a = "High five"
print("a =", a)
A variable is a named location used to store data in the memory.Refer to the above case.
Data type that a function return can be specified as shown
def hello() ->int:
return 6
NB Declaration of datatype is not mostly done
Operators
Operators are special symbols that carry out operations on operands (variables and values).Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication etc.
x = 14
y = 4
# Add two operands
print('x + y =', x+y) # Output: x + y = 18
# Subtract right operand from the left
print('x - y =', x-y) # Output: x - y = 10
# Multiply two operands
print('x * y =', x*y) # Output: x * y = 56
# Divide left operand by the right one
print('x / y =', x/y) # Output: x / y = 3.5
Assignment operators are used to assign values to variables. You have already seen the use of = operator. Let's try some more assignment operators.
x = 5
# x += 5 ----> x = x + 5
x +=5
print(x) # Output: 10
# x /= 5 ----> x = x / 5
x /= 5
print(x) # Output: 2.0
Other commonly used assignment operators: -=, =, %=, //= and *=.
Get Input from User
In python, you can take input from the user, this can be achieved using an input function.
inputString = input('Enter a sentence:')
print('The inputted string is:', inputString)
run the program and the output will:
Enter a sentence: Good Morning Lux Developers...
The inputted string is: Good Morning Lux Developers...
Python Comments
Python has three ways of writing comments.
For more about doc string please visit this Python Comments
# This is a comment
"""This is a
multiline
comment."""
'''This is a
multiline
comment.'''
Type Conversion
The process of converting the value of one data type (integer, string, float, etc.) to another is called type conversion/type casting. Python has two types of type conversion.
num_int = 13 # integer type
num_flo = 1.53 # float type
num_new = num_int + num_flo
print("Value of num_new:",num_new)
print("datatype of num_new:",type(num_new))
let's print this.
Value of num_new: 124.23
datatype of num_new: datatype of num_new: <class 'float'>
Here, num_new has float data type because Python always converts smaller data type to larger data type to avoid the loss of data.
Explicit Conversion
For explicit casting/conversion of datatype to required datatype, we use explicit functions like int(), float(), str().
Below is an implementation of explicit conversion.
num_int = 12 # int type
num_str = "10" # str type
# explicitly converted to int type
num_str = int(num_str)
print(num_int+num_str)# This outputs 22
but an error is expected if no direct casting of str to int
an error 'can't add a str to an int is expected'
Here is more on type conversion.
Python Data Structures
You will learn about those built-in types in this section.
Lists
A list is denoted using a square bracket and elements inside are separated using a comma. Items inside a list can be of different types(int, floats, boolean, str etc.
# empty list
my_list = []
# list of integers
my_list = [1, 2, 3]
# list with mixed data types
my_list = [1, "Hello", 3.4]
Here to access items in a list.
language = ["French", "German", "English", "Polish"]
# Accessing first element
print(language[0])
# Accessing fourth element
print(language[3])```
# Accessing first element
print(language[0])
# Accessing fourth element
print(language[3])
You use the index operator [] to access an item in a list. Index starts from 0. So, a list having 10 elements will have index from 0 to 9.
Python also allows negative indexing for its sequences. The index of -1 refers to the last item, -2 to the second last item and so on. For more information about lists check here:-
Python lists, Python list methods, Python list comprehension
Tuples
Tuple is similar to lists only that it's immutable once defined and use parenthesis() brackets. Remember for lists you can change the items after declaring them.
language = ("French", "German", "English", "Polish")
print(language)
You can also use tuple() function to create tuples.
You can access elements of a tuple in a similar way like a list.
language = ("French", "German", "English", "Polish")
print(language[1]) #Output: German
print(language[3]) #Output: Polish
print(language[-1]) # Output: Polish
You cannot delete elements of a tuple, however, you can entirely delete a tuple itself using del operator
String
String can be one character or a whore word.See below code snippet
# all of the following are equivalent
my_string = 'Hello'
print(my_string)
my_string = "Hello"
print(my_string)
my_string = '''Hello'''
print(my_string)
# triple quotes string can extend multiple lines
my_string = """Hello, welcome to
the world of Python"""
print(my_string)
You can access characters of a string using indexing and slicing.
str = 'luxAcademy'
print('str = ', str)
print('str[0] = ', str[0]) # Output: l
print('str[-1] = ', str[-1]) # Output: y
#slicing 2nd to 5th character
print('str[1:5] = ', str[1:5]) # Output: uxAc
#slicing 6th to 2nd last character
print('str[5:-2] = ', str[5:-2]) # Output: ade
Strings are immutable once declared. However, you can assign one string to another and also you can delete the string using del operator.
