This content originally appeared on DEV Community and was authored by Michael Burrows
In this tutorial we’ll create 3 different types percentage calculators using JavaScript.
- X is what % of Y?
- What is X% of Y?
- What is the % increase or decrease between X & Y?
Each calculator has almost identical HTML markup and the only difference in the JavaScript is the maths used to calculate the solution. If you just looking for a copy and paste solution a working HTML file with the 3 calculators can be downloaded from here.
1 – X is what % of Y?
Example – 50 is 20% of 250 || (50 / 250) * 100 = 20%
<form id="calc1">
<input id="calc1-num-x" type="number" /> is what percent of
<input id="calc1-num-y" type="number" /> ?
<input id="calc1-submit" type="submit" value="Calculate" />
<input id="calc1-solution" type="number" readonly="readonly" />
</form>
document.getElementById("calc1-submit")
.addEventListener("click", function (e) {
e.preventDefault();
const numX = document.getElementById("calc1-num-x").value;
const numY = document.getElementById("calc1-num-y").value;
const percentage = (numX / numY) * 100;
document.getElementById("calc1-solution").value = percentage;
});
2 – What is X% of Y?
Example – 50% of 250 = 125 || (50 / 100) * 250 = 125
<form id="calc2">
What is <input id="calc2-num-x" type="number" /> % of
<input id="calc2-num-y" type="number" /> ?
<input id="calc2-submit" type="submit" value="Calculate" />
<input id="calc2-solution" type="number" readonly="readonly" />
</form>
document.getElementById("calc2-submit")
.addEventListener("click", function (e) {
e.preventDefault();
const numX = document.getElementById("calc2-num-x").value;
const numY = document.getElementById("calc2-num-y").value;
const percentage = (numX / 100) * numY;
document.getElementById("calc2-solution").value = percentage;
});
3 – What is the % increase or decrease between X & Y?
Example – 50 to 75 = 50% || (75 / 50) / 50 * 100 = 50%
<form id="calc3">
What is the percentage increase or decrease from
<input id="calc3-num-x" type="number" /> to
<input id="calc3-num-y" type="number" /> ?
<input id="calc3-submit" type="submit" value="Calculate" />
<input id="calc3-solution" type="number" readonly="readonly" />
</form>
document.getElementById("calc3-submit")
.addEventListener("click", function (e) {
e.preventDefault();
const numX = document.getElementById("calc3-num-x").value;
const numY = document.getElementById("calc3-num-y").value;
const percentage = (numY - numX) / numX * 100;
document.getElementById("calc3-solution").value = percentage;
});
For each of the calculators we’ve added a click
event listener to the submit buttons. When these buttons are clicked the 2 numbers entered into the form are saved as variables. We then perform the percentage calculation on these numbers and output the value into the solution input field.
This content originally appeared on DEV Community and was authored by Michael Burrows
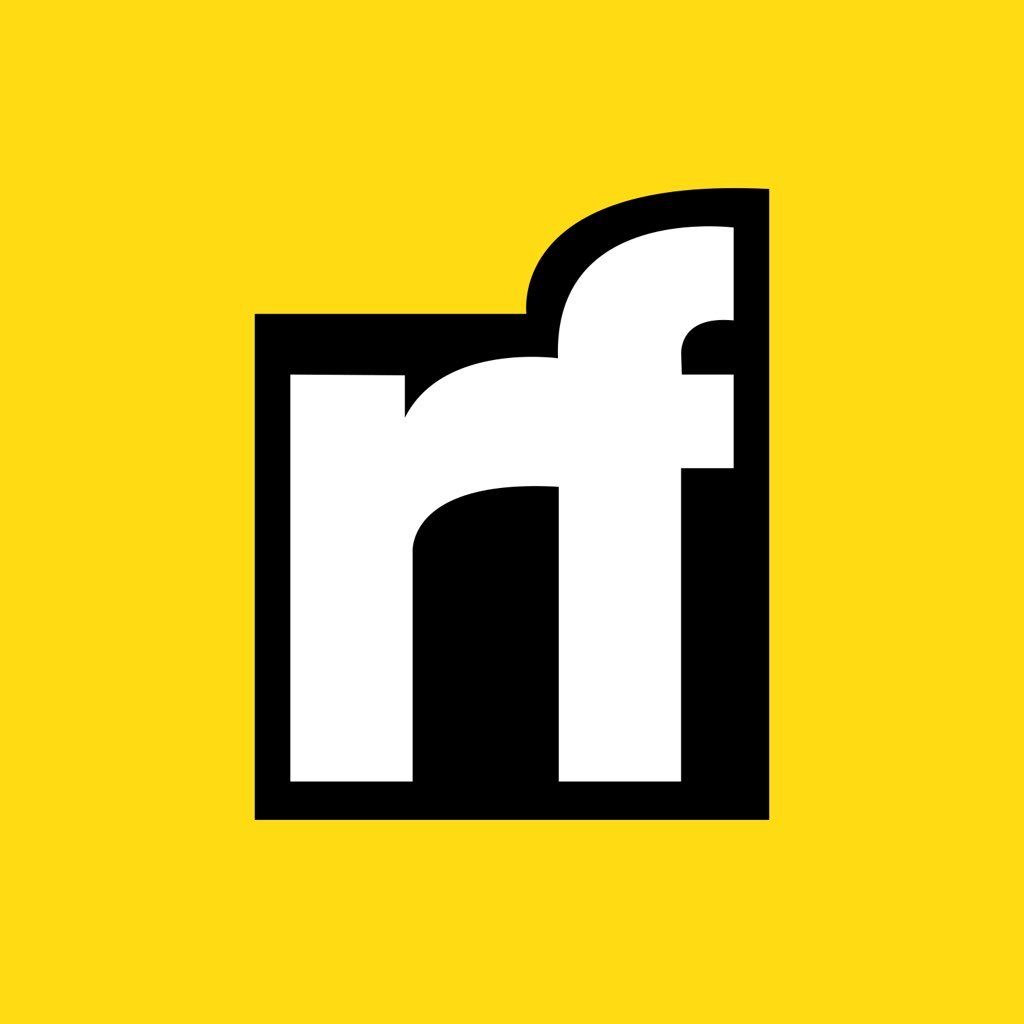
Michael Burrows | Sciencx (2022-02-18T00:55:39+00:00) How to create a percentage calculator using JavaScript. Retrieved from https://www.scien.cx/2022/02/18/how-to-create-a-percentage-calculator-using-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.