Concatenation is the most common string operation. Se below.
str1 = 'Hello '
str2 ='World!'
# Output: Hello World!
print(str1 + str2)
# Hello Hello Hello
print(str1 * 3)
For more about Stings check Here
Sets
A set is unordered collection of items where each item is unique(no duplicates)
# set of integers
my_set = {1, 2, 3}
print(my_set)
# set of mixed datatypes
my_set = {1.0, "Hello", (1, 2, 3)}
print(my_set)
you can learn more about set function here.
Sets are mutable. You can add, remove and delete elements of a set. However, you cannot replace one item of a set with another as they are unordered and indexing have no meaning.
Common methods on sets are add(), [update(), and remove()
# set of integers
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
my_set.add(2)
print(my_set) # Output: {1, 2, 3, 4}
my_set.update([3, 4, 5])
print(my_set) # Output: {1, 2, 3, 4, 5}
my_set.remove(4)
print(my_set) # Output: {1, 2, 3, 5}
Dictionaries
This is unordered collection of items.While other compound data have only items and you can access items using index. Dictionaries use key : value pair. Check below
# empty dictionary
my_dict = {}
# dictionary with integer keys
my_dict = {1: 'apple', 2: 'ball'}
# dictionary with mixed keys
my_dict = {'name': 'John', 1: [2, 4, 3]}
You can use dict() function to create dictionaries, as indicated above, to access values from a dictionary use dictionary[keys].
for example:-
person = {'name':'Jack', 'age': 26, 'salary': 4534.2}
print(person['age']) # Output: 26
To add or remove items from a dictionary, use this procedure below.
person = {'name':'Jack', 'age': 26}
# Changing age to 36
person['age'] = 36
print(person) # Output: {'name': 'Jack', 'age': 36}
# Adding salary key, value pair
person['salary'] = 4342.4
print(person) # Output: {'name': 'Jack', 'age': 36, 'salary': 4342.4}
# Deleting age
del person['age']
print(person) # Output: {'name': 'Jack', 'salary': 4342.4}
# Deleting entire dictionary
del person
Python Control Flow
if...else Statement
Like in real life scenarios, We do things depending with conditions, for example if lunch time, and feeling hungry, ...prepare lunch. Two conditions here must be met, i.e one must be hungry and is lunch time.
This is the same thing with if.... else in python. They control flows. The if...else statement is used if you want perform different action (run different code) on different condition.
For example:
num = -1
if num > 0:
print("Positive number")
elif num == 0:
print("Zero")
else:
print("Negative number")
# Output: Negative number
There can be zero or more elif parts, and the else part is optional.
Most programming languages use {} to specify the block of code. Python uses indentation.
A code block starts with indentation and ends with the first unindented line. The amount of indentation is up to you, but it must be consistent throughout that block.
Generally, four whitespace is used for indentation and is preferred over tabs.
Let's try another example:
if False:
print("I am inside the body of if.")
print("I am also inside the body of if.")
print("I am outside the body of if")
# Output: I am outside the body of if.
Before we proceed, Check on the comparison operator and logical operator, for more details about python if...else in details.
while Loop
Most programming stacks use while loop to iterate over a block of code as long as the condition is true. See the implementation below.
n = 50
initialize sum and counter
sum = 0
counter = 1
while counter <= n:
sum += counter#sum = sum + counter
counter += counter
print(sum)
# Output: The sum is 1275
for Loop
In Python, For loop is commonly used than while loop, iterating over sequence is called traversal, this can happen over(list, tuple, string)
Here's an example to find the sum of all numbers stored in a list.
numbers = [6, 5, 3, 8, 4, 2]
sum = 0
# iterate over the list
for val in numbers:
sum = sum+val
print("The sum is", sum) # Output: The sum is 28
Notice the use of in operator in the above example. The in operator returns True if value/variable is found in the sequence.
In Python, for loop can have optional else block. The else part is executed if the items in the sequence used in for loop exhausts. However, if the loop is terminated with break statement, Python interpreter ignores the else block.
break Statement
The break statement terminates the loop containing it. Control of the program flows to the statement immediately after the body of the loop.
for val in "string":
if val == "r":
break
print(val)
print("The end")
continue Statement
The continue statement is used to skip the rest of the code inside a loop for the current iteration only. Loop does not terminate but continues on with the next iteration. For example:
for val in "string":
if val == "r":
continue
print(val)
print("The end")
#here the output will be s t i n g, and finally The end
pass Statement
Suppose, you have a loop or a function that is not implemented yet, but want to implement it in the future. They cannot have an empty body. The interpreter would complain. So, you use the pass statement to construct a body that does nothing.
sequence = {'p', 'a', 's', 's'}
for val in sequence:
pass
Python Function
A function is a group of related statements that perform a specific task. You use def keyword to create functions in Python.
def print_lines():
print("I am line1 in Lux Academy.")
print("I am line2 in Lux Academy.")
# function call
print_lines()
Functions also accepts parameters. When it's the case, put the variables in the brackets after function name.See below.
def add_numbers(a, b):
sum = a + b
return sum
result = add_numbers(4, 5)
print(result)
# Output: 9
NOTE
To shine and excel in programming,practice is important, sitting down for long hours figuring out a problem and how best to solve it is paramount.
Ensure to always stay put until you achieve your goal, no one is whipped until they quit.
Enough of that, now lets dirt our fingers with code and challenge our minds.
This content originally appeared on DEV Community and was authored by dunnyk
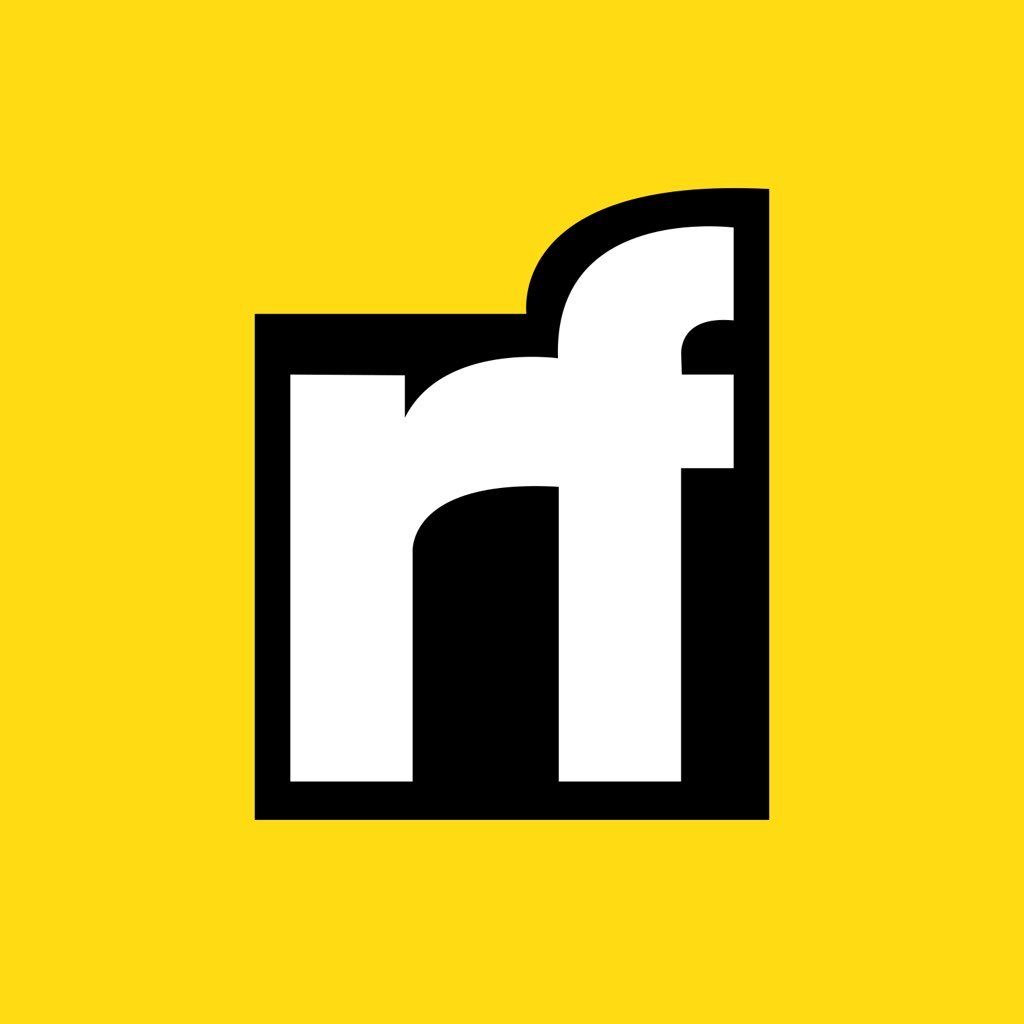
dunnyk | Sciencx (2022-02-14T14:09:37+00:00) Python 101: Ultimate Python guide.. Retrieved from https://www.scien.cx/2022/02/14/python-101-ultimate-python-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.